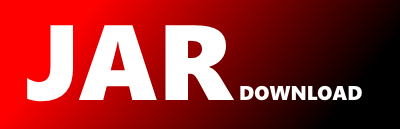
org.elasticsearch.xpack.esql.plan.logical.Aggregate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of x-pack-esql Show documentation
Show all versions of x-pack-esql Show documentation
The plugin that powers ESQL for Elasticsearch
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0; you may not use this file except in compliance with the Elastic License
* 2.0.
*/
package org.elasticsearch.xpack.esql.plan.logical;
import org.elasticsearch.TransportVersions;
import org.elasticsearch.common.io.stream.StreamInput;
import org.elasticsearch.common.io.stream.StreamOutput;
import org.elasticsearch.xpack.esql.core.capabilities.Resolvables;
import org.elasticsearch.xpack.esql.core.expression.Attribute;
import org.elasticsearch.xpack.esql.core.expression.Expression;
import org.elasticsearch.xpack.esql.core.expression.Expressions;
import org.elasticsearch.xpack.esql.core.expression.NamedExpression;
import org.elasticsearch.xpack.esql.core.plan.logical.LogicalPlan;
import org.elasticsearch.xpack.esql.core.plan.logical.UnaryPlan;
import org.elasticsearch.xpack.esql.core.tree.NodeInfo;
import org.elasticsearch.xpack.esql.core.tree.Source;
import org.elasticsearch.xpack.esql.io.stream.PlanStreamInput;
import org.elasticsearch.xpack.esql.io.stream.PlanStreamOutput;
import java.io.IOException;
import java.util.List;
import java.util.Objects;
public class Aggregate extends UnaryPlan {
public enum AggregateType {
STANDARD,
// include metrics aggregates such as rates
METRICS;
static void writeType(StreamOutput out, AggregateType type) throws IOException {
if (out.getTransportVersion().onOrAfter(TransportVersions.ESQL_ADD_AGGREGATE_TYPE)) {
out.writeString(type.name());
} else if (type != STANDARD) {
throw new IllegalStateException("cluster is not ready to support aggregate type [" + type + "]");
}
}
static AggregateType readType(StreamInput in) throws IOException {
if (in.getTransportVersion().onOrAfter(TransportVersions.ESQL_ADD_AGGREGATE_TYPE)) {
return AggregateType.valueOf(in.readString());
} else {
return STANDARD;
}
}
}
private final AggregateType aggregateType;
private final List groupings;
private final List extends NamedExpression> aggregates;
public Aggregate(
Source source,
LogicalPlan child,
AggregateType aggregateType,
List groupings,
List extends NamedExpression> aggregates
) {
super(source, child);
this.aggregateType = aggregateType;
this.groupings = groupings;
this.aggregates = aggregates;
}
public Aggregate(PlanStreamInput in) throws IOException {
this(
Source.readFrom(in),
in.readLogicalPlanNode(),
AggregateType.readType(in),
in.readNamedWriteableCollectionAsList(Expression.class),
in.readNamedWriteableCollectionAsList(NamedExpression.class)
);
}
public static void writeAggregate(PlanStreamOutput out, Aggregate aggregate) throws IOException {
Source.EMPTY.writeTo(out);
out.writeLogicalPlanNode(aggregate.child());
AggregateType.writeType(out, aggregate.aggregateType());
out.writeNamedWriteableCollection(aggregate.groupings);
out.writeNamedWriteableCollection(aggregate.aggregates());
}
@Override
protected NodeInfo info() {
return NodeInfo.create(this, Aggregate::new, child(), aggregateType, groupings, aggregates);
}
@Override
public Aggregate replaceChild(LogicalPlan newChild) {
return new Aggregate(source(), newChild, aggregateType, groupings, aggregates);
}
public AggregateType aggregateType() {
return aggregateType;
}
public List groupings() {
return groupings;
}
public List extends NamedExpression> aggregates() {
return aggregates;
}
@Override
public boolean expressionsResolved() {
return Resolvables.resolved(groupings) && Resolvables.resolved(aggregates);
}
@Override
public List output() {
return Expressions.asAttributes(aggregates);
}
@Override
public int hashCode() {
return Objects.hash(aggregateType, groupings, aggregates, child());
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Aggregate other = (Aggregate) obj;
return aggregateType == other.aggregateType
&& Objects.equals(groupings, other.groupings)
&& Objects.equals(aggregates, other.aggregates)
&& Objects.equals(child(), other.child());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy