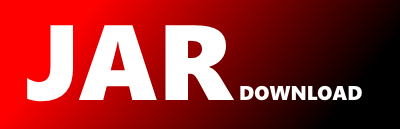
org.elasticsearch.test.hamcrest.ModuleDescriptorMatchers Maven / Gradle / Ivy
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.test.hamcrest;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
import java.lang.module.ModuleDescriptor.Exports;
import java.lang.module.ModuleDescriptor.Opens;
import java.lang.module.ModuleDescriptor.Provides;
import java.lang.module.ModuleDescriptor.Requires;
import java.util.List;
import java.util.Objects;
import java.util.Set;
public class ModuleDescriptorMatchers {
private ModuleDescriptorMatchers() {}
public static Matcher exportsOf(String pkg) {
return new ExportsMatcher(pkg, Set.of());
}
public static Matcher exportsOf(String pkg, Set targets) {
return new ExportsMatcher(pkg, targets);
}
public static Matcher opensOf(String pkg) {
return new OpensMatcher(pkg, Set.of());
}
public static Matcher opensOf(String pkg, Set targets) {
return new OpensMatcher(pkg, targets);
}
public static Matcher requiresOf(String mn) {
return new RequiresNameMatcher(mn);
}
public static Matcher providesOf(String service, List provides) {
return new ProvidesMatcher(service, provides);
}
/**
* Matcher that matches the source and targets of a {@code Requires}.
* The matcher is agnostic of the {@code Requires} modifiers.
*/
static class ExportsMatcher extends TypeSafeMatcher {
private final String source;
private final Set targets;
ExportsMatcher(String source, Set targets) {
this.source = source;
this.targets = Set.copyOf(targets);
}
@Override
protected boolean matchesSafely(final Exports item) {
return item != null && Objects.equals(item.source(), source) && Objects.equals(item.targets(), targets);
}
@Override
public void describeTo(final Description description) {
description.appendText(String.format(java.util.Locale.ROOT, "Exports[%s]", exportsToString(source, targets)));
}
@Override
protected void describeMismatchSafely(final Exports item, final Description mismatchDescription) {
describeTo(mismatchDescription);
if (item == null) {
mismatchDescription.appendText("was null");
} else {
mismatchDescription.appendText(String.format(java.util.Locale.ROOT, ", actual Exports[%s]", exportsToString(item)));
}
}
private static String exportsToString(String source, Set targets) {
if (targets.isEmpty()) {
return source;
} else {
return source + " to " + targets;
}
}
private static String exportsToString(Exports exports) {
if (exports.targets().isEmpty()) {
return exports.source();
} else {
return exports.source() + " to " + exports.targets();
}
}
}
/**
* Matcher that matches the name of a {@code Requires}.
* The matcher is agnostic of other {@code Requires} state, like the modifiers.
*/
static class RequiresNameMatcher extends TypeSafeMatcher {
private final String mn;
RequiresNameMatcher(String mn) {
this.mn = mn;
}
@Override
protected boolean matchesSafely(final Requires item) {
return item != null && Objects.equals(item.name(), mn);
}
@Override
public void describeTo(final Description description) {
description.appendText("Requires with name " + mn);
}
@Override
protected void describeMismatchSafely(final Requires item, final Description mismatchDescription) {
describeTo(mismatchDescription);
if (item == null) {
mismatchDescription.appendText("was null");
} else {
mismatchDescription.appendText(", actual Requires with name " + item);
}
}
}
/**
* Matcher that matches the source and targets of an {@code Opens}.
* The matcher is agnostic of the modifiers.
*/
static class OpensMatcher extends TypeSafeMatcher {
private final String source;
private final Set targets;
OpensMatcher(String source, Set targets) {
this.source = source;
this.targets = Set.copyOf(targets);
}
@Override
protected boolean matchesSafely(final Opens item) {
return item != null && Objects.equals(item.source(), source) && Objects.equals(item.targets(), targets);
}
@Override
public void describeTo(final Description description) {
description.appendText(String.format(java.util.Locale.ROOT, "Opens[%s]", opensToString(source, targets)));
}
@Override
protected void describeMismatchSafely(final Opens item, final Description mismatchDescription) {
describeTo(mismatchDescription);
if (item == null) {
mismatchDescription.appendText("was null");
} else {
mismatchDescription.appendText(String.format(java.util.Locale.ROOT, ", actual Opens[%s]", opensToString(item)));
}
}
private static String opensToString(String source, Set targets) {
if (targets.isEmpty()) {
return source;
} else {
return source + " to " + targets;
}
}
private static String opensToString(Opens opens) {
if (opens.targets().isEmpty()) {
return opens.source();
} else {
return opens.source() + " to " + opens.targets();
}
}
}
/**
* Matcher that matches the service and providers of a {@code Provides}.
*/
static class ProvidesMatcher extends TypeSafeMatcher {
private final String service;
private final List providers;
ProvidesMatcher(String service, List providers) {
this.service = service;
this.providers = List.copyOf(providers);
}
@Override
protected boolean matchesSafely(final Provides item) {
return item != null && item.service().equals(service) && item.providers().equals(providers);
}
@Override
public void describeTo(final Description description) {
description.appendText(String.format(java.util.Locale.ROOT, "Provides[%s]", providesToString(service, providers)));
}
@Override
protected void describeMismatchSafely(final Provides item, final Description mismatchDescription) {
describeTo(mismatchDescription);
if (item == null) {
mismatchDescription.appendText("was null");
} else {
mismatchDescription.appendText(String.format(java.util.Locale.ROOT, ", actual Provides[%s]", item));
}
}
private static String providesToString(String service, List provides) {
return service + " with " + provides;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy