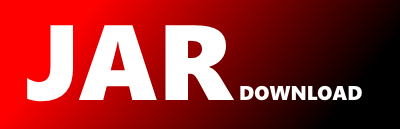
org.efaps.ui.wicket.components.values.BooleanField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of efaps-webapp Show documentation
Show all versions of efaps-webapp Show documentation
eFaps WebApp provides a web interface as the User Interface for eFaps
which can be easily expanded and altered.
/*
* Copyright 2003 - 2012 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8453 $
* Last Changed: $Date: 2012-12-27 16:37:27 -0500 (Thu, 27 Dec 2012) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.ui.wicket.components.values;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.wicket.markup.html.basic.Label;
import org.apache.wicket.markup.html.form.Radio;
import org.apache.wicket.markup.html.form.RadioGroup;
import org.apache.wicket.markup.html.panel.Panel;
import org.apache.wicket.model.IModel;
import org.apache.wicket.model.Model;
import org.efaps.ui.wicket.components.FormContainer;
import org.efaps.ui.wicket.models.cell.FieldConfiguration;
import org.efaps.ui.wicket.request.EFapsRequestParametersAdapter;
import org.efaps.util.EFapsException;
/**
* TODO comment!
*
* @author The eFaps Team
* @version $Id: BooleanField.java 8453 2012-12-27 21:37:27Z [email protected] $
*/
public class BooleanField
extends Panel
implements IValueConverter
{
/**
* Needed for serialization.
*/
private static final long serialVersionUID = 1L;
/**
* Configuration for this field.
*/
private final FieldConfiguration fieldConfiguration;
/**
* @param _wicketId wicket id for this component
* @param _value value of this component
* @param _choices choices
* @param _fieldConfiguration configuration for this field
*/
public BooleanField(final String _wicketId,
final Object _value,
final IModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy