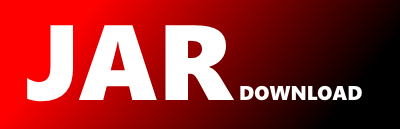
org.elasticsearch.watcher.AbstractResourceWatcher Maven / Gradle / Ivy
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the "Elastic License
* 2.0", the "GNU Affero General Public License v3.0 only", and the "Server Side
* Public License v 1"; you may not use this file except in compliance with, at
* your election, the "Elastic License 2.0", the "GNU Affero General Public
* License v3.0 only", or the "Server Side Public License, v 1".
*/
package org.elasticsearch.watcher;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
/**
* Abstract resource watcher framework, which handles adding and removing listeners
* and calling resource observer.
*/
public abstract class AbstractResourceWatcher implements ResourceWatcher {
private final List listeners = new CopyOnWriteArrayList<>();
private boolean initialized = false;
@Override
public void init() throws IOException {
if (initialized == false) {
doInit();
initialized = true;
}
}
@Override
public void checkAndNotify() throws IOException {
init();
doCheckAndNotify();
}
/**
* Registers new listener
*/
public void addListener(Listener listener) {
listeners.add(listener);
}
/**
* Unregisters a listener
*/
public void remove(Listener listener) {
listeners.remove(listener);
}
/**
* Returns a list of listeners
*/
protected List listeners() {
return listeners;
}
/**
* Will be called once on initialization
*/
protected abstract void doInit() throws IOException;
/**
* Will be called periodically
*
* Implementing watcher should check resource and notify all {@link #listeners()}.
*/
protected abstract void doCheckAndNotify() throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy