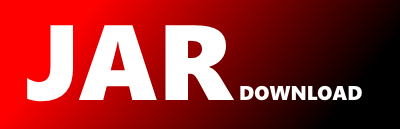
org.emfjson.common.EObjects Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Guillaume Hillairet.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Guillaume Hillairet - initial API and implementation
*
*/
package org.emfjson.common;
import org.eclipse.emf.ecore.*;
import org.eclipse.emf.ecore.impl.DynamicEObjectImpl.BasicEMapEntry;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.emf.ecore.util.FeatureMap;
import org.eclipse.emf.ecore.util.FeatureMapUtil;
import org.emfjson.jackson.JacksonOptions;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
/**
* Utility class to facilitate access or modification of eObjects.
*
*/
public class EObjects {
/**
* Set or add a value to an object reference. The value must be
* an EObject.
*
* @param owner
* @param reference
* @param value
*/
public static void setOrAdd(EObject owner, EReference reference, Object value) {
if (value != null) {
if (reference.isMany()) {
@SuppressWarnings("unchecked")
Collection values = (Collection) owner.eGet(reference);
if (values != null && value instanceof EObject) {
values.add((EObject) value);
}
} else {
owner.eSet(reference, value);
}
}
}
/**
* Set or add a value to an object attribute.
*
* @param owner
* @param attribute
* @param value
*/
public static void setOrAdd(EObject owner, EAttribute attribute, Object value) {
if (attribute.isMany()) {
@SuppressWarnings("unchecked")
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy