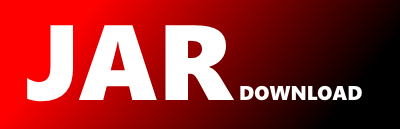
org.ehcache.jsr107.Eh107CacheStatisticsMXBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ehcache-107 Show documentation
Show all versions of ehcache-107 Show documentation
The JSR-107 compatibility module of Ehcache 3
/*
* Copyright Terracotta, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ehcache.jsr107;
import org.ehcache.Cache;
import org.ehcache.core.InternalCache;
import org.ehcache.core.statistics.CacheOperationOutcomes;
import org.ehcache.core.statistics.StoreOperationOutcomes;
import org.ehcache.core.statistics.BulkOps;
import org.terracotta.context.ContextManager;
import org.terracotta.context.TreeNode;
import org.terracotta.context.query.Matcher;
import org.terracotta.context.query.Matchers;
import org.terracotta.context.query.Query;
import org.terracotta.statistics.OperationStatistic;
import org.terracotta.statistics.StatisticsManager;
import org.terracotta.statistics.derived.LatencySampling;
import org.terracotta.statistics.derived.MinMaxAverage;
import org.terracotta.statistics.jsr166e.LongAdder;
import org.terracotta.statistics.observer.ChainedOperationObserver;
import java.util.Collections;
import java.util.EnumSet;
import java.util.Map;
import java.util.Set;
import static java.util.EnumSet.allOf;
import static org.terracotta.context.query.Matchers.attributes;
import static org.terracotta.context.query.Matchers.context;
import static org.terracotta.context.query.Matchers.hasAttribute;
import static org.terracotta.context.query.QueryBuilder.queryBuilder;
/**
* @author Ludovic Orban
*/
class Eh107CacheStatisticsMXBean extends Eh107MXBean implements javax.cache.management.CacheStatisticsMXBean {
private final CompensatingCounters compensatingCounters = new CompensatingCounters();
private final OperationStatistic get;
private final OperationStatistic put;
private final OperationStatistic remove;
private final OperationStatistic putIfAbsent;
private final OperationStatistic replace;
private final OperationStatistic conditionalRemove;
private final OperationStatistic authorityEviction;
private final Map bulkMethodEntries;
private final LatencyMonitor averageGetTime;
private final LatencyMonitor averagePutTime;
private final LatencyMonitor averageRemoveTime;
Eh107CacheStatisticsMXBean(String cacheName, Eh107CacheManager cacheManager, InternalCache cache) {
super(cacheName, cacheManager, "CacheStatistics");
this.bulkMethodEntries = cache.getBulkMethodEntries();
get = findCacheStatistic(cache, CacheOperationOutcomes.GetOutcome.class, "get");
put = findCacheStatistic(cache, CacheOperationOutcomes.PutOutcome.class, "put");
remove = findCacheStatistic(cache, CacheOperationOutcomes.RemoveOutcome.class, "remove");
putIfAbsent = findCacheStatistic(cache, CacheOperationOutcomes.PutIfAbsentOutcome.class, "putIfAbsent");
replace = findCacheStatistic(cache, CacheOperationOutcomes.ReplaceOutcome.class, "replace");
conditionalRemove = findCacheStatistic(cache, CacheOperationOutcomes.ConditionalRemoveOutcome.class, "conditionalRemove");
authorityEviction = findAuthoritativeTierStatistic(cache, StoreOperationOutcomes.EvictionOutcome.class, "eviction");
averageGetTime = new LatencyMonitor(allOf(CacheOperationOutcomes.GetOutcome.class));
get.addDerivedStatistic(averageGetTime);
averagePutTime = new LatencyMonitor(allOf(CacheOperationOutcomes.PutOutcome.class));
put.addDerivedStatistic(averagePutTime);
averageRemoveTime= new LatencyMonitor(allOf(CacheOperationOutcomes.RemoveOutcome.class));
remove.addDerivedStatistic(averageRemoveTime);
}
@Override
public void clear() {
compensatingCounters.snapshot();
averageGetTime.clear();
averagePutTime.clear();
averageRemoveTime.clear();
}
@Override
public long getCacheHits() {
return normalize(getHits() - compensatingCounters.cacheHits - compensatingCounters.bulkGetHits);
}
@Override
public float getCacheHitPercentage() {
long cacheHits = getCacheHits();
return normalize((float) cacheHits / (cacheHits + getCacheMisses())) * 100.0f;
}
@Override
public long getCacheMisses() {
return normalize(getMisses() - compensatingCounters.cacheMisses - compensatingCounters.bulkGetMiss);
}
@Override
public float getCacheMissPercentage() {
long cacheMisses = getCacheMisses();
return normalize((float) cacheMisses / (getCacheHits() + cacheMisses)) * 100.0f;
}
@Override
public long getCacheGets() {
return normalize(getHits() + getMisses()
- compensatingCounters.cacheGets
- compensatingCounters.bulkGetHits
- compensatingCounters.bulkGetMiss);
}
@Override
public long getCachePuts() {
return normalize(getBulkCount(BulkOps.PUT_ALL) - compensatingCounters.bulkPuts +
put.sum(EnumSet.of(CacheOperationOutcomes.PutOutcome.PUT)) +
put.sum(EnumSet.of(CacheOperationOutcomes.PutOutcome.UPDATED)) +
putIfAbsent.sum(EnumSet.of(CacheOperationOutcomes.PutIfAbsentOutcome.PUT)) +
replace.sum(EnumSet.of(CacheOperationOutcomes.ReplaceOutcome.HIT)) -
compensatingCounters.cachePuts);
}
@Override
public long getCacheRemovals() {
return normalize(getBulkCount(BulkOps.REMOVE_ALL) - compensatingCounters.bulkRemovals +
remove.sum(EnumSet.of(CacheOperationOutcomes.RemoveOutcome.SUCCESS)) +
conditionalRemove.sum(EnumSet.of(CacheOperationOutcomes.ConditionalRemoveOutcome.SUCCESS)) -
compensatingCounters.cacheRemovals);
}
@Override
public long getCacheEvictions() {
return normalize(authorityEviction.sum(EnumSet.of(StoreOperationOutcomes.EvictionOutcome.SUCCESS)) - compensatingCounters.cacheEvictions);
}
@Override
public float getAverageGetTime() {
return (float) averageGetTime.value();
}
@Override
public float getAveragePutTime() {
return (float) averagePutTime.value();
}
@Override
public float getAverageRemoveTime() {
return (float) averageRemoveTime.value();
}
private long getMisses() {
return getBulkCount(BulkOps.GET_ALL_MISS) +
get.sum(EnumSet.of(CacheOperationOutcomes.GetOutcome.MISS_NO_LOADER, CacheOperationOutcomes.GetOutcome.MISS_WITH_LOADER)) +
putIfAbsent.sum(EnumSet.of(CacheOperationOutcomes.PutIfAbsentOutcome.PUT)) +
replace.sum(EnumSet.of(CacheOperationOutcomes.ReplaceOutcome.MISS_NOT_PRESENT)) +
conditionalRemove.sum(EnumSet.of(CacheOperationOutcomes.ConditionalRemoveOutcome.FAILURE_KEY_MISSING));
}
private long getHits() {
return getBulkCount(BulkOps.GET_ALL_HITS) +
get.sum(EnumSet.of(CacheOperationOutcomes.GetOutcome.HIT_NO_LOADER, CacheOperationOutcomes.GetOutcome.HIT_WITH_LOADER)) +
putIfAbsent.sum(EnumSet.of(CacheOperationOutcomes.PutIfAbsentOutcome.PUT)) +
replace.sum(EnumSet.of(CacheOperationOutcomes.ReplaceOutcome.HIT, CacheOperationOutcomes.ReplaceOutcome.MISS_PRESENT)) +
conditionalRemove.sum(EnumSet.of(CacheOperationOutcomes.ConditionalRemoveOutcome.SUCCESS, CacheOperationOutcomes.ConditionalRemoveOutcome.FAILURE_KEY_PRESENT));
}
private long getBulkCount(BulkOps bulkOps) {
return bulkMethodEntries.get(bulkOps).longValue();
}
private static long normalize(long value) {
return Math.max(0, value);
}
private static float normalize(float value) {
if (Float.isNaN(value)) {
return 0.0f;
}
return Math.min(1.0f, Math.max(0.0f, value));
}
static > OperationStatistic findCacheStatistic(Cache cache, Class type, String statName) {
Query query = queryBuilder()
.children()
.filter(context(attributes(Matchers.