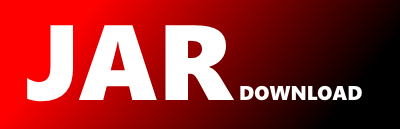
org.elasticsearch.client.tasks.ListTasksResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-rest-high-level-client Show documentation
Show all versions of elasticsearch-rest-high-level-client Show documentation
Elasticsearch subproject :client:rest-high-level
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.tasks;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
import static java.util.stream.Collectors.groupingBy;
import static java.util.stream.Collectors.toList;
public class ListTasksResponse {
protected final List taskFailures = new ArrayList<>();
protected final List nodeFailures = new ArrayList<>();
protected final List nodesInfoData = new ArrayList<>();
protected final List tasks = new ArrayList<>();
protected final List taskGroups = new ArrayList<>();
ListTasksResponse(List nodesInfoData, List taskFailures, List nodeFailures) {
if (taskFailures != null) {
this.taskFailures.addAll(taskFailures);
}
if (nodeFailures != null) {
this.nodeFailures.addAll(nodeFailures);
}
if (nodesInfoData != null) {
this.nodesInfoData.addAll(nodesInfoData);
}
this.tasks.addAll(this.nodesInfoData.stream().flatMap(nodeData -> nodeData.getTasks().stream()).collect(toList()));
this.taskGroups.addAll(buildTaskGroups());
}
private List buildTaskGroups() {
Map taskIdToBuilderMap = new HashMap<>();
List topLevelTasks = new ArrayList<>();
// First populate all tasks
for (TaskInfo taskInfo : this.tasks) {
taskIdToBuilderMap.put(taskInfo.getTaskId(), TaskGroup.builder(taskInfo));
}
// Now go through all task group builders and add children to their parents
for (TaskGroup.Builder taskGroup : taskIdToBuilderMap.values()) {
TaskId parentTaskId = taskGroup.getTaskInfo().getParentTaskId();
if (parentTaskId != null) {
TaskGroup.Builder parentTask = taskIdToBuilderMap.get(parentTaskId);
if (parentTask != null) {
// we found parent in the list of tasks - add it to the parent list
parentTask.addGroup(taskGroup);
} else {
// we got zombie or the parent was filtered out - add it to the top task list
topLevelTasks.add(taskGroup);
}
} else {
// top level task - add it to the top task list
topLevelTasks.add(taskGroup);
}
}
return Collections.unmodifiableList(topLevelTasks.stream().map(TaskGroup.Builder::build).collect(Collectors.toList()));
}
public List getTasks() {
return tasks;
}
public Map> getPerNodeTasks() {
return getTasks().stream().collect(groupingBy(TaskInfo::getNodeId));
}
public List getTaskFailures() {
return taskFailures;
}
public List getNodeFailures() {
return nodeFailures;
}
public List getTaskGroups() {
return taskGroups;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if ((o instanceof ListTasksResponse) == false) return false;
ListTasksResponse response = (ListTasksResponse) o;
return nodesInfoData.equals(response.nodesInfoData)
&& Objects.equals(getTaskFailures(), response.getTaskFailures())
&& Objects.equals(getNodeFailures(), response.getNodeFailures())
&& Objects.equals(getTasks(), response.getTasks())
&& Objects.equals(getTaskGroups(), response.getTaskGroups());
}
@Override
public int hashCode() {
return Objects.hash(nodesInfoData, getTaskFailures(), getNodeFailures(), getTasks(), getTaskGroups());
}
@Override
public String toString() {
return "CancelTasksResponse{"
+ "nodesInfoData="
+ nodesInfoData
+ ", taskFailures="
+ taskFailures
+ ", nodeFailures="
+ nodeFailures
+ ", tasks="
+ tasks
+ ", taskGroups="
+ taskGroups
+ '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy