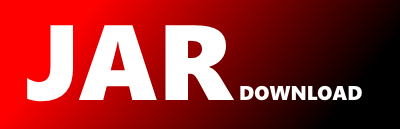
org.ejml.dense.row.mult.MatrixMatrixMult_CDRM Maven / Gradle / Ivy
/*
* Copyright (c) 2009-2017, Peter Abeles. All Rights Reserved.
*
* This file is part of Efficient Java Matrix Library (EJML).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ejml.dense.row.mult;
import org.ejml.MatrixDimensionException;
import org.ejml.data.CMatrixRMaj;
import org.ejml.dense.row.CommonOps_CDRM;
/**
* Matrix multiplication routines for complex row matrices in a row-major format.
*
*
* DO NOT MODIFY! Auto generated by org.ejml.dense.row.mult.GeneratorCMatrixMatrixMult.
*
*
* @author Peter Abeles
*/
@SuppressWarnings("Duplicates")
public class MatrixMatrixMult_CDRM {
public static void mult_reorder(CMatrixRMaj a , CMatrixRMaj b , CMatrixRMaj c )
{
if( a == c || b == c )
throw new IllegalArgumentException("Neither 'a' or 'b' can be the same matrix as 'c'");
else if( a.numCols != b.numRows ) {
throw new MatrixDimensionException("The 'a' and 'b' matrices do not have compatible dimensions");
} else if( a.numRows != c.numRows || b.numCols != c.numCols ) {
throw new MatrixDimensionException("The results matrix does not have the desired dimensions");
}
if( a.numCols == 0 || a.numRows == 0 ) {
CommonOps_CDRM.fill(c,0,0);
return;
}
float realA,imagA;
int indexCbase= 0;
int strideA = a.getRowStride();
int strideB = b.getRowStride();
int strideC = c.getRowStride();
int endOfKLoop = b.numRows*strideB;
for( int i = 0; i < a.numRows; i++ ) {
int indexA = i*strideA;
// need to assign c.data to a value initially
int indexB = 0;
int indexC = indexCbase;
int end = indexB + strideB;
realA = a.data[indexA++];
imagA = a.data[indexA++];
while( indexB < end ) {
float realB = b.data[indexB++];
float imgB = b.data[indexB++];
c.data[indexC++] = realA*realB - imagA*imgB;
c.data[indexC++] = realA*imgB + imagA*realB;
}
// now add to it
while( indexB != endOfKLoop ) { // k loop
indexC = indexCbase;
end = indexB + strideB;
realA = a.data[indexA++];
imagA = a.data[indexA++];
while( indexB < end ) { // j loop
float realB = b.data[indexB++];
float imgB = b.data[indexB++];
c.data[indexC++] += realA*realB - imagA*imgB;
c.data[indexC++] += realA*imgB + imagA*realB;
}
}
indexCbase += strideC;
}
}
public static void mult_small(CMatrixRMaj a , CMatrixRMaj b , CMatrixRMaj c )
{
if( a == c || b == c )
throw new IllegalArgumentException("Neither 'a' or 'b' can be the same matrix as 'c'");
else if( a.numCols != b.numRows ) {
throw new MatrixDimensionException("The 'a' and 'b' matrices do not have compatible dimensions");
} else if( a.numRows != c.numRows || b.numCols != c.numCols ) {
throw new MatrixDimensionException("The results matrix does not have the desired dimensions");
}
int aIndexStart = 0;
int indexC = 0;
int strideA = a.getRowStride();
int strideB = b.getRowStride();
for( int i = 0; i < a.numRows; i++ ) {
for( int j = 0; j < b.numCols; j++ ) {
float realTotal = 0;
float imgTotal = 0;
int indexA = aIndexStart;
int indexB = j*2;
int end = indexA + strideA;
while( indexA < end ) {
float realA = a.data[indexA++];
float imagA = a.data[indexA++];
float realB = b.data[indexB];
float imgB = b.data[indexB+1];
realTotal += realA*realB - imagA*imgB;
imgTotal += realA*imgB + imagA*realB;
indexB += strideB;
}
c.data[indexC++] = realTotal;
c.data[indexC++] = imgTotal;
}
aIndexStart += strideA;
}
}
public static void multTransA_reorder(CMatrixRMaj a , CMatrixRMaj b , CMatrixRMaj c )
{
if( a == c || b == c )
throw new IllegalArgumentException("Neither 'a' or 'b' can be the same matrix as 'c'");
else if( a.numRows != b.numRows ) {
throw new MatrixDimensionException("The 'a' and 'b' matrices do not have compatible dimensions");
} else if( a.numCols != c.numRows || b.numCols != c.numCols ) {
throw new MatrixDimensionException("The results matrix does not have the desired dimensions");
}
if( a.numCols == 0 || a.numRows == 0 ) {
CommonOps_CDRM.fill(c,0,0);
return;
}
float realA,imagA;
for( int i = 0; i < a.numCols; i++ ) {
int indexC_start = i*c.numCols*2;
// first assign R
realA = a.data[i*2];
imagA = a.data[i*2+1];
int indexB = 0;
int end = indexB+b.numCols*2;
int indexC = indexC_start;
while( indexB
© 2015 - 2025 Weber Informatics LLC | Privacy Policy