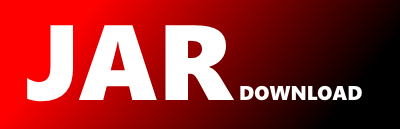
org.elasticsearch.client.internal.node.NodeClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch Show documentation
Show all versions of elasticsearch Show documentation
Elasticsearch - Open Source, Distributed, RESTful Search Engine
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.client.internal.node;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.ActionRequest;
import org.elasticsearch.action.ActionResponse;
import org.elasticsearch.action.ActionType;
import org.elasticsearch.action.support.TransportAction;
import org.elasticsearch.client.internal.Client;
import org.elasticsearch.client.internal.support.AbstractClient;
import org.elasticsearch.cluster.node.DiscoveryNode;
import org.elasticsearch.common.io.stream.NamedWriteableRegistry;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.tasks.Task;
import org.elasticsearch.tasks.TaskCancelledException;
import org.elasticsearch.tasks.TaskManager;
import org.elasticsearch.threadpool.ThreadPool;
import org.elasticsearch.transport.RemoteClusterService;
import org.elasticsearch.transport.Transport;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
/**
* Client that executes actions on the local node.
*/
public class NodeClient extends AbstractClient {
private Map, TransportAction extends ActionRequest, ? extends ActionResponse>> actions;
private TaskManager taskManager;
/**
* The id of the local {@link DiscoveryNode}. Useful for generating task ids from tasks returned by
* {@link #executeLocally(ActionType, ActionRequest, ActionListener)}.
*/
private Supplier localNodeId;
private Transport.Connection localConnection;
private RemoteClusterService remoteClusterService;
private NamedWriteableRegistry namedWriteableRegistry;
public NodeClient(Settings settings, ThreadPool threadPool) {
super(settings, threadPool);
}
public void initialize(
Map, TransportAction extends ActionRequest, ? extends ActionResponse>> actions,
TaskManager taskManager,
Supplier localNodeId,
Transport.Connection localConnection,
RemoteClusterService remoteClusterService,
NamedWriteableRegistry namedWriteableRegistry
) {
this.actions = actions;
this.taskManager = taskManager;
this.localNodeId = localNodeId;
this.localConnection = localConnection;
this.remoteClusterService = remoteClusterService;
this.namedWriteableRegistry = namedWriteableRegistry;
}
/**
* Return the names of all available actions registered with this client.
*/
public List getActionNames() {
return actions.keySet().stream().map(ActionType::name).toList();
}
@Override
public void close() {
// nothing really to do
}
@Override
public void doExecute(
ActionType action,
Request request,
ActionListener listener
) {
// Discard the task because the Client interface doesn't use it.
try {
executeLocally(action, request, listener);
} catch (TaskCancelledException | IllegalArgumentException | IllegalStateException e) {
// #executeLocally returns the task and throws TaskCancelledException if it fails to register the task because the parent
// task has been cancelled, IllegalStateException if the client was not in a state to execute the request because it was not
// yet properly initialized or IllegalArgumentException if header validation fails we forward them to listener since this API
// does not concern itself with the specifics of the task handling
listener.onFailure(e);
}
}
/**
* Execute an {@link ActionType} locally, returning that {@link Task} used to track it, and linking an {@link ActionListener}.
* Prefer this method if you don't need access to the task when listening for the response. This is the method used to
* implement the {@link Client} interface.
*
* @throws TaskCancelledException if the request's parent task has been cancelled already
*/
public Task executeLocally(
ActionType action,
Request request,
ActionListener listener
) {
return taskManager.registerAndExecute(
"transport",
transportAction(action),
request,
localConnection,
new SafelyWrappedActionListener<>(listener)
);
}
/**
* The id of the local {@link DiscoveryNode}. Useful for generating task ids from tasks returned by
* {@link #executeLocally(ActionType, ActionRequest, ActionListener)}.
*/
public String getLocalNodeId() {
return localNodeId.get();
}
/**
* Get the {@link TransportAction} for an {@link ActionType}, throwing exceptions if the action isn't available.
*/
private TransportAction transportAction(
ActionType action
) {
if (actions == null) {
throw new IllegalStateException("NodeClient has not been initialized");
}
@SuppressWarnings("unchecked")
TransportAction transportAction = (TransportAction) actions.get(action);
if (transportAction == null) {
throw new IllegalStateException("failed to find action [" + action + "] to execute");
}
return transportAction;
}
@Override
public Client getRemoteClusterClient(String clusterAlias) {
return remoteClusterService.getRemoteClusterClient(threadPool(), clusterAlias, true);
}
public NamedWriteableRegistry getNamedWriteableRegistry() {
return namedWriteableRegistry;
}
private record SafelyWrappedActionListener(ActionListener listener) implements ActionListener {
@Override
public void onResponse(Response response) {
try {
listener.onResponse(response);
} catch (Exception e) {
assert false : new AssertionError("callback must handle its own exceptions", e);
throw e;
}
}
@Override
public void onFailure(Exception e) {
try {
listener.onFailure(e);
} catch (Exception ex) {
ex.addSuppressed(e);
assert false : new AssertionError("callback must handle its own exceptions", ex);
throw ex;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy