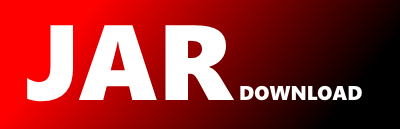
org.elasticsearch.index.mapper.MapperRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.mapper;
import org.elasticsearch.Version;
import org.elasticsearch.plugins.MapperPlugin;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* A registry for all field mappers.
*/
public final class MapperRegistry {
private final Map mapperParsers;
private final Map runtimeFieldParsers;
private final Map metadataMapperParsers;
private final Map metadataMapperParsers7x;
private final Map metadataMapperParsers5x;
private final Function> fieldFilter;
public MapperRegistry(
Map mapperParsers,
Map runtimeFieldParsers,
Map metadataMapperParsers,
Function> fieldFilter
) {
this.mapperParsers = Collections.unmodifiableMap(new LinkedHashMap<>(mapperParsers));
this.runtimeFieldParsers = runtimeFieldParsers;
this.metadataMapperParsers = Collections.unmodifiableMap(new LinkedHashMap<>(metadataMapperParsers));
Map metadata7x = new LinkedHashMap<>(metadataMapperParsers);
metadata7x.remove(NestedPathFieldMapper.NAME);
this.metadataMapperParsers7x = metadata7x;
Map metadata5x = new LinkedHashMap<>(metadata7x);
metadata5x.put(LegacyTypeFieldMapper.NAME, LegacyTypeFieldMapper.PARSER);
this.metadataMapperParsers5x = metadata5x;
this.fieldFilter = fieldFilter;
}
/**
* Return a map of the mappers that have been registered. The
* returned map uses the type of the field as a key.
*/
public Map getMapperParsers() {
return mapperParsers;
}
public Map getRuntimeFieldParsers() {
return runtimeFieldParsers;
}
/**
* Return a map of the meta mappers that have been registered. The
* returned map uses the name of the field as a key.
*/
public Map getMetadataMapperParsers(Version indexCreatedVersion) {
if (indexCreatedVersion.onOrAfter(Version.V_8_0_0)) {
return metadataMapperParsers;
} else if (indexCreatedVersion.major < 6) {
return metadataMapperParsers5x;
} else {
return metadataMapperParsers7x;
}
}
/**
* Return a map of all meta mappers that have been registered in all compatible versions.
*/
public Map getAllMetadataMapperParsers() {
return metadataMapperParsers;
}
/**
* Returns a function that given an index name, returns a predicate that fields must match in order to be returned by get mappings,
* get index, get field mappings and field capabilities API. Useful to filter the fields that such API return.
* The predicate receives the field name as input arguments. In case multiple plugins register a field filter through
* {@link MapperPlugin#getFieldFilter()}, only fields that match all the registered filters will be returned by get mappings,
* get index, get field mappings and field capabilities API.
*/
public Function> getFieldFilter() {
return fieldFilter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy