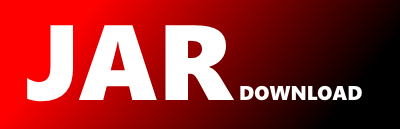
org.elasticsearch.index.get.GetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.get;
import org.elasticsearch.ElasticsearchParseException;
import org.elasticsearch.Version;
import org.elasticsearch.core.RestApiVersion;
import org.elasticsearch.common.Strings;
import org.elasticsearch.common.bytes.BytesReference;
import org.elasticsearch.common.compress.CompressorFactory;
import org.elasticsearch.common.document.DocumentField;
import org.elasticsearch.common.io.stream.StreamInput;
import org.elasticsearch.common.io.stream.StreamOutput;
import org.elasticsearch.common.io.stream.Writeable;
import org.elasticsearch.common.logging.DeprecationLogger;
import org.elasticsearch.common.xcontent.ToXContentObject;
import org.elasticsearch.common.xcontent.XContentBuilder;
import org.elasticsearch.common.xcontent.XContentHelper;
import org.elasticsearch.common.xcontent.XContentParser;
import org.elasticsearch.index.mapper.IgnoredFieldMapper;
import org.elasticsearch.index.mapper.MapperService;
import org.elasticsearch.index.mapper.SourceFieldMapper;
import org.elasticsearch.rest.action.document.RestMultiGetAction;
import org.elasticsearch.search.lookup.SourceLookup;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Objects;
import static java.util.Collections.emptyMap;
import static org.elasticsearch.common.xcontent.XContentParserUtils.ensureExpectedToken;
import static org.elasticsearch.index.seqno.SequenceNumbers.UNASSIGNED_PRIMARY_TERM;
import static org.elasticsearch.index.seqno.SequenceNumbers.UNASSIGNED_SEQ_NO;
public class GetResult implements Writeable, Iterable, ToXContentObject {
private static final DeprecationLogger deprecationLogger = DeprecationLogger.getLogger(GetResult.class);
public static final String _INDEX = "_index";
public static final String _ID = "_id";
private static final String _VERSION = "_version";
private static final String _SEQ_NO = "_seq_no";
private static final String _PRIMARY_TERM = "_primary_term";
private static final String FOUND = "found";
private static final String FIELDS = "fields";
private String index;
private String id;
private long version;
private long seqNo;
private long primaryTerm;
private boolean exists;
private final Map documentFields;
private final Map metaFields;
private Map sourceAsMap;
private BytesReference source;
private byte[] sourceAsBytes;
public GetResult(StreamInput in) throws IOException {
index = in.readString();
if (in.getVersion().before(Version.V_8_0_0)) {
in.readOptionalString();
}
id = in.readString();
seqNo = in.readZLong();
primaryTerm = in.readVLong();
version = in.readLong();
exists = in.readBoolean();
if (exists) {
source = in.readBytesReference();
if (source.length() == 0) {
source = null;
}
documentFields = readFields(in);
metaFields = readFields(in);
} else {
metaFields = Collections.emptyMap();
documentFields = Collections.emptyMap();
}
}
public GetResult(String index, String id, long seqNo, long primaryTerm, long version, boolean exists,
BytesReference source, Map documentFields, Map metaFields) {
this.index = index;
this.id = id;
this.seqNo = seqNo;
this.primaryTerm = primaryTerm;
assert (seqNo == UNASSIGNED_SEQ_NO && primaryTerm == UNASSIGNED_PRIMARY_TERM) || (seqNo >= 0 && primaryTerm >= 1) :
"seqNo: " + seqNo + " primaryTerm: " + primaryTerm;
assert exists || (seqNo == UNASSIGNED_SEQ_NO && primaryTerm == UNASSIGNED_PRIMARY_TERM) :
"doc not found but seqNo/primaryTerm are set";
this.version = version;
this.exists = exists;
this.source = source;
this.documentFields = documentFields == null ? emptyMap() : documentFields;
this.metaFields = metaFields == null ? emptyMap() : metaFields;
}
/**
* Does the document exist.
*/
public boolean isExists() {
return exists;
}
/**
* The index the document was fetched from.
*/
public String getIndex() {
return index;
}
/**
* The id of the document.
*/
public String getId() {
return id;
}
/**
* The version of the doc.
*/
public long getVersion() {
return version;
}
/**
* The sequence number assigned to the last operation that has changed this document, if found.
*/
public long getSeqNo() {
return seqNo;
}
/**
* The primary term of the last primary that has changed this document, if found.
*/
public long getPrimaryTerm() {
return primaryTerm;
}
/**
* The source of the document if exists.
*/
public byte[] source() {
if (source == null) {
return null;
}
if (sourceAsBytes != null) {
return sourceAsBytes;
}
this.sourceAsBytes = BytesReference.toBytes(sourceRef());
return this.sourceAsBytes;
}
/**
* Returns bytes reference, also un compress the source if needed.
*/
public BytesReference sourceRef() {
if (source == null) {
return null;
}
try {
this.source = CompressorFactory.uncompressIfNeeded(this.source);
return this.source;
} catch (IOException e) {
throw new ElasticsearchParseException("failed to decompress source", e);
}
}
/**
* Internal source representation, might be compressed....
*/
public BytesReference internalSourceRef() {
return source;
}
/**
* Is the source empty (not available) or not.
*/
public boolean isSourceEmpty() {
return source == null;
}
/**
* The source of the document (as a string).
*/
public String sourceAsString() {
if (source == null) {
return null;
}
BytesReference source = sourceRef();
try {
return XContentHelper.convertToJson(source, false);
} catch (IOException e) {
throw new ElasticsearchParseException("failed to convert source to a json string");
}
}
/**
* The source of the document (As a map).
*/
public Map sourceAsMap() throws ElasticsearchParseException {
if (source == null) {
return null;
}
if (sourceAsMap != null) {
return sourceAsMap;
}
sourceAsMap = SourceLookup.sourceAsMap(source);
return sourceAsMap;
}
public Map getSource() {
return sourceAsMap();
}
public Map getMetadataFields() {
return metaFields;
}
public Map getDocumentFields() {
return documentFields;
}
public Map getFields() {
Map fields = new HashMap<>();
fields.putAll(metaFields);
fields.putAll(documentFields);
return fields;
}
public DocumentField field(String name) {
return getFields().get(name);
}
@Override
public Iterator iterator() {
// need to join the fields and metadata fields
Map allFields = this.getFields();
return allFields.values().iterator();
}
public XContentBuilder toXContentEmbedded(XContentBuilder builder, Params params) throws IOException {
if (seqNo != UNASSIGNED_SEQ_NO) { // seqNo may not be assigned if read from an old node
builder.field(_SEQ_NO, seqNo);
builder.field(_PRIMARY_TERM, primaryTerm);
}
for (DocumentField field : metaFields.values()) {
// TODO: can we avoid having an exception here?
if (field.getName().equals(IgnoredFieldMapper.NAME)) {
builder.field(field.getName(), field.getValues());
} else {
builder.field(field.getName(), field.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy