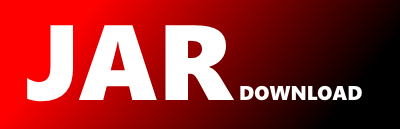
org.ehcache.impl.internal.classes.commonslang.SystemUtils Maven / Gradle / Ivy
Show all versions of ehcache Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* This is a modified version of the original Apache class. It has had unused
* members removed.
*/
package org.ehcache.impl.internal.classes.commonslang;
/**
*
* Helpers for {@code java.lang.System}.
*
*
* If a system property cannot be read due to security restrictions, the corresponding field in this class will be set
* to {@code null} and a message will be written to {@code System.err}.
*
*
* #ThreadSafe#
*
*
* @since 1.0
*/
public class SystemUtils {
/**
*
* The {@code java.specification.version} System Property. Java Runtime Environment specification version.
*
*
* Defaults to {@code null} if the runtime does not have security access to read this property or the property does
* not exist.
*
*
* This value is initialized when the class is loaded. If {@link System#setProperty(String,String)} or
* {@link System#setProperties(java.util.Properties)} is called after this class is loaded, the value will be out of
* sync with that System property.
*
*
* @since Java 1.3
*/
public static final String JAVA_SPECIFICATION_VERSION = getSystemProperty("java.specification.version");
private static final JavaVersion JAVA_SPECIFICATION_VERSION_AS_ENUM = JavaVersion.get(JAVA_SPECIFICATION_VERSION);
// -----------------------------------------------------------------------
/**
*
* Gets a System property, defaulting to {@code null} if the property cannot be read.
*
*
* If a {@code SecurityException} is caught, the return value is {@code null} and a message is written to
* {@code System.err}.
*
*
* @param property the system property name
* @return the system property value or {@code null} if a security problem occurs
*/
private static String getSystemProperty(final String property) {
try {
return System.getProperty(property);
} catch (final SecurityException ex) {
// we are not allowed to look at this property
System.err.println("Caught a SecurityException reading the system property '" + property
+ "'; the SystemUtils property value will default to null.");
return null;
}
}
/**
*
* Is the Java version at least the requested version.
*
*
* Example input:
*
*
* - {@code 1.2f} to test for Java 1.2
* - {@code 1.31f} to test for Java 1.3.1
*
*
* @param requiredVersion the required version, for example 1.31f
* @return {@code true} if the actual version is equal or greater than the required version
*/
public static boolean isJavaVersionAtLeast(final JavaVersion requiredVersion) {
return JAVA_SPECIFICATION_VERSION_AS_ENUM.atLeast(requiredVersion);
}
// -----------------------------------------------------------------------
/**
*
* SystemUtils instances should NOT be constructed in standard programming. Instead, the class should be used as
* {@code SystemUtils.FILE_SEPARATOR}.
*
*
* This constructor is public to permit tools that require a JavaBean instance to operate.
*
*/
public SystemUtils() {
super();
}
}