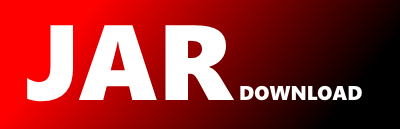
org.elasticsearch.client.WatcherClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.support.master.AcknowledgedResponse;
import org.elasticsearch.client.watcher.AckWatchRequest;
import org.elasticsearch.client.watcher.AckWatchResponse;
import org.elasticsearch.client.watcher.ActivateWatchRequest;
import org.elasticsearch.client.watcher.ActivateWatchResponse;
import org.elasticsearch.client.watcher.DeactivateWatchRequest;
import org.elasticsearch.client.watcher.DeactivateWatchResponse;
import org.elasticsearch.client.watcher.DeleteWatchRequest;
import org.elasticsearch.client.watcher.DeleteWatchResponse;
import org.elasticsearch.client.watcher.ExecuteWatchRequest;
import org.elasticsearch.client.watcher.ExecuteWatchResponse;
import org.elasticsearch.client.watcher.GetWatchRequest;
import org.elasticsearch.client.watcher.GetWatchResponse;
import org.elasticsearch.client.watcher.PutWatchRequest;
import org.elasticsearch.client.watcher.PutWatchResponse;
import org.elasticsearch.client.watcher.StartWatchServiceRequest;
import org.elasticsearch.client.watcher.StopWatchServiceRequest;
import org.elasticsearch.client.watcher.WatcherStatsRequest;
import org.elasticsearch.client.watcher.WatcherStatsResponse;
import java.io.IOException;
import static java.util.Collections.emptySet;
import static java.util.Collections.singleton;
/**
* @deprecated The High Level Rest Client is deprecated in favor of the
*
* Elasticsearch Java API Client
*/
@Deprecated
@SuppressWarnings("removal")
public final class WatcherClient {
private final RestHighLevelClient restHighLevelClient;
WatcherClient(RestHighLevelClient restHighLevelClient) {
this.restHighLevelClient = restHighLevelClient;
}
/**
* Start the watch service
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public AcknowledgedResponse startWatchService(StartWatchServiceRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::startWatchService,
options,
AcknowledgedResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously start the watch service
* See
* the docs for more.
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return cancellable that may be used to cancel the request
*/
public Cancellable startWatchServiceAsync(
StartWatchServiceRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::startWatchService,
options,
AcknowledgedResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Stop the watch service
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public AcknowledgedResponse stopWatchService(StopWatchServiceRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::stopWatchService,
options,
AcknowledgedResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously stop the watch service
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return cancellable that may be used to cancel the request
*/
public Cancellable stopWatchServiceAsync(
StopWatchServiceRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::stopWatchService,
options,
AcknowledgedResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Put a watch into the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public PutWatchResponse putWatch(PutWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::putWatch,
options,
PutWatchResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously put a watch into the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable putWatchAsync(PutWatchRequest request, RequestOptions options, ActionListener listener) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::putWatch,
options,
PutWatchResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Gets a watch from the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public GetWatchResponse getWatch(GetWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::getWatch,
options,
GetWatchResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously gets a watch into the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable getWatchAsync(GetWatchRequest request, RequestOptions options, ActionListener listener) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::getWatch,
options,
GetWatchResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Deactivate an existing watch
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public DeactivateWatchResponse deactivateWatch(DeactivateWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::deactivateWatch,
options,
DeactivateWatchResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously deactivate an existing watch
* See
* the docs for more.
*
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable deactivateWatchAsync(
DeactivateWatchRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::deactivateWatch,
options,
DeactivateWatchResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Deletes a watch from the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public DeleteWatchResponse deleteWatch(DeleteWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::deleteWatch,
options,
DeleteWatchResponse::fromXContent,
singleton(404)
);
}
/**
* Asynchronously deletes a watch from the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable deleteWatchAsync(DeleteWatchRequest request, RequestOptions options, ActionListener listener) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::deleteWatch,
options,
DeleteWatchResponse::fromXContent,
listener,
singleton(404)
);
}
/**
* Acknowledges a watch.
* See
* the docs for more information.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException if there is a problem sending the request or parsing back the response
*/
public AckWatchResponse ackWatch(AckWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::ackWatch,
options,
AckWatchResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously acknowledges a watch.
* See
* the docs for more information.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon completion of the request
* @return cancellable that may be used to cancel the request
*/
public Cancellable ackWatchAsync(AckWatchRequest request, RequestOptions options, ActionListener listener) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::ackWatch,
options,
AckWatchResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Activate a watch from the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public ActivateWatchResponse activateWatch(ActivateWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::activateWatch,
options,
ActivateWatchResponse::fromXContent,
singleton(404)
);
}
/**
* Asynchronously activates a watch from the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable activateWatchAsync(
ActivateWatchRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::activateWatch,
options,
ActivateWatchResponse::fromXContent,
listener,
singleton(404)
);
}
/**
* Execute a watch on the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException if there is a problem sending the request or parsing the response
*/
public ExecuteWatchResponse executeWatch(ExecuteWatchRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::executeWatch,
options,
ExecuteWatchResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously execute a watch on the cluster
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notifed upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable executeWatchAsync(
ExecuteWatchRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::executeWatch,
options,
ExecuteWatchResponse::fromXContent,
listener,
emptySet()
);
}
/**
* Get the watcher stats
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @return the response
* @throws IOException in case there is a problem sending the request or parsing back the response
*/
public WatcherStatsResponse watcherStats(WatcherStatsRequest request, RequestOptions options) throws IOException {
return restHighLevelClient.performRequestAndParseEntity(
request,
WatcherRequestConverters::watcherStats,
options,
WatcherStatsResponse::fromXContent,
emptySet()
);
}
/**
* Asynchronously get the watcher stats
* See
* the docs for more.
* @param request the request
* @param options the request options (e.g. headers), use {@link RequestOptions#DEFAULT} if nothing needs to be customized
* @param listener the listener to be notified upon request completion
* @return cancellable that may be used to cancel the request
*/
public Cancellable watcherStatsAsync(
WatcherStatsRequest request,
RequestOptions options,
ActionListener listener
) {
return restHighLevelClient.performRequestAsyncAndParseEntity(
request,
WatcherRequestConverters::watcherStats,
options,
WatcherStatsResponse::fromXContent,
listener,
emptySet()
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy