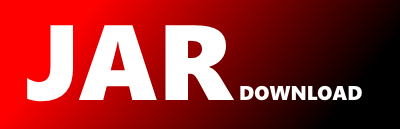
org.enhydra.wireless.chtml.dom.xerces.CHTMLDocumentImpl Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* Contributor(s):
*
* $Id: CHTMLDocumentImpl.java,v 1.5 2005/01/26 08:28:45 jkjome Exp $
*/
package org.enhydra.wireless.chtml.dom.xerces;
import java.lang.reflect.Constructor;
import java.util.HashMap;
import org.enhydra.apache.html.dom.HTMLAnchorElementImpl;
import org.enhydra.apache.html.dom.HTMLBRElementImpl;
import org.enhydra.apache.html.dom.HTMLBaseElementImpl;
import org.enhydra.apache.html.dom.HTMLBodyElementImpl;
import org.enhydra.apache.html.dom.HTMLDListElementImpl;
import org.enhydra.apache.html.dom.HTMLDOMImplementationImpl;
import org.enhydra.apache.html.dom.HTMLDirectoryElementImpl;
import org.enhydra.apache.html.dom.HTMLDivElementImpl;
import org.enhydra.apache.html.dom.HTMLDocumentImpl;
import org.enhydra.apache.html.dom.HTMLElementImpl;
import org.enhydra.apache.html.dom.HTMLFontElementImpl;
import org.enhydra.apache.html.dom.HTMLFormElementImpl;
import org.enhydra.apache.html.dom.HTMLHRElementImpl;
import org.enhydra.apache.html.dom.HTMLHeadElementImpl;
import org.enhydra.apache.html.dom.HTMLHeadingElementImpl;
import org.enhydra.apache.html.dom.HTMLHtmlElementImpl;
import org.enhydra.apache.html.dom.HTMLImageElementImpl;
import org.enhydra.apache.html.dom.HTMLInputElementImpl;
import org.enhydra.apache.html.dom.HTMLLIElementImpl;
import org.enhydra.apache.html.dom.HTMLMenuElementImpl;
import org.enhydra.apache.html.dom.HTMLMetaElementImpl;
import org.enhydra.apache.html.dom.HTMLOListElementImpl;
import org.enhydra.apache.html.dom.HTMLOptionElementImpl;
import org.enhydra.apache.html.dom.HTMLParagraphElementImpl;
import org.enhydra.apache.html.dom.HTMLPreElementImpl;
import org.enhydra.apache.html.dom.HTMLQuoteElementImpl;
import org.enhydra.apache.html.dom.HTMLSelectElementImpl;
import org.enhydra.apache.html.dom.HTMLTextAreaElementImpl;
import org.enhydra.apache.html.dom.HTMLTitleElementImpl;
import org.enhydra.apache.html.dom.HTMLUListElementImpl;
import org.enhydra.wireless.chtml.dom.CHTMLDocument;
import org.enhydra.xml.xmlc.XMLObject;
import org.enhydra.xml.xmlc.XMLObjectLink;
import org.w3c.dom.DOMException;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Element;
/**
* CHTML Document interfaces. This implements a subset of the HTML DOM that
* corresponds to CHTML defined by DoCoMo. See
* CHTML specification
* for details.
*
* @see org.w3c.dom.html.HTMLDocument
*/
public class CHTMLDocumentImpl extends HTMLDocumentImpl
implements CHTMLDocument, XMLObjectLink {
/**
* Names and classes of HTML element types.
*/
private static HashMap fElementTypes;
/**
* Signature used to locate constructor of HTML element classes.
*/
private static final Class[] fElemClassSig = new Class[] {
HTMLDocumentImpl.class, String.class
};
/**
* overload in order to return the correct dom implementation for cHTML (actually HTML)
* @see org.enhydra.apache.xerces.dom.DocumentImpl#getImplementation
*/
public DOMImplementation getImplementation() {
return HTMLDOMImplementationImpl.getDOMImplementation();
}
/**
* @see HTMLDocumentImpl#createElementNS
*/
public Element createElementNS(String namespaceURI,
String qualifiedName) throws DOMException {
if ((namespaceURI == null) || (namespaceURI.length() == 0)) {
return createElement(qualifiedName);
} else {
return super.createElementNS(namespaceURI, qualifiedName);
}
}
/**
* @see HTMLDocumentImpl#createElement
*/
public Element createElement(String tagName) throws DOMException {
Class elemClass;
Constructor cnst;
elemClass = (Class)fElementTypes.get(tagName.toUpperCase());
if (elemClass != null) {
try {
cnst = elemClass.getConstructor(fElemClassSig);
return (Element)cnst.newInstance(new Object[] {
this, tagName
});
} catch (Exception except) {
Throwable thrw;
if (except instanceof java.lang.reflect.InvocationTargetException) {
thrw =
((java.lang.reflect.InvocationTargetException) except).getTargetException();
} else {
thrw = except;
}
throw new IllegalStateException("HTM15 Tag '" + tagName
+ "' associated with an Element class that failed to construct.\n"
+ thrw.getClass().getName() + " " + thrw.getMessage());
}
}
return new HTMLElementImpl(this, tagName);
}
/**
* Class initializer.
*/
static {
fElementTypes = new HashMap();
fElementTypes.put("A", HTMLAnchorElementImpl.class);
fElementTypes.put("BASE", HTMLBaseElementImpl.class);
fElementTypes.put("BLOCKQUOTE", HTMLQuoteElementImpl.class);
fElementTypes.put("BODY", HTMLBodyElementImpl.class);
fElementTypes.put("BR", HTMLBRElementImpl.class);
fElementTypes.put("DIR", HTMLDirectoryElementImpl.class);
fElementTypes.put("DIV", HTMLDivElementImpl.class);
fElementTypes.put("DL", HTMLDListElementImpl.class);
fElementTypes.put("FONT", HTMLFontElementImpl.class);
fElementTypes.put("FORM", HTMLFormElementImpl.class);
fElementTypes.put("H1", HTMLHeadingElementImpl.class);
fElementTypes.put("H2", HTMLHeadingElementImpl.class);
fElementTypes.put("H3", HTMLHeadingElementImpl.class);
fElementTypes.put("H4", HTMLHeadingElementImpl.class);
fElementTypes.put("H5", HTMLHeadingElementImpl.class);
fElementTypes.put("H6", HTMLHeadingElementImpl.class);
fElementTypes.put("HEAD", HTMLHeadElementImpl.class);
fElementTypes.put("HEADING", HTMLHeadingElementImpl.class);
fElementTypes.put("HR", HTMLHRElementImpl.class);
fElementTypes.put("HTML", HTMLHtmlElementImpl.class);
fElementTypes.put("IMG", HTMLImageElementImpl.class);
fElementTypes.put("INPUT", HTMLInputElementImpl.class);
fElementTypes.put("LI", HTMLLIElementImpl.class);
fElementTypes.put("MENU", HTMLMenuElementImpl.class);
fElementTypes.put("META", HTMLMetaElementImpl.class);
fElementTypes.put("OL", HTMLOListElementImpl.class);
fElementTypes.put("OPTION", HTMLOptionElementImpl.class);
fElementTypes.put("P", HTMLParagraphElementImpl.class);
fElementTypes.put("PLAINTEXT", HTMLPreElementImpl.class);
fElementTypes.put("PRE", HTMLPreElementImpl.class);
fElementTypes.put("SELECT", HTMLSelectElementImpl.class);
fElementTypes.put("TEXTAREA", HTMLTextAreaElementImpl.class);
fElementTypes.put("TITLE", HTMLTitleElementImpl.class);
fElementTypes.put("UL", HTMLUListElementImpl.class);
}
//-------------------------------------------------------------------------
// XMLObjectLink implementation
//-------------------------------------------------------------------------
/**
* Reference to XMLObject containing this Document.
*/
private XMLObject fXmlObjectLink;
/**
* @see XMLObjectLink#setXMLObject
*/
public void setXMLObject(XMLObject xmlObject) {
fXmlObjectLink = xmlObject;
}
/**
* @see XMLObjectLink#getXMLObject
*/
public XMLObject getXMLObject() {
return fXmlObjectLink;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy