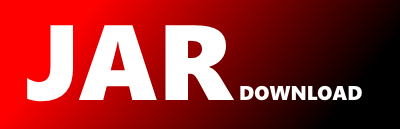
org.elasticsearch.action.support.ActionTestUtils Maven / Gradle / Ivy
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.action.support;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.ActionRequest;
import org.elasticsearch.action.ActionResponse;
import org.elasticsearch.client.Response;
import org.elasticsearch.client.ResponseListener;
import org.elasticsearch.core.CheckedConsumer;
import org.elasticsearch.tasks.Task;
import org.elasticsearch.tasks.TaskManager;
import org.elasticsearch.transport.Transport;
import static org.elasticsearch.action.support.PlainActionFuture.newFuture;
import static org.mockito.Mockito.mock;
public class ActionTestUtils {
private ActionTestUtils() { /* no construction */ }
public static Response executeBlocking(
TransportAction action,
Request request
) {
PlainActionFuture future = newFuture();
Task task = mock(Task.class);
action.execute(task, request, future);
return future.actionGet();
}
public static Response executeBlockingWithTask(
TaskManager taskManager,
Transport.Connection localConnection,
TransportAction action,
Request request
) {
PlainActionFuture future = newFuture();
taskManager.registerAndExecute(
"transport",
action,
request,
localConnection,
(t, r) -> future.onResponse(r),
(t, e) -> future.onFailure(e)
);
return future.actionGet();
}
/**
* Executes the given action.
*
* This is a shim method to make execution publicly available in tests.
*/
public static void execute(
TransportAction action,
Task task,
Request request,
ActionListener listener
) {
action.execute(task, request, listener);
}
public static ActionListener assertNoFailureListener(CheckedConsumer consumer) {
return ActionListener.wrap(consumer, e -> { throw new AssertionError(e); });
}
public static ResponseListener wrapAsRestResponseListener(ActionListener listener) {
return new ResponseListener() {
@Override
public void onSuccess(Response response) {
listener.onResponse(response);
}
@Override
public void onFailure(Exception exception) {
listener.onFailure(exception);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy