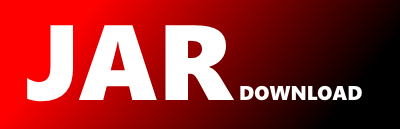
org.elasticsearch.transport.RequestHandlerRegistry Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.elasticsearch.transport;
import org.elasticsearch.common.io.stream.StreamInput;
import org.elasticsearch.common.io.stream.Writeable;
import org.elasticsearch.common.lease.Releasable;
import org.elasticsearch.common.lease.Releasables;
import org.elasticsearch.tasks.CancellableTask;
import org.elasticsearch.tasks.Task;
import org.elasticsearch.tasks.TaskManager;
import java.io.IOException;
public class RequestHandlerRegistry {
private final String action;
private final TransportRequestHandler handler;
private final boolean forceExecution;
private final boolean canTripCircuitBreaker;
private final String executor;
private final TaskManager taskManager;
private final Writeable.Reader requestReader;
public RequestHandlerRegistry(String action, Writeable.Reader requestReader, TaskManager taskManager,
TransportRequestHandler handler, String executor, boolean forceExecution,
boolean canTripCircuitBreaker) {
this.action = action;
this.requestReader = requestReader;
this.handler = handler;
this.forceExecution = forceExecution;
this.canTripCircuitBreaker = canTripCircuitBreaker;
this.executor = executor;
this.taskManager = taskManager;
}
public String getAction() {
return action;
}
public Request newRequest(StreamInput in) throws IOException {
return requestReader.read(in);
}
public void processMessageReceived(Request request, TransportChannel channel) throws Exception {
final Task task = taskManager.register(channel.getChannelType(), action, request);
Releasable unregisterTask = () -> taskManager.unregister(task);
try {
if (channel instanceof TcpTransportChannel && task instanceof CancellableTask) {
final TcpChannel tcpChannel = ((TcpTransportChannel) channel).getChannel();
final Releasable stopTracking = taskManager.startTrackingCancellableChannelTask(tcpChannel, (CancellableTask) task);
unregisterTask = Releasables.wrap(unregisterTask, stopTracking);
}
final TaskTransportChannel taskTransportChannel = new TaskTransportChannel(channel, unregisterTask);
handler.messageReceived(request, taskTransportChannel, task);
unregisterTask = null;
} finally {
Releasables.close(unregisterTask);
}
}
public boolean isForceExecution() {
return forceExecution;
}
public boolean canTripCircuitBreaker() {
return canTripCircuitBreaker;
}
public String getExecutor() {
return executor;
}
public TransportRequestHandler getHandler() {
return handler;
}
@Override
public String toString() {
return handler.toString();
}
public static RequestHandlerRegistry replaceHandler(RequestHandlerRegistry registry,
TransportRequestHandler handler) {
return new RequestHandlerRegistry<>(registry.action, registry.requestReader, registry.taskManager, handler,
registry.executor, registry.forceExecution, registry.canTripCircuitBreaker);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy