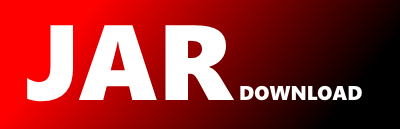
org.efaps.ui.wicket.models.objects.UITable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of efaps-webapp Show documentation
Show all versions of efaps-webapp Show documentation
eFaps WebApp provides a web interface as the User Interface for eFaps
which can be easily expanded and altered.
/*
* Copyright 2003 - 2012 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 9036 $
* Last Changed: $Date: 2013-03-12 19:54:27 -0500 (Tue, 12 Mar 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.ui.wicket.models.objects;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.UUID;
import org.apache.wicket.RestartResponseException;
import org.apache.wicket.util.io.IClusterable;
import org.efaps.admin.datamodel.Attribute;
import org.efaps.admin.datamodel.Type;
import org.efaps.admin.datamodel.ui.FieldValue;
import org.efaps.admin.event.EventDefinition;
import org.efaps.admin.event.EventType;
import org.efaps.admin.event.Parameter.ParameterValues;
import org.efaps.admin.event.Return;
import org.efaps.admin.event.Return.ReturnValues;
import org.efaps.admin.ui.AbstractCommand;
import org.efaps.admin.ui.AbstractCommand.SortDirection;
import org.efaps.admin.ui.Image;
import org.efaps.admin.ui.field.Field;
import org.efaps.admin.ui.field.Filter;
import org.efaps.db.Context;
import org.efaps.db.Instance;
import org.efaps.db.MultiPrintQuery;
import org.efaps.ui.wicket.models.cell.UIHiddenCell;
import org.efaps.ui.wicket.models.cell.UITableCell;
import org.efaps.ui.wicket.models.objects.UITableHeader.FilterType;
import org.efaps.ui.wicket.pages.error.ErrorPage;
import org.efaps.util.DateTimeUtil;
import org.efaps.util.EFapsException;
import org.efaps.util.cache.CacheReloadException;
import org.joda.time.DateMidnight;
import org.joda.time.DateTime;
import org.joda.time.Interval;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* TODO description!
*
* @author The eFaps Team
* @version $Id: UITable.java 9036 2013-03-13 00:54:27Z [email protected] $
*/
public class UITable
extends AbstractUIHeaderObject
{
/**
* Logging instance used in this class.
*/
private static final Logger LOG = LoggerFactory.getLogger(UITable.class);
/**
* Serial Id.
*/
private static final long serialVersionUID = 1L;
/**
* Map contains the applied filters to this table.
*/
private final Map filters = new HashMap();
/**
* Map is used to store the filters in relation to a field name. It is used
* temporarily when the table is (due to a database based filter) requeried,
* to be able to set the filters to the headers by copying it from the old
* header to the new one.
*
* @see #getInstanceListsOld()
* @see #execute4InstanceOld()
*/
private final Map filterTempCache = new HashMap();
/**
* All evaluated rows of this table are stored in this list.
*
* @see #getValues
*/
private final List values = new ArrayList();
/**
* Thie Row is used in case of edit to create new empty rows.
*/
private UIRow emptyRow;
/**
* Constructor setting the uuid and Key of the instance.
*
* @param _commandUUID UUID of the Command
* @param _instanceKey Key of the instance
* @throws EFapsException on error
*/
public UITable(final UUID _commandUUID,
final String _instanceKey)
throws EFapsException
{
super(_commandUUID, _instanceKey);
initialise();
}
/**
* Constructor setting the uuid and Key of the instance.
*
* @param _commandUUID UUID of the Command
* @param _instanceKey Key of the instance
* @param _openerId id of the opener
* @throws EFapsException on error
*/
public UITable(final UUID _commandUUID,
final String _instanceKey,
final String _openerId)
throws EFapsException
{
super(_commandUUID, _instanceKey, _openerId);
initialise();
}
/**
* Method that initializes the TableModel.
*
* @throws EFapsException on error
*/
private void initialise()
throws EFapsException
{
final AbstractCommand command = getCommand();
if (command == null) {
setShowCheckBoxes(false);
} else {
// set target table
if (command.getTargetTable() != null) {
setTableUUID(command.getTargetTable().getUUID());
if (Context.getThreadContext().containsSessionAttribute(getCacheKey(UITable.UserCacheKey.FILTER))) {
@SuppressWarnings("unchecked")
final Map sessfilter = (Map) Context.getThreadContext()
.getSessionAttribute(getCacheKey(UITable.UserCacheKey.FILTER));
for (final Field field : command.getTargetTable().getFields()) {
if (sessfilter.containsKey(field.getName())) {
final TableFilter filter = sessfilter.get(field.getName());
filter.setHeaderFieldId(field.getId());
this.filters.put(field.getName(), filter);
}
}
} else {
// add the filter here, if it is a required filter that must be
// applied against the database
for (final Field field : command.getTargetTable().getFields()) {
if (field.getFilter().isRequired()
&& field.getFilter().getBase().equals(Filter.Base.DATABASE)) {
this.filters.put(field.getName(), new TableFilter());
}
}
}
}
// set default sort
if (command.getTargetTableSortKey() != null) {
setSortKeyInternal(command.getTargetTableSortKey());
setSortDirection(command.getTargetTableSortDirection());
}
setShowCheckBoxes(command.isTargetShowCheckBoxes());
// get the User specific Attributes if exist overwrite the defaults
try {
if (Context.getThreadContext().containsUserAttribute(
getCacheKey(UITable.UserCacheKey.SORTKEY))) {
setSortKeyInternal(Context.getThreadContext().getUserAttribute(
getCacheKey(UITable.UserCacheKey.SORTKEY)));
}
if (Context.getThreadContext().containsUserAttribute(
getCacheKey(UITable.UserCacheKey.SORTDIRECTION))) {
setSortDirection(SortDirection.getEnum(Context.getThreadContext()
.getUserAttribute(getCacheKey(UITable.UserCacheKey.SORTDIRECTION))));
}
} catch (final EFapsException e) {
// we don't throw an error because this are only Usersettings
UITable.LOG.error("error during the retrieve of UserAttributes", e);
}
}
}
/**
* Method to get the list of instance.
*
* @return List of instances
* @throws EFapsException on error
*/
@SuppressWarnings("unchecked")
protected List getInstanceList()
throws EFapsException
{
// get the filters that must be applied against the database
final Map> dataBasefilters = new HashMap>();
final Iterator> iter = this.filters.entrySet().iterator();
this.filterTempCache.clear();
while (iter.hasNext()) {
final Entry entry = iter.next();
if (entry.getValue().getUiTableHeader() == null
|| (entry.getValue().getUiTableHeader() != null
&& entry.getValue().getUiTableHeader().getFilter().getBase().equals(Filter.Base.DATABASE))) {
final Map map = entry.getValue().getMap4esjp();
dataBasefilters.put(entry.getKey(), map);
}
this.filterTempCache.put(entry.getKey(), entry.getValue());
iter.remove();
}
final List ret = getCommand().executeEvents(EventType.UI_TABLE_EVALUATE,
ParameterValues.INSTANCE, getInstance(),
ParameterValues.OTHERS, dataBasefilters);
List lists = null;
if (ret.size() < 1) {
throw new EFapsException(UITable.class, "getInstanceList");
} else {
lists = (List) ret.get(0).get(ReturnValues.VALUES);
}
return lists;
}
/**
* {@inheritDoc}
*/
@Override
public void execute()
{
try {
if (isCreateMode()) {
execute4NoInstance();
} else {
final List instances = getInstanceList();
if (instances.isEmpty() && isEditMode()) {
execute4NoInstance();
} else {
execute4Instance(instances);
}
}
} catch (final EFapsException e) {
throw new RestartResponseException(new ErrorPage(e));
}
super.setInitialized(true);
}
/**
* @param _instances list of instances the table is executed for
* @throws EFapsException on error
*/
private void execute4Instance(final List _instances)
throws EFapsException
{
final SetaltOIDSel = new HashSet();
// evaluate for all expressions in the table
final MultiPrintQuery multi = new MultiPrintQuery(_instances);
final List userWidthList = getUserWidths();
final List fields = getUserSortedColumns();
int i = 0;
Type type;
if (_instances.size() > 0) {
type = _instances.get(0).getType();
} else {
type = getTypeFromEvent();
}
for (final Field field : fields) {
if (field.hasAccess(getMode(), getInstance(), getCommand()) && !field.isNoneDisplay(getMode())) {
Attribute attr = null;
if (_instances.size() > 0) {
if (field.getSelect() != null) {
multi.addSelect(field.getSelect());
} else if (field.getAttribute() != null) {
multi.addAttribute(field.getAttribute());
} else if (field.getPhrase() != null) {
multi.addPhrase(field.getName(), field.getPhrase());
}
if (field.getSelectAlternateOID() != null) {
multi.addSelect(field.getSelectAlternateOID());
altOIDSel.add(field.getSelectAlternateOID());
}
}
if (field.getAttribute() != null && type != null) {
attr = type.getAttribute(field.getAttribute());
}
SortDirection sortdirection = SortDirection.NONE;
if (field.getName().equals(getSortKey())) {
sortdirection = getSortDirection();
}
if (field.getFilter().getAttributes() != null) {
if (field.getFilter().getAttributes().contains(",")) {
if (field.getFilter().getAttributes().contains("/")) {
attr = Attribute.get(field.getFilter().getAttributes().split(",")[0]);
} else {
attr = type.getAttribute(field.getFilter().getAttributes().split(",")[0]);
}
} else {
if (field.getFilter().getAttributes().contains("/")) {
attr = Attribute.get(field.getFilter().getAttributes());
} else {
attr = type.getAttribute(field.getFilter().getAttributes());
}
}
}
if (!field.isHiddenDisplay(getMode())) {
final UITableHeader uiTableHeader = new UITableHeader(field, sortdirection, attr);
if (this.filterTempCache.containsKey(uiTableHeader.getFieldName())) {
this.filters.put(uiTableHeader.getFieldName(),
this.filterTempCache.get(uiTableHeader.getFieldName()));
uiTableHeader.setFilterApplied(true);
} else if (uiTableHeader.getFilter().isRequired()) {
this.filters.put(uiTableHeader.getFieldName(), new TableFilter(uiTableHeader));
}
getHeaders().add(uiTableHeader);
if (!field.isFixedWidth()) {
if (userWidthList != null && userWidthList.size() > i) {
if (isShowCheckBoxes() && userWidthList.size() > i + 1) {
uiTableHeader.setWidth(userWidthList.get(i + 1));
} else {
uiTableHeader.setWidth(userWidthList.get(i));
}
}
setWidthWeight(getWidthWeight() + field.getWidth());
}
}
i++;
}
}
multi.execute();
if (!altOIDSel.isEmpty()) {
final List inst = new ArrayList();
for (final String sel : altOIDSel) {
inst.addAll(multi.getInstances4Select(sel));
}
checkAccessToInstances(inst);
}
executeRowResult(multi, fields);
if (getSortKey() != null) {
sort();
}
}
/**
* @param _multi Query
* @param _fields Fields
* @throws EFapsException on error
*/
private void executeRowResult(final MultiPrintQuery _multi,
final List _fields)
throws EFapsException
{
boolean first = true;
while (_multi.next()) {
Instance instance = _multi.getCurrentInstance();
final UIRow row = new UIRow(this, instance.getKey());
String strValue = "";
if (isEditMode() && first) {
this.emptyRow = new UIRow(this);
}
for (final Field field : _fields) {
if (field.getSelectAlternateOID() != null) {
instance = Instance.get(_multi. getSelect(field.getSelectAlternateOID()));
} else {
instance = _multi.getCurrentInstance();
}
if (field.hasAccess(getMode(), instance, getCommand()) && !field.isNoneDisplay(getMode())) {
Object value = null;
Attribute attr = null;
if (field.getSelect() != null) {
value = _multi.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy