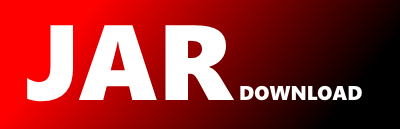
org.elasticsearch.indices.recovery.AsyncRecoveryTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of framework Show documentation
Show all versions of framework Show documentation
Elasticsearch subproject :test:framework
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.indices.recovery;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.common.bytes.ReleasableBytesReference;
import org.elasticsearch.index.seqno.ReplicationTracker;
import org.elasticsearch.index.seqno.RetentionLeases;
import org.elasticsearch.index.snapshots.blobstore.BlobStoreIndexShardSnapshot;
import org.elasticsearch.index.store.Store;
import org.elasticsearch.index.store.StoreFileMetadata;
import org.elasticsearch.index.translog.Translog;
import org.elasticsearch.repositories.IndexId;
import java.util.List;
import java.util.concurrent.Executor;
/**
* Wraps a {@link RecoveryTarget} to make all remote calls to be executed asynchronously using the provided {@code executor}.
*/
public class AsyncRecoveryTarget implements RecoveryTargetHandler {
private final RecoveryTargetHandler target;
private final Executor executor;
public AsyncRecoveryTarget(RecoveryTargetHandler target, Executor executor) {
this.executor = executor;
this.target = target;
}
@Override
public void prepareForTranslogOperations(int totalTranslogOps, ActionListener listener) {
executor.execute(() -> target.prepareForTranslogOperations(totalTranslogOps, listener));
}
@Override
public void finalizeRecovery(long globalCheckpoint, long trimAboveSeqNo, ActionListener listener) {
executor.execute(() -> target.finalizeRecovery(globalCheckpoint, trimAboveSeqNo, listener));
}
@Override
public void handoffPrimaryContext(ReplicationTracker.PrimaryContext primaryContext, ActionListener listener) {
executor.execute(() -> target.handoffPrimaryContext(primaryContext, listener));
}
@Override
public void indexTranslogOperations(
List operations,
int totalTranslogOps,
long maxSeenAutoIdTimestampOnPrimary,
long maxSeqNoOfDeletesOrUpdatesOnPrimary,
RetentionLeases retentionLeases,
long mappingVersionOnPrimary,
ActionListener listener
) {
executor.execute(
() -> target.indexTranslogOperations(
operations,
totalTranslogOps,
maxSeenAutoIdTimestampOnPrimary,
maxSeqNoOfDeletesOrUpdatesOnPrimary,
retentionLeases,
mappingVersionOnPrimary,
listener
)
);
}
@Override
public void receiveFileInfo(
List phase1FileNames,
List phase1FileSizes,
List phase1ExistingFileNames,
List phase1ExistingFileSizes,
int totalTranslogOps,
ActionListener listener
) {
executor.execute(
() -> target.receiveFileInfo(
phase1FileNames,
phase1FileSizes,
phase1ExistingFileNames,
phase1ExistingFileSizes,
totalTranslogOps,
listener
)
);
}
@Override
public void cleanFiles(
int totalTranslogOps,
long globalCheckpoint,
Store.MetadataSnapshot sourceMetadata,
ActionListener listener
) {
executor.execute(() -> target.cleanFiles(totalTranslogOps, globalCheckpoint, sourceMetadata, listener));
}
@Override
public void writeFileChunk(
StoreFileMetadata fileMetadata,
long position,
ReleasableBytesReference content,
boolean lastChunk,
int totalTranslogOps,
ActionListener listener
) {
final ReleasableBytesReference retained = content.retain();
final ActionListener wrappedListener = ActionListener.runBefore(listener, retained::close);
boolean success = false;
try {
executor.execute(() -> target.writeFileChunk(fileMetadata, position, retained, lastChunk, totalTranslogOps, wrappedListener));
success = true;
} finally {
if (success == false) {
content.decRef();
}
}
}
@Override
public void restoreFileFromSnapshot(
String repository,
IndexId indexId,
BlobStoreIndexShardSnapshot.FileInfo snapshotFile,
ActionListener listener
) {
executor.execute(() -> target.restoreFileFromSnapshot(repository, indexId, snapshotFile, listener));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy