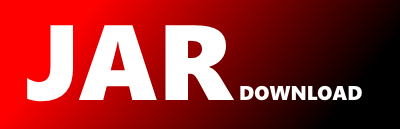
org.elasticsearch.client.security.GetUserPrivilegesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.security;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.security.user.privileges.ApplicationResourcePrivileges;
import org.elasticsearch.client.security.user.privileges.GlobalPrivileges;
import org.elasticsearch.client.security.user.privileges.UserIndicesPrivileges;
import org.elasticsearch.xcontent.ConstructingObjectParser;
import org.elasticsearch.xcontent.ParseField;
import org.elasticsearch.xcontent.XContentParser;
import java.io.IOException;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.Set;
import static org.elasticsearch.xcontent.ConstructingObjectParser.constructorArg;
/**
* The response for the {@link org.elasticsearch.client.SecurityClient#getUserPrivileges(RequestOptions)} API.
* See the API docs
*/
public class GetUserPrivilegesResponse {
private static final ConstructingObjectParser PARSER = new ConstructingObjectParser<>(
"get_user_privileges_response",
true,
GetUserPrivilegesResponse::buildResponseFromParserArgs
);
@SuppressWarnings("unchecked")
private static GetUserPrivilegesResponse buildResponseFromParserArgs(Object[] args) {
return new GetUserPrivilegesResponse(
(Collection) args[0],
(Collection) args[1],
(Collection) args[2],
(Collection) args[3],
(Collection) args[4]
);
}
static {
PARSER.declareStringArray(constructorArg(), new ParseField("cluster"));
PARSER.declareObjectArray(constructorArg(), (parser, ignore) -> GlobalPrivileges.fromXContent(parser), new ParseField("global"));
PARSER.declareObjectArray(
constructorArg(),
(parser, ignore) -> UserIndicesPrivileges.fromXContent(parser),
new ParseField("indices")
);
PARSER.declareObjectArray(
constructorArg(),
(parser, ignore) -> ApplicationResourcePrivileges.fromXContent(parser),
new ParseField("applications")
);
PARSER.declareStringArray(constructorArg(), new ParseField("run_as"));
}
public static GetUserPrivilegesResponse fromXContent(XContentParser parser) throws IOException {
return PARSER.parse(parser, null);
}
private Set clusterPrivileges;
private Set globalPrivileges;
private Set indicesPrivileges;
private Set applicationPrivileges;
private Set runAsPrivilege;
public GetUserPrivilegesResponse(
Collection clusterPrivileges,
Collection globalPrivileges,
Collection indicesPrivileges,
Collection applicationPrivileges,
Collection runAsPrivilege
) {
this.clusterPrivileges = Collections.unmodifiableSet(new LinkedHashSet<>(clusterPrivileges));
this.globalPrivileges = Collections.unmodifiableSet(new LinkedHashSet<>(globalPrivileges));
this.indicesPrivileges = Collections.unmodifiableSet(new LinkedHashSet<>(indicesPrivileges));
this.applicationPrivileges = Collections.unmodifiableSet(new LinkedHashSet<>(applicationPrivileges));
this.runAsPrivilege = Collections.unmodifiableSet(new LinkedHashSet<>(runAsPrivilege));
}
public Set getClusterPrivileges() {
return clusterPrivileges;
}
public Set getGlobalPrivileges() {
return globalPrivileges;
}
public Set getIndicesPrivileges() {
return indicesPrivileges;
}
public Set getApplicationPrivileges() {
return applicationPrivileges;
}
public Set getRunAsPrivilege() {
return runAsPrivilege;
}
@Override
public String toString() {
return "GetUserPrivilegesResponse{"
+ "clusterPrivileges="
+ clusterPrivileges
+ ", globalPrivileges="
+ globalPrivileges
+ ", indicesPrivileges="
+ indicesPrivileges
+ ", applicationPrivileges="
+ applicationPrivileges
+ ", runAsPrivilege="
+ runAsPrivilege
+ '}';
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final GetUserPrivilegesResponse that = (GetUserPrivilegesResponse) o;
return Objects.equals(this.clusterPrivileges, that.clusterPrivileges)
&& Objects.equals(this.globalPrivileges, that.globalPrivileges)
&& Objects.equals(this.indicesPrivileges, that.indicesPrivileges)
&& Objects.equals(this.applicationPrivileges, that.applicationPrivileges)
&& Objects.equals(this.runAsPrivilege, that.runAsPrivilege);
}
@Override
public int hashCode() {
return Objects.hash(clusterPrivileges, globalPrivileges, indicesPrivileges, applicationPrivileges, runAsPrivilege);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy