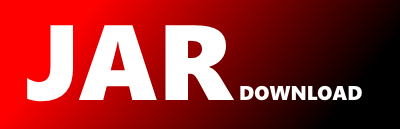
org.elasticsearch.cluster.metadata.MetaData Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.elasticsearch.cluster.metadata;
import com.carrotsearch.hppc.ObjectArrayList;
import com.carrotsearch.hppc.ObjectOpenHashSet;
import com.carrotsearch.hppc.cursors.ObjectCursor;
import com.carrotsearch.hppc.cursors.ObjectObjectCursor;
import com.google.common.base.Predicate;
import com.google.common.collect.*;
import org.elasticsearch.ElasticsearchIllegalArgumentException;
import org.elasticsearch.action.support.IndicesOptions;
import org.elasticsearch.cluster.block.ClusterBlock;
import org.elasticsearch.cluster.block.ClusterBlockLevel;
import org.elasticsearch.common.Nullable;
import org.elasticsearch.common.Strings;
import org.elasticsearch.common.collect.HppcMaps;
import org.elasticsearch.common.collect.ImmutableOpenMap;
import org.elasticsearch.common.io.stream.StreamInput;
import org.elasticsearch.common.io.stream.StreamOutput;
import org.elasticsearch.common.regex.Regex;
import org.elasticsearch.common.settings.ImmutableSettings;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.settings.SettingsFilter;
import org.elasticsearch.common.settings.loader.SettingsLoader;
import org.elasticsearch.common.xcontent.*;
import org.elasticsearch.index.Index;
import org.elasticsearch.indices.IndexClosedException;
import org.elasticsearch.indices.IndexMissingException;
import org.elasticsearch.rest.RestStatus;
import org.elasticsearch.search.warmer.IndexWarmersMetaData;
import java.io.IOException;
import java.util.*;
import static com.google.common.collect.Lists.newArrayList;
import static com.google.common.collect.Maps.newHashMap;
import static org.elasticsearch.common.settings.ImmutableSettings.*;
/**
*
*/
public class MetaData implements Iterable {
public static final String ALL = "_all";
public enum XContentContext {
/* Custom metadata should be returns as part of API call */
API,
/* Custom metadata should be stored as part of the persistent cluster state */
GATEWAY,
/* Custom metadata should be stored as part of a snapshot */
SNAPSHOT;
}
public static EnumSet API_ONLY = EnumSet.of(XContentContext.API);
public static EnumSet API_AND_GATEWAY = EnumSet.of(XContentContext.API, XContentContext.GATEWAY);
public static EnumSet API_AND_SNAPSHOT = EnumSet.of(XContentContext.API, XContentContext.SNAPSHOT);
public interface Custom {
abstract class Factory {
public abstract String type();
public abstract T readFrom(StreamInput in) throws IOException;
public abstract void writeTo(T customIndexMetaData, StreamOutput out) throws IOException;
public abstract T fromXContent(XContentParser parser) throws IOException;
public abstract void toXContent(T customIndexMetaData, XContentBuilder builder, ToXContent.Params params) throws IOException;
public EnumSet context() {
return API_ONLY;
}
}
/**
* The factory should implement this interface in order to filter out some of the settings from appearing in get cluster state request
*/
interface ToXFilteredContent {
void toXContent(T customIndexMetaData, XContentBuilder builder, ToXContent.Params params, SettingsFilter settingsFilter) throws IOException;
}
}
public static Map customFactories = new HashMap<>();
static {
// register non plugin custom metadata
registerFactory(RepositoriesMetaData.TYPE, RepositoriesMetaData.FACTORY);
registerFactory(SnapshotMetaData.TYPE, SnapshotMetaData.FACTORY);
registerFactory(RestoreMetaData.TYPE, RestoreMetaData.FACTORY);
}
/**
* Register a custom index meta data factory. Make sure to call it from a static block.
*/
public static void registerFactory(String type, Custom.Factory factory) {
customFactories.put(type, factory);
}
@Nullable
public static Custom.Factory lookupFactory(String type) {
return customFactories.get(type);
}
public static Custom.Factory lookupFactorySafe(String type) throws ElasticsearchIllegalArgumentException {
Custom.Factory factory = customFactories.get(type);
if (factory == null) {
throw new ElasticsearchIllegalArgumentException("No custom index metadata factory registered for type [" + type + "]");
}
return factory;
}
public static final String SETTING_READ_ONLY = "cluster.blocks.read_only";
public static final ClusterBlock CLUSTER_READ_ONLY_BLOCK = new ClusterBlock(6, "cluster read-only (api)", false, false, RestStatus.FORBIDDEN, EnumSet.of(ClusterBlockLevel.WRITE, ClusterBlockLevel.METADATA_WRITE));
public static final MetaData EMPTY_META_DATA = builder().build();
public static final String CONTEXT_MODE_PARAM = "context_mode";
public static final String CONTEXT_MODE_SNAPSHOT = XContentContext.SNAPSHOT.toString();
public static final String CONTEXT_MODE_GATEWAY = XContentContext.GATEWAY.toString();
private final String uuid;
private final long version;
private final Settings transientSettings;
private final Settings persistentSettings;
private final Settings settings;
private final ImmutableOpenMap indices;
private final ImmutableOpenMap templates;
private final ImmutableOpenMap customs;
private final transient int totalNumberOfShards; // Transient ? not serializable anyway?
private final int numberOfShards;
private final String[] allIndices;
private final String[] allOpenIndices;
private final String[] allClosedIndices;
private final ImmutableOpenMap> aliases;
private final ImmutableOpenMap aliasAndIndexToIndexMap;
@SuppressWarnings("unchecked")
MetaData(String uuid, long version, Settings transientSettings, Settings persistentSettings, ImmutableOpenMap indices, ImmutableOpenMap templates, ImmutableOpenMap customs) {
this.uuid = uuid;
this.version = version;
this.transientSettings = transientSettings;
this.persistentSettings = persistentSettings;
this.settings = ImmutableSettings.settingsBuilder().put(persistentSettings).put(transientSettings).build();
this.indices = indices;
this.customs = customs;
this.templates = templates;
int totalNumberOfShards = 0;
int numberOfShards = 0;
int numAliases = 0;
for (ObjectCursor cursor : indices.values()) {
totalNumberOfShards += cursor.value.totalNumberOfShards();
numberOfShards += cursor.value.numberOfShards();
numAliases += cursor.value.aliases().size();
}
this.totalNumberOfShards = totalNumberOfShards;
this.numberOfShards = numberOfShards;
// build all indices map
List allIndicesLst = Lists.newArrayList();
for (ObjectCursor cursor : indices.values()) {
allIndicesLst.add(cursor.value.index());
}
allIndices = allIndicesLst.toArray(new String[allIndicesLst.size()]);
int numIndices = allIndicesLst.size();
List allOpenIndices = Lists.newArrayList();
List allClosedIndices = Lists.newArrayList();
for (ObjectCursor cursor : indices.values()) {
IndexMetaData indexMetaData = cursor.value;
if (indexMetaData.state() == IndexMetaData.State.OPEN) {
allOpenIndices.add(indexMetaData.index());
} else if (indexMetaData.state() == IndexMetaData.State.CLOSE) {
allClosedIndices.add(indexMetaData.index());
}
}
this.allOpenIndices = allOpenIndices.toArray(new String[allOpenIndices.size()]);
this.allClosedIndices = allClosedIndices.toArray(new String[allClosedIndices.size()]);
// build aliases map
ImmutableOpenMap.Builder tmpAliases = ImmutableOpenMap.builder(numAliases);
for (ObjectCursor cursor : indices.values()) {
IndexMetaData indexMetaData = cursor.value;
String index = indexMetaData.index();
for (ObjectCursor aliasCursor : indexMetaData.aliases().values()) {
AliasMetaData aliasMd = aliasCursor.value;
ImmutableOpenMap.Builder indexAliasMap = (ImmutableOpenMap.Builder) tmpAliases.get(aliasMd.alias());
if (indexAliasMap == null) {
indexAliasMap = ImmutableOpenMap.builder(1); // typically, there is 1 alias pointing to an index
tmpAliases.put(aliasMd.alias(), indexAliasMap);
}
indexAliasMap.put(index, aliasMd);
}
}
for (ObjectCursor cursor : tmpAliases.keys()) {
String alias = cursor.value;
// if there is access to the raw values buffer of the map that the immutable maps wraps, then we don't need to use put, and just set array slots
ImmutableOpenMap map = ((ImmutableOpenMap.Builder) tmpAliases.get(alias)).cast().build();
tmpAliases.put(alias, map);
}
this.aliases = tmpAliases.>cast().build();
ImmutableOpenMap.Builder aliasAndIndexToIndexMap = ImmutableOpenMap.builder(numAliases + numIndices);
for (ObjectCursor cursor : indices.values()) {
IndexMetaData indexMetaData = cursor.value;
ObjectArrayList indicesLst = (ObjectArrayList) aliasAndIndexToIndexMap.get(indexMetaData.index());
if (indicesLst == null) {
indicesLst = new ObjectArrayList<>();
aliasAndIndexToIndexMap.put(indexMetaData.index(), indicesLst);
}
indicesLst.add(indexMetaData.index());
for (ObjectCursor cursor1 : indexMetaData.aliases().keys()) {
String alias = cursor1.value;
indicesLst = (ObjectArrayList) aliasAndIndexToIndexMap.get(alias);
if (indicesLst == null) {
indicesLst = new ObjectArrayList<>();
aliasAndIndexToIndexMap.put(alias, indicesLst);
}
indicesLst.add(indexMetaData.index());
}
}
for (ObjectObjectCursor cursor : aliasAndIndexToIndexMap) {
String[] indicesLst = ((ObjectArrayList) cursor.value).toArray(String.class);
aliasAndIndexToIndexMap.put(cursor.key, indicesLst);
}
this.aliasAndIndexToIndexMap = aliasAndIndexToIndexMap.cast().build();
}
public long version() {
return this.version;
}
public String uuid() {
return this.uuid;
}
/**
* Returns the merges transient and persistent settings.
*/
public Settings settings() {
return this.settings;
}
public Settings transientSettings() {
return this.transientSettings;
}
public Settings persistentSettings() {
return this.persistentSettings;
}
public ImmutableOpenMap> aliases() {
return this.aliases;
}
public ImmutableOpenMap> getAliases() {
return aliases();
}
/**
* Finds the specific index aliases that match with the specified aliases directly or partially via wildcards and
* that point to the specified concrete indices or match partially with the indices via wildcards.
*
* @param aliases The names of the index aliases to find
* @param concreteIndices The concrete indexes the index aliases must point to order to be returned.
* @return the found index aliases grouped by index
*/
public ImmutableOpenMap> findAliases(final String[] aliases, String[] concreteIndices) {
assert aliases != null;
assert concreteIndices != null;
if (concreteIndices.length == 0) {
return ImmutableOpenMap.of();
}
boolean matchAllAliases = matchAllAliases(aliases);
ImmutableOpenMap.Builder> mapBuilder = ImmutableOpenMap.builder();
Iterable intersection = HppcMaps.intersection(ObjectOpenHashSet.from(concreteIndices), indices.keys());
for (String index : intersection) {
IndexMetaData indexMetaData = indices.get(index);
List filteredValues = Lists.newArrayList();
for (ObjectCursor cursor : indexMetaData.getAliases().values()) {
AliasMetaData value = cursor.value;
if (matchAllAliases || Regex.simpleMatch(aliases, value.alias())) {
filteredValues.add(value);
}
}
if (!filteredValues.isEmpty()) {
mapBuilder.put(index, ImmutableList.copyOf(filteredValues));
}
}
return mapBuilder.build();
}
private static boolean matchAllAliases(final String[] aliases) {
for (String alias : aliases) {
if (alias.equals(ALL)) {
return true;
}
}
return aliases.length == 0;
}
/**
* Checks if at least one of the specified aliases exists in the specified concrete indices. Wildcards are supported in the
* alias names for partial matches.
*
* @param aliases The names of the index aliases to find
* @param concreteIndices The concrete indexes the index aliases must point to order to be returned.
* @return whether at least one of the specified aliases exists in one of the specified concrete indices.
*/
public boolean hasAliases(final String[] aliases, String[] concreteIndices) {
assert aliases != null;
assert concreteIndices != null;
if (concreteIndices.length == 0) {
return false;
}
Iterable intersection = HppcMaps.intersection(ObjectOpenHashSet.from(concreteIndices), indices.keys());
for (String index : intersection) {
IndexMetaData indexMetaData = indices.get(index);
List filteredValues = Lists.newArrayList();
for (ObjectCursor cursor : indexMetaData.getAliases().values()) {
AliasMetaData value = cursor.value;
if (Regex.simpleMatch(aliases, value.alias())) {
filteredValues.add(value);
}
}
if (!filteredValues.isEmpty()) {
return true;
}
}
return false;
}
/*
* Finds all mappings for types and concrete indices. Types are expanded to
* include all types that match the glob patterns in the types array. Empty
* types array, null or {"_all"} will be expanded to all types available for
* the given indices.
*/
public ImmutableOpenMap> findMappings(String[] concreteIndices, final String[] types) {
assert types != null;
assert concreteIndices != null;
if (concreteIndices.length == 0) {
return ImmutableOpenMap.of();
}
ImmutableOpenMap.Builder> indexMapBuilder = ImmutableOpenMap.builder();
Iterable intersection = HppcMaps.intersection(ObjectOpenHashSet.from(concreteIndices), indices.keys());
for (String index : intersection) {
IndexMetaData indexMetaData = indices.get(index);
ImmutableOpenMap.Builder filteredMappings;
if (isAllTypes(types)) {
indexMapBuilder.put(index, indexMetaData.getMappings()); // No types specified means get it all
} else {
filteredMappings = ImmutableOpenMap.builder();
for (ObjectObjectCursor cursor : indexMetaData.mappings()) {
if (Regex.simpleMatch(types, cursor.key)) {
filteredMappings.put(cursor.key, cursor.value);
}
}
if (!filteredMappings.isEmpty()) {
indexMapBuilder.put(index, filteredMappings.build());
}
}
}
return indexMapBuilder.build();
}
public ImmutableOpenMap> findWarmers(String[] concreteIndices, final String[] types, final String[] uncheckedWarmers) {
assert uncheckedWarmers != null;
assert concreteIndices != null;
if (concreteIndices.length == 0) {
return ImmutableOpenMap.of();
}
// special _all check to behave the same like not specifying anything for the warmers (not for the indices)
final String[] warmers = Strings.isAllOrWildcard(uncheckedWarmers) ? Strings.EMPTY_ARRAY : uncheckedWarmers;
ImmutableOpenMap.Builder> mapBuilder = ImmutableOpenMap.builder();
Iterable intersection = HppcMaps.intersection(ObjectOpenHashSet.from(concreteIndices), indices.keys());
for (String index : intersection) {
IndexMetaData indexMetaData = indices.get(index);
IndexWarmersMetaData indexWarmersMetaData = indexMetaData.custom(IndexWarmersMetaData.TYPE);
if (indexWarmersMetaData == null || indexWarmersMetaData.entries().isEmpty()) {
continue;
}
Collection filteredWarmers = Collections2.filter(indexWarmersMetaData.entries(), new Predicate() {
@Override
public boolean apply(IndexWarmersMetaData.Entry warmer) {
if (warmers.length != 0 && types.length != 0) {
return Regex.simpleMatch(warmers, warmer.name()) && Regex.simpleMatch(types, warmer.types());
} else if (warmers.length != 0) {
return Regex.simpleMatch(warmers, warmer.name());
} else if (types.length != 0) {
return Regex.simpleMatch(types, warmer.types());
} else {
return true;
}
}
});
if (!filteredWarmers.isEmpty()) {
mapBuilder.put(index, ImmutableList.copyOf(filteredWarmers));
}
}
return mapBuilder.build();
}
/**
* Returns all the concrete indices.
*/
public String[] concreteAllIndices() {
return allIndices;
}
public String[] getConcreteAllIndices() {
return concreteAllIndices();
}
public String[] concreteAllOpenIndices() {
return allOpenIndices;
}
public String[] getConcreteAllOpenIndices() {
return allOpenIndices;
}
public String[] concreteAllClosedIndices() {
return allClosedIndices;
}
public String[] getConcreteAllClosedIndices() {
return allClosedIndices;
}
/**
* Returns indexing routing for the given index.
*/
public String resolveIndexRouting(@Nullable String routing, String aliasOrIndex) {
// Check if index is specified by an alias
ImmutableOpenMap indexAliases = aliases.get(aliasOrIndex);
if (indexAliases == null || indexAliases.isEmpty()) {
return routing;
}
if (indexAliases.size() > 1) {
throw new ElasticsearchIllegalArgumentException("Alias [" + aliasOrIndex + "] has more than one index associated with it [" + Arrays.toString(indexAliases.keys().toArray(String.class)) + "], can't execute a single index op");
}
AliasMetaData aliasMd = indexAliases.values().iterator().next().value;
if (aliasMd.indexRouting() != null) {
if (routing != null) {
if (!routing.equals(aliasMd.indexRouting())) {
throw new ElasticsearchIllegalArgumentException("Alias [" + aliasOrIndex + "] has index routing associated with it [" + aliasMd.indexRouting() + "], and was provided with routing value [" + routing + "], rejecting operation");
}
}
routing = aliasMd.indexRouting();
}
if (routing != null) {
if (routing.indexOf(',') != -1) {
throw new ElasticsearchIllegalArgumentException("index/alias [" + aliasOrIndex + "] provided with routing value [" + routing + "] that resolved to several routing values, rejecting operation");
}
}
return routing;
}
public Map> resolveSearchRouting(@Nullable String routing, String aliasOrIndex) {
return resolveSearchRouting(routing, convertFromWildcards(new String[]{aliasOrIndex}, IndicesOptions.lenientExpandOpen()));
}
public Map> resolveSearchRouting(@Nullable String routing, String[] aliasesOrIndices) {
if (isAllIndices(aliasesOrIndices)) {
return resolveSearchRoutingAllIndices(routing);
}
aliasesOrIndices = convertFromWildcards(aliasesOrIndices, IndicesOptions.lenientExpandOpen());
if (aliasesOrIndices.length == 1) {
return resolveSearchRoutingSingleValue(routing, aliasesOrIndices[0]);
}
Map> routings = null;
Set paramRouting = null;
// List of indices that don't require any routing
Set norouting = new HashSet<>();
if (routing != null) {
paramRouting = Strings.splitStringByCommaToSet(routing);
}
for (String aliasOrIndex : aliasesOrIndices) {
ImmutableOpenMap indexToRoutingMap = aliases.get(aliasOrIndex);
if (indexToRoutingMap != null && !indexToRoutingMap.isEmpty()) {
for (ObjectObjectCursor indexRouting : indexToRoutingMap) {
if (!norouting.contains(indexRouting.key)) {
if (!indexRouting.value.searchRoutingValues().isEmpty()) {
// Routing alias
if (routings == null) {
routings = newHashMap();
}
Set r = routings.get(indexRouting.key);
if (r == null) {
r = new HashSet<>();
routings.put(indexRouting.key, r);
}
r.addAll(indexRouting.value.searchRoutingValues());
if (paramRouting != null) {
r.retainAll(paramRouting);
}
if (r.isEmpty()) {
routings.remove(indexRouting.key);
}
} else {
// Non-routing alias
if (!norouting.contains(indexRouting.key)) {
norouting.add(indexRouting.key);
if (paramRouting != null) {
Set r = new HashSet<>(paramRouting);
if (routings == null) {
routings = newHashMap();
}
routings.put(indexRouting.key, r);
} else {
if (routings != null) {
routings.remove(indexRouting.key);
}
}
}
}
}
}
} else {
// Index
if (!norouting.contains(aliasOrIndex)) {
norouting.add(aliasOrIndex);
if (paramRouting != null) {
Set r = new HashSet<>(paramRouting);
if (routings == null) {
routings = newHashMap();
}
routings.put(aliasOrIndex, r);
} else {
if (routings != null) {
routings.remove(aliasOrIndex);
}
}
}
}
}
if (routings == null || routings.isEmpty()) {
return null;
}
return routings;
}
private Map> resolveSearchRoutingSingleValue(@Nullable String routing, String aliasOrIndex) {
Map> routings = null;
Set paramRouting = null;
if (routing != null) {
paramRouting = Strings.splitStringByCommaToSet(routing);
}
ImmutableOpenMap indexToRoutingMap = aliases.get(aliasOrIndex);
if (indexToRoutingMap != null && !indexToRoutingMap.isEmpty()) {
// It's an alias
for (ObjectObjectCursor indexRouting : indexToRoutingMap) {
if (!indexRouting.value.searchRoutingValues().isEmpty()) {
// Routing alias
Set r = new HashSet<>(indexRouting.value.searchRoutingValues());
if (paramRouting != null) {
r.retainAll(paramRouting);
}
if (!r.isEmpty()) {
if (routings == null) {
routings = newHashMap();
}
routings.put(indexRouting.key, r);
}
} else {
// Non-routing alias
if (paramRouting != null) {
Set r = new HashSet<>(paramRouting);
if (routings == null) {
routings = newHashMap();
}
routings.put(indexRouting.key, r);
}
}
}
} else {
// It's an index
if (paramRouting != null) {
routings = ImmutableMap.of(aliasOrIndex, paramRouting);
}
}
return routings;
}
/**
* Sets the same routing for all indices
*/
private Map> resolveSearchRoutingAllIndices(String routing) {
if (routing != null) {
Set r = Strings.splitStringByCommaToSet(routing);
Map> routings = newHashMap();
String[] concreteIndices = concreteAllIndices();
for (String index : concreteIndices) {
routings.put(index, r);
}
return routings;
}
return null;
}
/**
* Translates the provided indices or aliases, eventually containing wildcard expressions, into actual indices.
* @deprecated use {@link #concreteIndices(org.elasticsearch.action.support.IndicesOptions, String...)} instead and
* be explicit about indices options to be used for indices resolution
*/
@Deprecated
public String[] concreteIndices(String[] indices) throws IndexMissingException {
return concreteIndices(IndicesOptions.strictExpand(), indices);
}
/**
* Translates the provided indices or aliases, eventually containing wildcard expressions, into actual indices.
*
* @param indicesOptions how the aliases or indices need to be resolved to concrete indices
* @param aliasesOrIndices the aliases or indices to be resolved to concrete indices
* @return the obtained concrete indices
* @throws IndexMissingException if one of the aliases or indices is missing and the provided indices options
* don't allow such a case, or if the final result of the indices resolution is no indices and the indices options
* don't allow such a case.
* @throws ElasticsearchIllegalArgumentException if one of the aliases resolve to multiple indices and the provided
* indices options don't allow such a case.
*/
public String[] concreteIndices(IndicesOptions indicesOptions, String... aliasesOrIndices) throws IndexMissingException, ElasticsearchIllegalArgumentException {
if (indicesOptions.expandWildcardsOpen() || indicesOptions.expandWildcardsClosed()) {
if (isAllIndices(aliasesOrIndices)) {
String[] concreteIndices;
if (indicesOptions.expandWildcardsOpen() && indicesOptions.expandWildcardsClosed()) {
concreteIndices = concreteAllIndices();
} else if (indicesOptions.expandWildcardsOpen()) {
concreteIndices = concreteAllOpenIndices();
} else {
concreteIndices = concreteAllClosedIndices();
}
if (!indicesOptions.allowNoIndices() && concreteIndices.length == 0) {
throw new IndexMissingException(new Index("_all"));
}
return concreteIndices;
}
aliasesOrIndices = convertFromWildcards(aliasesOrIndices, indicesOptions);
}
if (aliasesOrIndices == null || aliasesOrIndices.length == 0) {
if (!indicesOptions.allowNoIndices()) {
throw new ElasticsearchIllegalArgumentException("no indices were specified and wildcard expansion is disabled.");
} else {
return Strings.EMPTY_ARRAY;
}
}
boolean failClosed = indicesOptions.forbidClosedIndices() && !indicesOptions.ignoreUnavailable();
// optimize for single element index (common case)
if (aliasesOrIndices.length == 1) {
return concreteIndices(aliasesOrIndices[0], indicesOptions, !indicesOptions.allowNoIndices());
}
// check if its a possible aliased index, if not, just return the passed array
boolean possiblyAliased = false;
boolean closedIndices = false;
for (String index : aliasesOrIndices) {
IndexMetaData indexMetaData = indices.get(index);
if (indexMetaData == null) {
possiblyAliased = true;
break;
} else {
if (indicesOptions.forbidClosedIndices() && indexMetaData.getState() == IndexMetaData.State.CLOSE) {
if (failClosed) {
throw new IndexClosedException(new Index(index));
} else {
closedIndices = true;
}
}
}
}
if (!possiblyAliased) {
if (closedIndices) {
Set actualIndices = new HashSet<>(Arrays.asList(aliasesOrIndices));
actualIndices.retainAll(new HashSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy