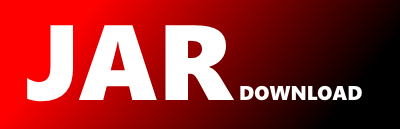
org.enhydra.error.ChainedError Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ChainedError.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.error;
import java.io.PrintStream;
import java.io.PrintWriter;
/**
* Error used as a base for creating an error that has a chain of
* exceptions that lead to the derived error. Very useful for interfaces
* where the implementation exception is not known.
*/
public class ChainedError extends Error implements ChainedThrowable {
/** Causing exception, or null if none */
private Throwable fCause;
/**
* Construct an error without a specified cause.
*
* @param msg The message associated with the exception.
*/
protected ChainedError(String msg) {
super(msg);
fCause = null;
}
/**
* Construct an exception with an associated causing exception.
*
* @param msg The message associated with the exception.
* @param cause The error or exception that cause this exception.
*/
protected ChainedError(String msg,
Throwable cause) {
super(msg);
fCause = cause;
}
/**
* Construct an exception from a causing exception.
*
* @param cause The error or exception that cause this exception. The
* message will be take be this object's messasge.
*/
protected ChainedError(Throwable cause) {
super(ChainedThrowableSupport.makeMessage(cause));
fCause = cause;
}
/**
* Return the message associated with this exception. If causes are
* included, they will be appended to the message.
*/
public String getMessage() {
return ChainedThrowableSupport.getMessage(this, super.getMessage());
}
/**
* Creates a localized description of this exception.
*/
public String getLocalizedMessage() {
return ChainedThrowableSupport.getLocalizedMessage(this, super.getLocalizedMessage());
}
/**
* Get the causing exception associated with this exception.
* @return The causing exception or null if no cause is specified.
*/
public Throwable getCause() {
return fCause;
}
/**
* Prints this exception and its backtrace, and the causes and their stack
* traces to the standard error stream.
*/
public void printStackTrace() {
super.printStackTrace();
ChainedThrowableSupport.printCauseTrace(this);
}
/**
* Prints this exception and its backtrace, and the causes and their stack
* traces to the e specified print stream.
*/
public void printStackTrace(PrintStream s) {
super.printStackTrace(s);
ChainedThrowableSupport.printCauseTrace(this, s);
}
/**
* Prints this exception and its backtrace, and the causes and their stack
* traces to the e specified print writer.
*/
public void printStackTrace(PrintWriter s) {
super.printStackTrace(s);
ChainedThrowableSupport.printCauseTrace(this, s);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy