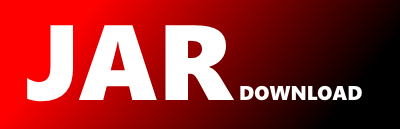
org.elasticsearch.index.fieldvisitor.FieldsVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.fieldvisitor;
import org.apache.lucene.index.FieldInfo;
import org.apache.lucene.index.StoredFieldVisitor;
import org.apache.lucene.util.BytesRef;
import org.elasticsearch.common.bytes.BytesArray;
import org.elasticsearch.common.bytes.BytesReference;
import org.elasticsearch.index.mapper.IdFieldMapper;
import org.elasticsearch.index.mapper.IgnoredFieldMapper;
import org.elasticsearch.index.mapper.LegacyTypeFieldMapper;
import org.elasticsearch.index.mapper.MappedFieldType;
import org.elasticsearch.index.mapper.RoutingFieldMapper;
import org.elasticsearch.index.mapper.SourceFieldMapper;
import org.elasticsearch.index.mapper.Uid;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import static java.util.Collections.emptyMap;
import static java.util.Collections.unmodifiableSet;
import static org.elasticsearch.common.util.set.Sets.newHashSet;
/**
* Base {@link StoredFieldVisitor} that retrieves all non-redundant metadata.
*/
public class FieldsVisitor extends FieldNamesProvidingStoredFieldsVisitor {
private static final Set BASE_REQUIRED_FIELDS = unmodifiableSet(newHashSet(IdFieldMapper.NAME, RoutingFieldMapper.NAME));
private final boolean loadSource;
private final String sourceFieldName;
private final Set requiredFields;
protected BytesReference source;
protected String id;
protected Map> fieldsValues;
public FieldsVisitor(boolean loadSource) {
this(loadSource, SourceFieldMapper.NAME);
}
public FieldsVisitor(boolean loadSource, String sourceFieldName) {
this.loadSource = loadSource;
this.sourceFieldName = sourceFieldName;
requiredFields = new HashSet<>();
reset();
}
@Override
public Status needsField(FieldInfo fieldInfo) {
if (requiredFields.remove(fieldInfo.name)) {
return Status.YES;
}
// Always load _ignored to be explicit about ignored fields
// This works because _ignored is added as the first metadata mapper,
// so its stored fields always appear first in the list.
if (IgnoredFieldMapper.NAME.equals(fieldInfo.name)) {
return Status.YES;
}
// support _uid for loading older indices
if ("_uid".equals(fieldInfo.name)) {
if (requiredFields.remove(IdFieldMapper.NAME) || requiredFields.remove(LegacyTypeFieldMapper.NAME)) {
return Status.YES;
}
}
// All these fields are single-valued so we can stop when the set is
// empty
return requiredFields.isEmpty() ? Status.STOP : Status.NO;
}
@Override
public Set getFieldNames() {
return requiredFields;
}
public final void postProcess(Function fieldTypeLookup) {
for (Map.Entry> entry : fields().entrySet()) {
MappedFieldType fieldType = fieldTypeLookup.apply(entry.getKey());
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy