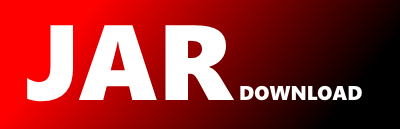
org.elasticsearch.index.mapper.SourceValueFetcher Maven / Gradle / Ivy
Show all versions of elasticsearch Show documentation
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.mapper;
import org.elasticsearch.core.Nullable;
import org.elasticsearch.index.query.SearchExecutionContext;
import org.elasticsearch.search.lookup.SourceLookup;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.List;
import java.util.Queue;
import java.util.Set;
/**
* An implementation of {@link ValueFetcher} that knows how to extract values
* from the document source. Most standard field mappers will use this class
* to implement value fetching.
*
* Field types that handle arrays directly should instead use {@link ArraySourceValueFetcher}.
*/
public abstract class SourceValueFetcher implements ValueFetcher {
private final Set sourcePaths;
private final @Nullable Object nullValue;
public SourceValueFetcher(String fieldName, SearchExecutionContext context) {
this(fieldName, context, null);
}
/**
* @param fieldName The name of the field.
* @param context The query shard context
* @param nullValue An optional substitute value if the _source value is 'null'.
*/
public SourceValueFetcher(String fieldName, SearchExecutionContext context, Object nullValue) {
this(context.sourcePath(fieldName), nullValue);
}
/**
* @param sourcePaths The paths to pull source values from
* @param nullValue An optional substitute value if the _source value is `null`
*/
public SourceValueFetcher(Set sourcePaths, Object nullValue) {
this.sourcePaths = sourcePaths;
this.nullValue = nullValue;
}
@Override
public List