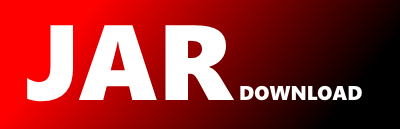
org.elasticsearch.index.mapper.AbstractShapeGeometryFieldMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.mapper;
import org.elasticsearch.common.Explicit;
import org.elasticsearch.common.geo.Orientation;
import org.elasticsearch.index.analysis.NamedAnalyzer;
import java.util.Collections;
import java.util.Map;
import java.util.function.Function;
/**
* Base class for {@link GeoShapeFieldMapper}
*/
public abstract class AbstractShapeGeometryFieldMapper extends AbstractGeometryFieldMapper {
public static Parameter> coerceParam(Function> initializer, boolean coerceByDefault) {
return Parameter.explicitBoolParam("coerce", true, initializer, coerceByDefault);
}
private static final Explicit IMPLICIT_RIGHT = new Explicit<>(Orientation.RIGHT, false);
public static Parameter> orientationParam(Function> initializer) {
return new Parameter<>(
"orientation",
true,
() -> IMPLICIT_RIGHT,
(n, c, o) -> new Explicit<>(Orientation.fromString(o.toString()), true),
initializer,
(b, f, v) -> b.field(f, v.value()),
v -> v.value().toString()
);
}
public abstract static class AbstractShapeGeometryFieldType extends AbstractGeometryFieldType {
private final Orientation orientation;
protected AbstractShapeGeometryFieldType(
String name,
boolean isSearchable,
boolean isStored,
boolean hasDocValues,
Parser parser,
Orientation orientation,
Map meta
) {
super(name, isSearchable, isStored, hasDocValues, parser, meta);
this.orientation = orientation;
}
public Orientation orientation() {
return this.orientation;
}
}
protected Explicit coerce;
protected Explicit orientation;
protected AbstractShapeGeometryFieldMapper(
String simpleName,
MappedFieldType mappedFieldType,
Map indexAnalyzers,
Explicit ignoreMalformed,
Explicit coerce,
Explicit ignoreZValue,
Explicit orientation,
MultiFields multiFields,
CopyTo copyTo,
Parser parser
) {
super(simpleName, mappedFieldType, indexAnalyzers, ignoreMalformed, ignoreZValue, multiFields, copyTo, parser);
this.coerce = coerce;
this.orientation = orientation;
}
protected AbstractShapeGeometryFieldMapper(
String simpleName,
MappedFieldType mappedFieldType,
Explicit ignoreMalformed,
Explicit coerce,
Explicit ignoreZValue,
Explicit orientation,
MultiFields multiFields,
CopyTo copyTo,
Parser parser
) {
this(
simpleName,
mappedFieldType,
Collections.emptyMap(),
ignoreMalformed,
coerce,
ignoreZValue,
orientation,
multiFields,
copyTo,
parser
);
}
public boolean coerce() {
return coerce.value();
}
public Orientation orientation() {
return orientation.value();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy