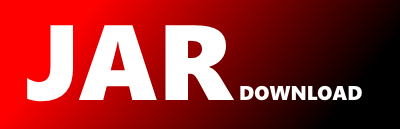
org.elasticsearch.client.security.user.privileges.UserIndicesPrivileges Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.security.user.privileges;
import org.elasticsearch.client.security.GetUserPrivilegesResponse;
import org.elasticsearch.xcontent.ConstructingObjectParser;
import org.elasticsearch.xcontent.XContentParser;
import java.io.IOException;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import static org.elasticsearch.xcontent.ConstructingObjectParser.constructorArg;
import static org.elasticsearch.xcontent.ConstructingObjectParser.optionalConstructorArg;
/**
* Represents an "index" privilege in the {@link GetUserPrivilegesResponse}. This differs from the
* {@link org.elasticsearch.client.security.user.privileges.IndicesPrivileges}" object in a
* {@link org.elasticsearch.client.security.user.privileges.Role}
* as it supports an array value for {@link #getFieldSecurity() field_security} and {@link #getQueries() query}.
* See the API docs
*/
public class UserIndicesPrivileges extends AbstractIndicesPrivileges {
private final Set fieldSecurity;
private final Set query;
private static final ConstructingObjectParser PARSER = new ConstructingObjectParser<>(
"user_indices_privilege",
true,
UserIndicesPrivileges::buildObjectFromParserArgs
);
static {
PARSER.declareStringArray(constructorArg(), IndicesPrivileges.NAMES);
PARSER.declareStringArray(constructorArg(), IndicesPrivileges.PRIVILEGES);
PARSER.declareBoolean(constructorArg(), IndicesPrivileges.ALLOW_RESTRICTED_INDICES);
PARSER.declareObjectArray(optionalConstructorArg(), IndicesPrivileges.FieldSecurity::parse, IndicesPrivileges.FIELD_PERMISSIONS);
PARSER.declareStringArray(optionalConstructorArg(), IndicesPrivileges.QUERY);
}
@SuppressWarnings("unchecked")
private static UserIndicesPrivileges buildObjectFromParserArgs(Object[] args) {
return new UserIndicesPrivileges(
(List) args[0],
(List) args[1],
(Boolean) args[2],
(List) args[3],
(List) args[4]
);
}
public static UserIndicesPrivileges fromXContent(XContentParser parser) throws IOException {
return PARSER.parse(parser, null);
}
public UserIndicesPrivileges(
Collection indices,
Collection privileges,
boolean allowRestrictedIndices,
Collection fieldSecurity,
Collection query
) {
super(indices, privileges, allowRestrictedIndices);
this.fieldSecurity = fieldSecurity == null ? Collections.emptySet() : Collections.unmodifiableSet(new HashSet<>(fieldSecurity));
this.query = query == null ? Collections.emptySet() : Collections.unmodifiableSet(new HashSet<>(query));
}
public Set getFieldSecurity() {
return fieldSecurity;
}
public Set getQueries() {
return query;
}
@Override
public boolean isUsingDocumentLevelSecurity() {
return query.isEmpty() == false;
}
@Override
public boolean isUsingFieldLevelSecurity() {
return fieldSecurity.stream().anyMatch(FieldSecurity::isUsingFieldLevelSecurity);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final UserIndicesPrivileges that = (UserIndicesPrivileges) o;
return Objects.equals(indices, that.indices)
&& Objects.equals(privileges, that.privileges)
&& allowRestrictedIndices == that.allowRestrictedIndices
&& Objects.equals(fieldSecurity, that.fieldSecurity)
&& Objects.equals(query, that.query);
}
@Override
public int hashCode() {
return Objects.hash(indices, privileges, allowRestrictedIndices, fieldSecurity, query);
}
@Override
public String toString() {
return "UserIndexPrivilege{"
+ "indices="
+ indices
+ ", privileges="
+ privileges
+ ", allow_restricted_indices="
+ allowRestrictedIndices
+ ", fieldSecurity="
+ fieldSecurity
+ ", query="
+ query
+ '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy