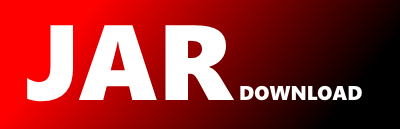
org.elasticsearch.client.SearchableSnapshotsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.admin.cluster.snapshots.restore.RestoreSnapshotResponse;
import org.elasticsearch.client.searchable_snapshots.CachesStatsRequest;
import org.elasticsearch.client.searchable_snapshots.CachesStatsResponse;
import org.elasticsearch.client.searchable_snapshots.MountSnapshotRequest;
import java.io.IOException;
import java.util.Collections;
import java.util.Objects;
/**
* A wrapper for the {@link RestHighLevelClient} that provides methods for accessing searchable snapshots APIs.
*
* See the Searchable Snapshots
* APIs on elastic.co for more information.
*
* @deprecated The High Level Rest Client is deprecated in favor of the
*
* Elasticsearch Java API Client
*/
@Deprecated
@SuppressWarnings("removal")
public class SearchableSnapshotsClient {
private RestHighLevelClient restHighLevelClient;
public SearchableSnapshotsClient(final RestHighLevelClient restHighLevelClient) {
this.restHighLevelClient = Objects.requireNonNull(restHighLevelClient);
}
/**
* Executes the mount snapshot API, which mounts a snapshot as a searchable snapshot.
*
* See the
* docs for more information.
*
* @param request the request
* @param options the request options
* @return the response
* @throws IOException if an I/O exception occurred sending the request, or receiving or parsing the response
*/
public RestoreSnapshotResponse mountSnapshot(final MountSnapshotRequest request, final RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
SearchableSnapshotsRequestConverters::mountSnapshot,
options,
RestoreSnapshotResponse::fromXContent,
Collections.emptySet()
);
}
/**
* Asynchronously executes the mount snapshot API, which mounts a snapshot as a searchable snapshot.
*
* @param request the request
* @param options the request options
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable mountSnapshotAsync(
final MountSnapshotRequest request,
final RequestOptions options,
final ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
SearchableSnapshotsRequestConverters::mountSnapshot,
options,
RestoreSnapshotResponse::fromXContent,
listener,
Collections.emptySet()
);
}
/**
* Executes the cache stats API, which provides statistics about searchable snapshot cache.
*
* See the
* docs for more information.
*
* @param request the request
* @param options the request options
* @return the response
* @throws IOException if an I/O exception occurred sending the request, or receiving or parsing the response
*/
public CachesStatsResponse cacheStats(final CachesStatsRequest request, final RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
SearchableSnapshotsRequestConverters::cacheStats,
options,
CachesStatsResponse::fromXContent,
Collections.emptySet()
);
}
/**
* Asynchronously executes the cache stats API, which provides statistics about searchable snapshot cache.
*
* @param request the request
* @param options the request options
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable cacheStatsAsync(
final CachesStatsRequest request,
final RequestOptions options,
final ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
SearchableSnapshotsRequestConverters::cacheStats,
options,
CachesStatsResponse::fromXContent,
listener,
Collections.emptySet()
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy