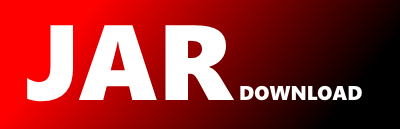
org.ejml.simple.SimpleMatrix Maven / Gradle / Ivy
Show all versions of ejml-simple Show documentation
/*
* Copyright (c) 2023, Peter Abeles. All Rights Reserved.
*
* This file is part of Efficient Java Matrix Library (EJML).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ejml.simple;
import org.ejml.data.*;
import org.ejml.dense.row.*;
import org.ejml.ops.DConvertMatrixStruct;
import org.ejml.ops.FConvertMatrixStruct;
import java.util.Random;
import java.util.concurrent.ThreadLocalRandom;
/**
*
* {@link SimpleMatrix} is a wrapper around a primitive matrix type
* (for example, {@link DMatrixRMaj} or {@link FMatrixSparseCSC}) that provides an
* easy to use object oriented interface for performing matrix operations. It is designed to be
* more accessible to novice programmers and provide a way to rapidly code up solutions by simplifying
* memory management and providing easy to use functions.
*
*
*
* Most functions in SimpleMatrix do not modify the original matrix. Instead they
* create a new SimpleMatrix instance which is modified and returned. This greatly simplifies memory
* management and writing of code in general. It also allows operations to be chained, as is shown
* below:
*
* {@code SimpleMatrix K = P.mult(H.transpose().mult(S.invert()));}
*
*
*
* Working with both a primitive matrix and SimpleMatrix in the same code base is easy.
* To access the internal Matrix in a SimpleMatrix simply call {@link SimpleMatrix#getMatrix()}.
* To turn a Matrix into a SimpleMatrix use {@link SimpleMatrix#wrap(org.ejml.data.Matrix)}. Not
* all operations in EJML are provided for SimpleMatrix, but can be accessed by extracting the internal
* matrix.
*
*
*
* The object oriented approach used in SimpleMatrix was originally inspired by
* JAMA.
*
*
* Extending
*
* SimpleMatrix contains a list of narrowly focused functions for linear algebra. To harness
* the functionality for another application and to the number of functions it supports it is recommended
* that one extends {@link SimpleBase} instead. This way the returned matrix type's of SimpleMatrix functions
* will be of the appropriate types. See StatisticsMatrix inside of the examples directory.
*
*
*
* If SimpleMatrix is extended then the protected function {@link #createMatrix} should be extended and return
* the child class. The results of SimpleMatrix operations will then be of the correct matrix type.
*
*
* Performance
*
* The disadvantage of using this class is that it is more resource intensive, since
* it creates a new matrix each time an operation is performed. This makes the JavaVM work harder and
* Java automatically initializes the matrix to be all zeros. Typically operations on small matrices
* or operations that have a runtime linear with the number of elements are the most affected. More
* computationally intensive operations have only a slight unnoticeable performance loss. MOST PEOPLE
* SHOULD NOT WORRY ABOUT THE SLIGHT LOSS IN PERFORMANCE.
*
*
*
* It is hard to judge how significant the performance hit will be in general. Often the performance
* hit is insignificant since other parts of the application are more processor intensive or the bottle
* neck is a more computationally complex operation. The best approach is benchmark and then optimize the code.
*
*
* Creating matrices
*
* Method Description
* {@link #SimpleMatrix(int, int, Class)}
* Create a matrix filled with zeros with the specified internal type.
* {@link #SimpleMatrix(int, int, MatrixType)}
* Create a matrix filled with zeros with the specified internal matrix type.
* {@link #SimpleMatrix(int, int)}
* Create a matrix filled with zeros.
* {@link #SimpleMatrix(int, int, boolean, double...)}
* Create a matrix with the provided double values, in either row-major or column-major order.
* {@link #SimpleMatrix(int, int, boolean, float...)}
* Create a matrix with the provided float values, in either row-major or column-major order.
* {@link #SimpleMatrix(double[][])}
* Create a matrix from a 2D double array.
* {@link #SimpleMatrix(float[][])}
* Create a matrix from a 2D float array.
* {@link #SimpleMatrix(double[])}
* Create a column vector from a 1D double array.
* {@link #SimpleMatrix(float[])}
* Create a column vector from a 1D float array.
* {@link #SimpleMatrix(Matrix)}
* Create a matrix copying the provided Matrix.
* {@link #SimpleMatrix(SimpleMatrix)}
* Create a matrix copying the provided SimpleMatrix.
* {@link #wrap(Matrix)}
* Create a matrix wrapping the provided Matrix.
* {@link #filled(int, int, double)}
* Create a matrix filled with the specified value.
* {@link #ones(int, int)}
* Create a matrix filled with ones.
* {@link #diag(double...)}
* Create a diagonal matrix.
* {@link #diag(Class, double...)}
* Create a diagonal matrix with the specified internal type.
* {@link #identity(int)}
* Create an identity matrix.
* {@link #identity(int, Class)}
* Create an identity matrix with the specified internal type.
* {@link #random(int, int)}
* Create a random {@link DMatrixRMaj} with values drawn from a continuous uniform distribution on the
* unit interval.
* {@link #random_DDRM(int, int, double, double, Random)}
* Create a random {@link DMatrixRMaj} with values drawn from a continuous uniform distribution using the
* provided random number generator.
* {@link #random_DDRM(int, int)}
* Create a random {@link DMatrixRMaj} with values drawn from a continuous uniform distribution on the
* unit interval.
* {@link #random_FDRM(int, int, float, float, Random)}
* Create a random {@link FMatrixRMaj} with values drawn from a continuous uniform distribution using the
* provided random number generator.
* {@link #random_FDRM(int, int)}
* Create a random {@link FMatrixRMaj} with values drawn from a continuous uniform distribution on the
* unit interval.
* {@link #random_ZDRM(int, int, double, double, Random)}
* Create a random {@link ZMatrixRMaj} with values drawn from a continuous uniform distribution using the
* provided random number generator.
* {@link #random_ZDRM(int, int)}
* Create a random {@link ZMatrixRMaj} with values drawn from a continuous uniform distribution on the
* unit interval.
* {@link #random_CDRM(int, int, float, float, Random)}
* Create a random {@link CMatrixRMaj} with values drawn from a continuous uniform distribution using the
* provided random number generator.
* {@link #random_CDRM(int, int)}
* Create a random {@link CMatrixRMaj} with values drawn from a continuous uniform distribution on the
* unit interval.
* {@link #randomNormal(SimpleMatrix, Random)}
* Create a random vector drawn from a multivariate normal distribution
* with the specified covariance.
* {@link #createLike()}
* Create a matrix with the same shape and internal type as this matrix.
* {@link #copy()}
* Create a copy of this matrix.
*
*
* Getting elements, rows and columns
*
* Method Description
* {@link #get(int)}
* Get the value of the {@code i}th entry in row-major order.
* {@link #get(int, int)}
* Get the value of the {@code i,j}th entry.
* {@link #get(int, int, Complex_F64)}
* Get the value of the {@code i,j}th entry as a complex number.
* {@link #getReal(int, int)}
* Get the real component of the {@code i,j}th entry.
* {@link #getImaginary(int, int)}
* Get the imaginary component of the {@code i,j}th entry.
* {@link #getImag(int, int)}
* Alias for {@link #getImaginary(int, int)}
* {@link #getRow(int)}
* Get the {@code i}th row.
* {@link #getColumn(int)}
* Get the {@code j}th column.
* {@link #extractVector(boolean, int)}
* Extract the specified row or column vector.
* {@link #extractMatrix(int, int, int, int)}
* Extract the specified submatrix.
* {@link #rows(int, int)}
* Extract the specified rows.
* {@link #cols(int, int)}
* Extract the specified columns.
* {@link #diag()}
* Extract the matrix diagonal, or construct a diagonal matrix from a vector.
*
*
* Setting elements, rows and columns
*
* Method Description
* {@link #set(int, double)}
* Set the value of the {@code i}th entry in row-major order.
* {@link #set(int, int, double)}
* Set the value of the {@code i,j}th entry.
* {@link #set(int, int, Complex_F64)}
* Set the value of the {@code i,j}th entry as a complex number.
* {@link #set(int, int, double, double)}
* Set the real and imaginary components of the {@code i,j}th entry.
* {@link #setRow(int, ConstMatrix)}
* Set the {@code i}th row.
* {@link #setRow(int, int, double...)}
* Set the values in the {@code i}th row.
* {@link #setColumn(int, ConstMatrix)}
* Set the {@code j}th column.
* {@link #setColumn(int, int, double...)}
* Set the values in the {@code j}th column.
* {@link #setTo(SimpleBase)}
* Set the elements of this matrix to be equal to elements from another matrix.
* {@link #insertIntoThis(int, int, SimpleBase)}
* Insert values from another matrix, starting in position {@code i,j}.
* {@link #fill(double)}
* Set all elements of this matrix to be equal to specified value.
* {@link #fillComplex(double, double)}
* Set all elements of this matrix to be equal to specified complex value.
* {@link #zero()}
* Set all elements of this matrix to zero.
*
*
* Basic operations
*
* Method Description
* {@link #plus(double)}
* Add a scalar value.
* {@link #plusComplex(double, double)}
* Add a complex scalar value.
* {@link #plus(ConstMatrix)}
* Add another matrix.
* {@link #plus(double, ConstMatrix)}
* Add another matrix, first applying the specified scale factor.
* {@link #minus(double)}
* Subtract a scalar value.
* {@link #minusComplex(double, double)}
* Subtract a complex scalar value.
* {@link #minus(ConstMatrix)}
* Subtract another matrix.
* {@link #scale(double)}
* Multiply by a scalar value.
* {@link #scaleComplex(double, double)}
* Multiply by a complex scalar value.
* {@link #divide(double)}
* Divide by a scalar value.
* {@link #mult(ConstMatrix)}
* Multiply with another matrix.
* {@link #dot(ConstMatrix)}
* Calculate the dot product with another vector.
* {@link #negative()}
* Get the negative of each entry.
* {@link #real()}
* Get the real component of each entry.
* {@link #imaginary()}
* Get the imaginary component of each entry.
* {@link #imag()}
* Alias for {@link #imaginary()}.
* {@link #magnitude()}
* Get the imaginary component of each entry.
* {@link #transpose()}
* Get the transpose.
* {@link #transposeConjugate()}
* Get the conjugate transpose.
* {@link #equation(String, Object...)}
* Perform an equation in place on the matrix.
*
*
* Elementwise operations
*
* Method Description
* {@link #elementMult(ConstMatrix)}
* Perform element by element multiplication with another matrix.
* {@link #elementDiv(ConstMatrix)}
* Perform element by element division with another matrix.
* {@link #elementPower(double)}
* Raise each entry to the specified power.
* {@link #elementPower(ConstMatrix)}
* Raise each entry to the corresponding power in another matrix.
* {@link #elementExp()}
* Compute the exponent of each entry.
* {@link #elementLog()}
* Compute the logarithm of each entry.
* {@link #elementOp(SimpleOperations.ElementOpReal)}
* Apply the specified real-valued function to each entry.
* {@link #elementOp(SimpleOperations.ElementOpComplex)}
* Apply the specified complex-valued function to each entry.
*
*
* Aggregations
*
* Method Description
* {@link #elementSum()}
* Compute the sum of all elements of this matrix.
* {@link #elementSumComplex()}
* Compute the sum of all elements of a complex matrix.
* {@link #elementMax()}
* Compute the maximum of all elements of this matrix.
* {@link #elementMaxAbs()}
* Compute the maximum absolute value of all elements of this matrix.
* {@link #elementMin()}
* Compute the minimum of all elements of this matrix.
* {@link #elementMinAbs()}
* Compute the minimum absolute value of all elements of this matrix.
*
*
* Linear algebra
*
* Method Description
* {@link #solve(ConstMatrix)}
* Solve the equation {@code Ax = b}.
* {@link #conditionP2()}
* Compute the matrix condition number.
* {@link #invert()}
* Compute the matrix inverse.
* {@link #pseudoInverse()}
* Compute the Moore-Penrose pseudo-inverse.
* {@link #determinant()}
* Compute the determinant.
* {@link #determinantComplex()}
* Compute the determinant of a complex matrix.
* {@link #trace()}
* Compute the trace.
* {@link #traceComplex()}
* Compute the trace of a complex matrix.
* {@link #normF()}
* Compute the Frobenius norm.
* {@link #eig()}
* Compute the eigenvalue decomposition.
* {@link #svd()}
* Compute the singular value decomposition.
* {@link #svd(boolean)}
* Compute the singular value decomposition in compact or full format.
*
*
* Combining matrices
*
* Method Description
* {@link #combine(int, int, ConstMatrix)}
* Combine with another matrix.
* {@link #concatRows(ConstMatrix...)}
* Concatenate vertically with one or more other matrices.
* {@link #concatColumns(ConstMatrix...)}
* Concatenate horizontally with one or more other matrices.
* {@link #kron(ConstMatrix)}
* Compute the Kronecker product with another matrix.
*
*
* Matrix properties
*
* Method Description
* {@link #getNumRows()}
* Get the number of rows.
* {@link #getNumCols()}
* Get the number of columns.
* {@link #getNumElements()}
* Get the number of elements.
* {@link #bits()}
* Get the size of the internal array elements (32 or 64).
* {@link #isVector()}
* Check if this matrix is a vector.
* {@link #isIdentical(ConstMatrix, double)}
* Check if this matrix is the same as another matrix, up to the specified tolerance.
* {@link #hasUncountable()}
* Check if any of the matrix elements are NaN or infinite.
*
*
* Converting and reshaping
*
* Method Description
* {@link #convertToComplex()}
* Convert to a complex matrix.
* {@link #convertToDense()}
* Convert to a dense matrix.
* {@link #convertToSparse()}
* Convert to a sparse matrix.
* {@link #reshape(int, int)}
* Change the number of rows and columns.
*
*
* Accessing the internal matrix
*
* Method Description
* {@link #getType()}
* Get the type of the wrapped matrix.
* {@link #getMatrix()}
* Get the wrapped matrix.
* {@link #getDDRM()}
* Get the wrapped matrix as a {@link DMatrixRMaj}.
* {@link #getFDRM()}
* Get the wrapped matrix as a {@link FMatrixRMaj}.
* {@link #getZDRM()}
* Get the wrapped matrix as a {@link ZMatrixRMaj}.
* {@link #getCDRM()}
* Get the wrapped matrix as a {@link CMatrixRMaj}.
* {@link #getDSCC()}
* Get the wrapped matrix as a {@link DMatrixSparseCSC}.
* {@link #getFSCC()}
* Get the wrapped matrix as a {@link FMatrixSparseCSC}.
*
*
* Loading and saving
*
* Method Description
* {@link #loadCSV(String)}
* Load a matrix from a CSV file.
* {@link #saveToFileCSV(String)}
* Save this matrix to a CSV file.
* {@link #saveToMatrixMarket(String)}
* Save this matrix in matrix market format.
*
*
* Miscellaneous
*
* Method Description
* {@link #iterator(boolean, int, int, int, int)}
* Create an iterator for traversing a submatrix.
* {@link #getIndex(int, int)}
* Get the row-major index corresponding to {@code i,j}.
* {@link #isInBounds(int, int)}
* Check if the indices {@code i,j} are in bounds.
* {@link #toString()}
* Get the string representation of the matrix.
* {@link #toArray2()}
* Convert the matrix to a 2D array of doubles.
* {@link #print()}
* Print the matrix to standard out.
* {@link #print(String)}
* Print the matrix to standard out using the specified floating point format.
* {@link #printDimensions()}
* Print the number of rows and columns.
*
*
* @author Peter Abeles
*/
public class SimpleMatrix extends SimpleBase {
/**
* A simplified way to reference the last row or column in the matrix for some functions.
*/
public static final int END = Integer.MAX_VALUE;
/**
*
* Creates a new matrix which has the same value as the matrix encoded in the
* provided array. The input matrix's format can either be row-major or
* column-major.
*
*
*
* Note that 'data' is a variable argument type, so either 1D arrays or a set of numbers can be
* passed in:
* SimpleMatrix a = new SimpleMatrix(2,2,true,new double[]{1,2,3,4});
* SimpleMatrix b = new SimpleMatrix(2,2,true,1,2,3,4);
*
* Both are equivalent.
*
*
* @param numRows The number of rows.
* @param numCols The number of columns.
* @param rowMajor If the array is encoded in a row-major or a column-major format.
* @param data The formatted 1D array. Not modified.
* @see DMatrixRMaj#DMatrixRMaj(int, int, boolean, double...)
*/
public SimpleMatrix( int numRows, int numCols, boolean rowMajor, double... data ) {
setMatrix(new DMatrixRMaj(numRows, numCols, rowMajor, data));
}
/**
*
* Creates a new matrix which has the same value as the matrix encoded in the
* provided array. The input matrix's format can either be row-major or
* column-major.
*
*
*
* Note that 'data' is a variable argument type, so either 1D arrays or a set of numbers can be
* passed in:
* SimpleMatrix a = new SimpleMatrix(2,2,true,new float[]{1,2,3,4});
* SimpleMatrix b = new SimpleMatrix(2,2,true,1,2,3,4);
*
* Both are equivalent.
*
*
* @param numRows The number of rows.
* @param numCols The number of columns.
* @param rowMajor If the array is encoded in a row-major or a column-major format.
* @param data The formatted 1D array. Not modified.
* @see FMatrixRMaj#FMatrixRMaj(int, int, boolean, float...)
*/
public SimpleMatrix( int numRows, int numCols, boolean rowMajor, float... data ) {
setMatrix(new FMatrixRMaj(numRows, numCols, rowMajor, data));
}
/**
*
* Creates a matrix with the values and shape defined by the 2D array 'data'.
* It is assumed that 'data' has a row-major formatting:
*
* data[ row ][ column ]
*
*
* @param data 2D array representation of the matrix. Not modified.
* @see DMatrixRMaj#DMatrixRMaj(double[][])
*/
public SimpleMatrix( double[][] data ) {
setMatrix(new DMatrixRMaj(data));
}
/**
*
* Creates a matrix with the values and shape defined by the 2D array 'data'.
* It is assumed that 'data' has a row-major formatting:
*
* data[ row ][ column ]
*
*
* @param data 2D array representation of the matrix. Not modified.
* @see FMatrixRMaj#FMatrixRMaj(float[][])
*/
public SimpleMatrix( float[][] data ) {
setMatrix(new FMatrixRMaj(data));
}
/**
* Creates a column vector with the values and shape defined by the 1D array 'data'.
*
* @param data 1D array representation of the vector. Not modified.
*/
public SimpleMatrix( double[] data ) {
setMatrix(new DMatrixRMaj(data.length, 1, true, data));
}
/**
* Creates a column vector with the values and shape defined by the 1D array 'data'.
*
* @param data 1D array representation of the vector. Not modified.
*/
public SimpleMatrix( float[] data ) {
setMatrix(new FMatrixRMaj(data.length, 1, true, data));
}
/**
* Creates a new matrix that is initially set to zero with the specified dimensions. This will wrap a
* {@link DMatrixRMaj}.
*
* @param numRows The number of rows in the matrix.
* @param numCols The number of columns in the matrix.
*/
public SimpleMatrix( int numRows, int numCols ) {
setMatrix(new DMatrixRMaj(numRows, numCols));
}
/**
* Creates a new matrix that is initially set to zero with the specified dimensions and type.
*
* @param numRows The number of rows in the matrix.
* @param numCols The number of columns in the matrix.
* @param type The matrix type
*/
public SimpleMatrix( int numRows, int numCols, Class> type ) {
this(numRows, numCols, MatrixType.lookup(type));
}
/**
* Creates a new matrix that is initially set to zero with the specified dimensions and matrix type.
*
* @param numRows The number of rows in the matrix.
* @param numCols The number of columns in the matrix.
* @param type The matrix type
*/
public SimpleMatrix( int numRows, int numCols, MatrixType type ) {
switch (type) {
case DDRM -> setMatrix(new DMatrixRMaj(numRows, numCols));
case FDRM -> setMatrix(new FMatrixRMaj(numRows, numCols));
case ZDRM -> setMatrix(new ZMatrixRMaj(numRows, numCols));
case CDRM -> setMatrix(new CMatrixRMaj(numRows, numCols));
case DSCC -> setMatrix(new DMatrixSparseCSC(numRows, numCols));
case FSCC -> setMatrix(new FMatrixSparseCSC(numRows, numCols));
default -> throw new RuntimeException("Unknown matrix type");
}
}
/**
* Creates a new SimpleMatrix which is identical to the original.
*
* @param orig The matrix which is to be copied. Not modified.
*/
public SimpleMatrix( SimpleMatrix orig ) {
setMatrix(orig.mat.copy());
}
/**
* Creates a new SimpleMatrix which is a copy of the Matrix.
*
* @param orig The original matrix whose value is copied. Not modified.
*/
public SimpleMatrix( Matrix orig ) {
Matrix mat;
if (orig instanceof DMatrixRBlock) {
var a = new DMatrixRMaj(orig.getNumRows(), orig.getNumCols());
DConvertMatrixStruct.convert((DMatrixRBlock)orig, a);
mat = a;
} else if (orig instanceof FMatrixRBlock) {
var a = new FMatrixRMaj(orig.getNumRows(), orig.getNumCols());
FConvertMatrixStruct.convert((FMatrixRBlock)orig, a);
mat = a;
} else {
mat = orig.copy();
}
setMatrix(mat);
}
/**
* Constructor for internal library use only. Nothing is configured and is intended for serialization.
*/
protected SimpleMatrix() {}
/**
* Creates a new SimpleMatrix with the specified Matrix used as its internal matrix. This means
* that the reference is saved and calls made to the returned SimpleMatrix will modify the passed in Matrix.
*
* @param internalMat The internal Matrix of the returned SimpleMatrix. Will be modified.
*/
public static SimpleMatrix wrap( Matrix internalMat ) {
var ret = new SimpleMatrix();
ret.setMatrix(internalMat);
return ret;
}
/**
* Creates a new matrix filled with the specified value. This will wrap a {@link DMatrixRMaj}.
*
* @param numRows The number of rows in the matrix.
* @param numCols The number of columns in the matrix.
* @param a The value to fill the matrix with.
* @return A matrix filled with the value a.
*/
public static SimpleMatrix filled( int numRows, int numCols, double a ) {
var res = new SimpleMatrix(numRows, numCols);
res.fill(a);
return res;
}
/**
* Creates a new matrix filled with ones. This will wrap a {@link DMatrixRMaj}.
*
* @param numRows The number of rows in the matrix.
* @param numCols The number of columns in the matrix.
* @return A matrix of ones.
*/
public static SimpleMatrix ones( int numRows, int numCols ) {
return filled(numRows, numCols, 1);
}
/**
* Creates a new identity matrix with the specified size. This will wrap a {@link DMatrixRMaj}.
*
* @param width The width and height of the matrix.
* @return An identity matrix.
* @see CommonOps_DDRM#identity(int)
*/
public static SimpleMatrix identity( int width ) {
return identity(width, DMatrixRMaj.class);
}
/**
* Creates a new identity matrix with the specified size and type.
*
* @param width The width and height of the matrix.
* @param type The matrix type
* @return An identity matrix.
*/
public static SimpleMatrix identity( int width, Class> type ) {
var ret = new SimpleMatrix(width, width, type);
ret.ops.setIdentity(ret.mat);
return ret;
}
/**
*
* Creates a matrix where all but the diagonal elements are zero. The values
* of the diagonal elements are specified by the parameter 'vals'. This will wrap a {@link DMatrixRMaj}.
*
*
*
* To extract the diagonal elements from a matrix see {@link #diag()}.
*
*
* @param vals The values of the diagonal elements.
* @return A diagonal matrix.
* @see CommonOps_DDRM#diag(double...)
*/
public static SimpleMatrix diag( double... vals ) {
return wrap(CommonOps_DDRM.diag(vals));
}
/**
* Creates a matrix where all but the diagonal elements are zero. The values
* of the diagonal elements are specified by the parameter 'vals'.
*
* @param type The matrix type
* @param vals The values of the diagonal elements.
* @return A diagonal matrix.
*/
public static SimpleMatrix diag( Class> type, double... vals ) {
var M = new SimpleMatrix(vals.length, vals.length, type);
for (int i = 0; i < vals.length; i++) {
M.set(i, i, vals[i]);
}
return M;
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from minValue (inclusive) to
* maxValue (exclusive). This will wrap a {@link DMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
* @param minValue Lower bound
* @param maxValue Upper bound
* @param rand The random number generator that's used to fill the matrix.
* @return The new random matrix.
* @see RandomMatrices_DDRM#fillUniform(DMatrixD1, double, double, java.util.Random)
*/
public static SimpleMatrix random_DDRM( int numRows, int numCols, double minValue, double maxValue, Random rand ) {
var ret = new SimpleMatrix(numRows, numCols);
RandomMatrices_DDRM.fillUniform((DMatrixRMaj)ret.mat, minValue, maxValue, rand);
return ret;
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from 0.0 (inclusive) to
* 1.0 (exclusive).
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
* @see #random_DDRM(int, int)
*/
public static SimpleMatrix random( int numRows, int numCols ) {
return random_DDRM(numRows, numCols, 0.0, 1.0, ThreadLocalRandom.current());
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from 0.0 (inclusive) to
* 1.0 (exclusive).
* The random number generator is {@link ThreadLocalRandom#current()}. This will wrap a {@link DMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
*/
public static SimpleMatrix random_DDRM( int numRows, int numCols ) {
return random_DDRM(numRows, numCols, 0.0, 1.0, ThreadLocalRandom.current());
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from minValue (inclusive) to
* maxValue (exclusive). This will wrap a {@link FMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
* @param minValue Lower bound
* @param maxValue Upper bound
* @param rand The random number generator that's used to fill the matrix.
* @return The new random matrix.
* @see RandomMatrices_FDRM#fillUniform(FMatrixD1, float, float, java.util.Random)
*/
public static SimpleMatrix random_FDRM( int numRows, int numCols, float minValue, float maxValue, Random rand ) {
var ret = new SimpleMatrix(numRows, numCols, FMatrixRMaj.class);
RandomMatrices_FDRM.fillUniform((FMatrixRMaj)ret.mat, minValue, maxValue, rand);
return ret;
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from 0.0 (inclusive) to
* 1.0 (exclusive). The random number generator is {@link ThreadLocalRandom#current()}.
* This will wrap a {@link FMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
*/
public static SimpleMatrix random_FDRM( int numRows, int numCols ) {
return random_FDRM(numRows, numCols, 0.0f, 1.0f, ThreadLocalRandom.current());
}
/**
* Creates a random matrix with real and complex components drawn from the continuous uniform distribution from
* minValue (inclusive) to maxValue (exclusive). This will wrap a {@link ZMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
* @param minValue Lower bound
* @param maxValue Upper bound
* @param rand The random number generator that's used to fill the matrix.
* @return The new random matrix.
* @see RandomMatrices_ZDRM#fillUniform(ZMatrixD1, double, double, java.util.Random)
*/
public static SimpleMatrix random_ZDRM( int numRows, int numCols, double minValue, double maxValue, Random rand ) {
var ret = new SimpleMatrix(numRows, numCols, MatrixType.ZDRM);
RandomMatrices_ZDRM.fillUniform((ZMatrixRMaj)ret.mat, minValue, maxValue, rand);
return ret;
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from 0.0 (inclusive) to
* 1.0 (exclusive). The random number generator is {@link ThreadLocalRandom#current()}.
* This will wrap a {@link ZMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
*/
public static SimpleMatrix random_ZDRM( int numRows, int numCols ) {
return random_ZDRM(numRows, numCols, 0.0, 1.0, ThreadLocalRandom.current());
}
/**
* Creates a random matrix with real and complex components drawn from the continuous uniform distribution from
* minValue (inclusive) to maxValue (exclusive). This will wrap a {@link CMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
* @param minValue Lower bound
* @param maxValue Upper bound
* @param rand The random number generator that's used to fill the matrix.
* @return The new random matrix.
* @see RandomMatrices_CDRM#fillUniform(CMatrixD1, float, float, java.util.Random)
*/
public static SimpleMatrix random_CDRM( int numRows, int numCols, float minValue, float maxValue, Random rand ) {
var ret = new SimpleMatrix(numRows, numCols, MatrixType.CDRM);
RandomMatrices_CDRM.fillUniform((CMatrixRMaj)ret.mat, minValue, maxValue, rand);
return ret;
}
/**
* Creates a random matrix with values drawn from the continuous uniform distribution from 0.0 (inclusive) to
* 1.0 (exclusive). The random number generator is {@link ThreadLocalRandom#current()}.
* This will wrap a {@link CMatrixRMaj}.
*
* @param numRows The number of rows in the new matrix
* @param numCols The number of columns in the new matrix
*/
public static SimpleMatrix random_CDRM( int numRows, int numCols ) {
return random_CDRM(numRows, numCols, 0.0f, 1.0f, ThreadLocalRandom.current());
}
/**
*
* Creates a new vector which is drawn from a multivariate normal distribution with zero mean
* and the provided covariance.
*
*
* @param covariance Covariance of the multivariate normal distribution
* @param random The random number generator that's used to fill the matrix.
* @return Vector randomly drawn from the distribution
* @see CovarianceRandomDraw_DDRM
*/
public static SimpleMatrix randomNormal( SimpleMatrix covariance, Random random ) {
var found = new SimpleMatrix(covariance.numRows(), 1, covariance.getType());
switch (found.getType()) {
case DDRM -> {
var draw = new CovarianceRandomDraw_DDRM(random, covariance.getMatrix());
draw.next(found.getMatrix());
}
case FDRM -> {
var draw = new CovarianceRandomDraw_FDRM(random, covariance.getMatrix());
draw.next(found.getMatrix());
}
default -> throw new IllegalArgumentException("Matrix type is currently not supported");
}
return found;
}
@Override
protected SimpleMatrix createMatrix( int numRows, int numCols, MatrixType type ) {
return new SimpleMatrix(numRows, numCols, type);
}
@Override
protected SimpleMatrix wrapMatrix( Matrix m ) {
return new SimpleMatrix(m);
}
// TODO should this function be added back? It makes the code hard to read when its used
// /**
// *
// * Performs one of the following matrix multiplication operations:
// *
// * c = a * b
// * c = aT * b
// * c = a * b T
// * c = aT * b T
// *
// * where c is the returned matrix, a is this matrix, and b is the passed in matrix.
// *
// *
// * @see CommonOps#mult(DMatrixRMaj, DMatrixRMaj, DMatrixRMaj)
// * @see CommonOps#multTransA(DMatrixRMaj, DMatrixRMaj, DMatrixRMaj)
// * @see CommonOps#multTransB(DMatrixRMaj, DMatrixRMaj, DMatrixRMaj)
// * @see CommonOps#multTransAB(DMatrixRMaj, DMatrixRMaj, DMatrixRMaj)
// *
// * @param tranA If true matrix A is transposed.
// * @param tranB If true matrix B is transposed.
// * @param b A matrix that is n by bn. Not modified.
// *
// * @return The results of this operation.
// */
// public SimpleMatrix mult( boolean tranA , boolean tranB , SimpleMatrix b) {
// SimpleMatrix ret;
//
// if( tranA && tranB ) {
// ret = createMatrix(mat.numCols,b.mat.numRows);
// CommonOps.multTransAB(mat,b.mat,ret.mat);
// } else if( tranA ) {
// ret = createMatrix(mat.numCols,b.mat.numCols);
// CommonOps.multTransA(mat,b.mat,ret.mat);
// } else if( tranB ) {
// ret = createMatrix(mat.numRows,b.mat.numRows);
// CommonOps.multTransB(mat,b.mat,ret.mat);
// } else {
// ret = createMatrix(mat.numRows,b.mat.numCols);
// CommonOps.mult(mat,b.mat,ret.mat);
// }
//
// return ret;
// }
}