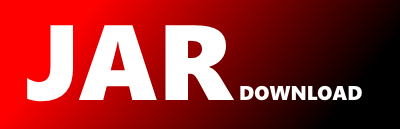
org.elasticsearch.index.mapper.AbstractGeometryFieldMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.index.mapper;
import org.apache.lucene.search.Query;
import org.elasticsearch.common.Explicit;
import org.elasticsearch.common.geo.GeometryFormatterFactory;
import org.elasticsearch.core.CheckedConsumer;
import org.elasticsearch.index.analysis.NamedAnalyzer;
import org.elasticsearch.index.query.SearchExecutionContext;
import org.elasticsearch.xcontent.DeprecationHandler;
import org.elasticsearch.xcontent.NamedXContentRegistry;
import org.elasticsearch.xcontent.XContentParser;
import org.elasticsearch.xcontent.XContentType;
import org.elasticsearch.xcontent.support.MapXContentParser;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Base field mapper class for all spatial field types
*/
public abstract class AbstractGeometryFieldMapper extends FieldMapper {
public static Parameter> ignoreMalformedParam(
Function> initializer,
boolean ignoreMalformedByDefault
) {
return Parameter.explicitBoolParam("ignore_malformed", true, initializer, ignoreMalformedByDefault);
}
public static Parameter> ignoreZValueParam(Function> initializer) {
return Parameter.explicitBoolParam("ignore_z_value", true, initializer, true);
}
/**
* Interface representing parser in geometry indexing pipeline.
*/
public abstract static class Parser {
/**
* Parse the given xContent value to one or more objects of type {@link T}. The value can be
* in any supported format.
*/
public abstract void parse(XContentParser parser, CheckedConsumer consumer, Consumer onMalformed)
throws IOException;
private void fetchFromSource(Object sourceMap, Consumer consumer) {
try (XContentParser parser = wrapObject(sourceMap)) {
parse(parser, v -> consumer.accept(normalizeFromSource(v)), e -> {}); /* ignore malformed */
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Normalize a geometry when reading from source. When reading from source we can skip
* some expensive steps as the geometry has already been indexed.
*/
// TODO: move geometry normalization to the geometry parser.
public abstract T normalizeFromSource(T geometry);
private static XContentParser wrapObject(Object sourceMap) throws IOException {
XContentParser parser = new MapXContentParser(
NamedXContentRegistry.EMPTY,
DeprecationHandler.IGNORE_DEPRECATIONS,
Collections.singletonMap("dummy_field", sourceMap),
XContentType.JSON
);
parser.nextToken(); // start object
parser.nextToken(); // field name
parser.nextToken(); // field value
return parser;
}
}
public abstract static class AbstractGeometryFieldType extends MappedFieldType {
protected final Parser geometryParser;
protected AbstractGeometryFieldType(
String name,
boolean indexed,
boolean stored,
boolean hasDocValues,
Parser geometryParser,
Map meta
) {
super(name, indexed, stored, hasDocValues, TextSearchInfo.NONE, meta);
this.geometryParser = geometryParser;
}
@Override
public final Query termQuery(Object value, SearchExecutionContext context) {
throw new IllegalArgumentException(
"Geometry fields do not support exact searching, use dedicated geometry queries instead: [" + name() + "]"
);
}
/**
* Gets the formatter by name.
*/
protected abstract Function, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy