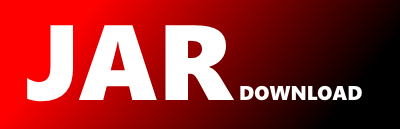
org.elasticsearch.client.transform.PreviewTransformResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.transform;
import org.elasticsearch.action.admin.indices.alias.Alias;
import org.elasticsearch.client.indices.CreateIndexRequest;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.xcontent.ConstructingObjectParser;
import org.elasticsearch.xcontent.ParseField;
import org.elasticsearch.xcontent.XContentParser;
import java.io.IOException;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import static org.elasticsearch.xcontent.ConstructingObjectParser.optionalConstructorArg;
public class PreviewTransformResponse {
public static class GeneratedDestIndexSettings {
static final ParseField MAPPINGS = new ParseField("mappings");
private static final ParseField SETTINGS = new ParseField("settings");
private static final ParseField ALIASES = new ParseField("aliases");
private final Map mappings;
private final Settings settings;
private final Set aliases;
private static final ConstructingObjectParser PARSER = new ConstructingObjectParser<>(
"transform_preview_generated_dest_index",
true,
args -> {
@SuppressWarnings("unchecked")
Map mappings = (Map) args[0];
Settings settings = (Settings) args[1];
@SuppressWarnings("unchecked")
Set aliases = (Set) args[2];
return new GeneratedDestIndexSettings(mappings, settings, aliases);
}
);
static {
PARSER.declareObject(optionalConstructorArg(), (p, c) -> p.mapOrdered(), MAPPINGS);
PARSER.declareObject(optionalConstructorArg(), (p, c) -> Settings.fromXContent(p), SETTINGS);
PARSER.declareObject(optionalConstructorArg(), (p, c) -> {
Set aliases = new HashSet<>();
while ((p.nextToken()) != XContentParser.Token.END_OBJECT) {
aliases.add(Alias.fromXContent(p));
}
return aliases;
}, ALIASES);
}
public GeneratedDestIndexSettings(Map mappings, Settings settings, Set aliases) {
this.mappings = mappings == null ? Collections.emptyMap() : Collections.unmodifiableMap(mappings);
this.settings = settings == null ? Settings.EMPTY : settings;
this.aliases = aliases == null ? Collections.emptySet() : Collections.unmodifiableSet(aliases);
}
public Map getMappings() {
return mappings;
}
public Settings getSettings() {
return settings;
}
public Set getAliases() {
return aliases;
}
public static GeneratedDestIndexSettings fromXContent(final XContentParser parser) {
return PARSER.apply(parser, null);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj == null || obj.getClass() != getClass()) {
return false;
}
GeneratedDestIndexSettings other = (GeneratedDestIndexSettings) obj;
return Objects.equals(other.mappings, mappings)
&& Objects.equals(other.settings, settings)
&& Objects.equals(other.aliases, aliases);
}
@Override
public int hashCode() {
return Objects.hash(mappings, settings, aliases);
}
}
public static final ParseField PREVIEW = new ParseField("preview");
public static final ParseField GENERATED_DEST_INDEX_SETTINGS = new ParseField("generated_dest_index");
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy