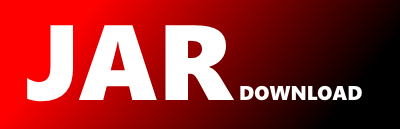
org.elasticsearch.client.indices.ReloadAnalyzersResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.indices;
import org.elasticsearch.client.core.BroadcastResponse;
import org.elasticsearch.core.Tuple;
import org.elasticsearch.xcontent.ConstructingObjectParser;
import org.elasticsearch.xcontent.ParseField;
import org.elasticsearch.xcontent.XContentParser;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import static org.elasticsearch.xcontent.ConstructingObjectParser.constructorArg;
/**
* The response object that will be returned when reloading analyzers
*/
public class ReloadAnalyzersResponse extends BroadcastResponse {
private final Map reloadDetails;
ReloadAnalyzersResponse(final Shards shards, Map reloadDetails) {
super(shards);
this.reloadDetails = reloadDetails;
}
@SuppressWarnings({ "unchecked" })
private static final ConstructingObjectParser PARSER = new ConstructingObjectParser<>(
"reload_analyzer",
true,
arg -> {
Shards shards = (Shards) arg[0];
List> results = (List>) arg[1];
Map reloadDetails = new HashMap<>();
for (Tuple result : results) {
reloadDetails.put(result.v1(), result.v2());
}
return new ReloadAnalyzersResponse(shards, reloadDetails);
}
);
@SuppressWarnings({ "unchecked" })
private static final ConstructingObjectParser, Void> ENTRY_PARSER = new ConstructingObjectParser<>(
"reload_analyzer.entry",
true,
arg -> {
String index = (String) arg[0];
Set nodeIds = new HashSet<>((List) arg[1]);
Set analyzers = new HashSet<>((List) arg[2]);
return new Tuple<>(index, new ReloadDetails(index, nodeIds, analyzers));
}
);
static {
declareShardsField(PARSER);
PARSER.declareObjectArray(constructorArg(), ENTRY_PARSER, new ParseField("reload_details"));
ENTRY_PARSER.declareString(constructorArg(), new ParseField("index"));
ENTRY_PARSER.declareStringArray(constructorArg(), new ParseField("reloaded_node_ids"));
ENTRY_PARSER.declareStringArray(constructorArg(), new ParseField("reloaded_analyzers"));
}
public static ReloadAnalyzersResponse fromXContent(XContentParser parser) {
return PARSER.apply(parser, null);
}
public Map getReloadedDetails() {
return reloadDetails;
}
public static class ReloadDetails {
private final String indexName;
private final Set reloadedIndicesNodes;
private final Set reloadedAnalyzers;
public ReloadDetails(String name, Set reloadedIndicesNodes, Set reloadedAnalyzers) {
this.indexName = name;
this.reloadedIndicesNodes = reloadedIndicesNodes;
this.reloadedAnalyzers = reloadedAnalyzers;
}
public String getIndexName() {
return indexName;
}
public Set getReloadedIndicesNodes() {
return reloadedIndicesNodes;
}
public Set getReloadedAnalyzers() {
return reloadedAnalyzers;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy