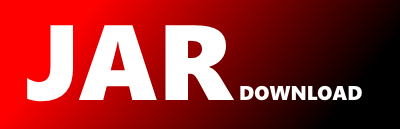
org.enhydra.wireless.voicexml.dom.xerces.VoiceXMLDocumentImpl Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Original Code is DigitalSesame
* Portions created by DigitalSesame are Copyright (C) 1997-2000 DigitalSesame
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: VoiceXMLDocumentImpl.java,v 1.5 2005/04/05 06:17:30 jkjome Exp $
*/
package org.enhydra.wireless.voicexml.dom.xerces;
import java.lang.reflect.Constructor;
import java.util.Hashtable;
import org.enhydra.apache.xerces.dom.DocumentImpl;
import org.enhydra.wireless.voicexml.dom.VoiceXMLDocument;
import org.enhydra.xml.xmlc.XMLCError;
import org.enhydra.xml.xmlc.XMLObject;
import org.enhydra.xml.xmlc.XMLObjectLink;
import org.w3c.dom.DOMException;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
public class VoiceXMLDocumentImpl extends DocumentImpl implements VoiceXMLDocument, XMLObjectLink {
private static Hashtable fElementTypes;
private static final Class[] fElemConstructorSig
= new Class[] {VoiceXMLDocumentImpl.class, String.class, String.class};
/**
* overload in order to return the correct dom implementation for VoiceXML
* @see org.enhydra.apache.xerces.dom.DocumentImpl#getImplementation
*/
public DOMImplementation getImplementation() {
return VoiceXMLDOMImplementationImpl.getDOMImplementation();
}
public Element createElementNS(String namespaceURI,
String qualifiedName) throws DOMException {
// FIXME: should be a common method for doing this
int index = qualifiedName.indexOf(':');
String tagName;
if (index < 0) {
tagName = qualifiedName;
} else {
tagName = qualifiedName.substring(index+1);
}
Class elemClass = (Class)fElementTypes.get(tagName);
if (elemClass != null) {
try {
Constructor cnst = elemClass.getConstructor(fElemConstructorSig);
return (Element)cnst.newInstance(new Object[] {this, namespaceURI, qualifiedName});
} catch (Exception except) {
// Need more specific error..
throw new XMLCError("failed to construct element for \""
+ qualifiedName + "\"", except);
}
} else {
return new VoiceXMLElementImpl(this, null, tagName);
}
}
public Element createElement(String tagName) throws DOMException {
return createElementNS(null, tagName);
}
static {
fElementTypes = new Hashtable();
fElementTypes.put("exit", VoiceXMLExitElementImpl.class);
fElementTypes.put("script", VoiceXMLScriptElementImpl.class);
fElementTypes.put("link", VoiceXMLLinkElementImpl.class);
fElementTypes.put("param", VoiceXMLParamElementImpl.class);
fElementTypes.put("meta", VoiceXMLMetaElementImpl.class);
fElementTypes.put("field", VoiceXMLFieldElementImpl.class);
fElementTypes.put("enumerate", VoiceXMLEnumerateElementImpl.class);
fElementTypes.put("block", VoiceXMLBlockElementImpl.class);
fElementTypes.put("throw", VoiceXMLThrowElementImpl.class);
fElementTypes.put("subdialog", VoiceXMLSubdialogElementImpl.class);
fElementTypes.put("pros", VoiceXMLProsElementImpl.class);
fElementTypes.put("else", VoiceXMLElseElementImpl.class);
fElementTypes.put("goto", VoiceXMLGotoElementImpl.class);
fElementTypes.put("submit", VoiceXMLSubmitElementImpl.class);
fElementTypes.put("audio", VoiceXMLAudioElementImpl.class);
fElementTypes.put("sayas", VoiceXMLSayasElementImpl.class);
fElementTypes.put("var", VoiceXMLVarElementImpl.class);
fElementTypes.put("break", VoiceXMLBreakElementImpl.class);
fElementTypes.put("error", VoiceXMLErrorElementImpl.class);
fElementTypes.put("emp", VoiceXMLEmpElementImpl.class);
fElementTypes.put("transfer", VoiceXMLTransferElementImpl.class);
fElementTypes.put("help", VoiceXMLHelpElementImpl.class);
fElementTypes.put("noinput", VoiceXMLNoinputElementImpl.class);
fElementTypes.put("if", VoiceXMLIfElementImpl.class);
fElementTypes.put("choice", VoiceXMLChoiceElementImpl.class);
fElementTypes.put("form", VoiceXMLFormElementImpl.class);
fElementTypes.put("clear", VoiceXMLClearElementImpl.class);
fElementTypes.put("nomatch", VoiceXMLNomatchElementImpl.class);
fElementTypes.put("option", VoiceXMLOptionElementImpl.class);
fElementTypes.put("filled", VoiceXMLFilledElementImpl.class);
fElementTypes.put("record", VoiceXMLRecordElementImpl.class);
fElementTypes.put("elseif", VoiceXMLElseifElementImpl.class);
fElementTypes.put("object", VoiceXMLObjectElementImpl.class);
fElementTypes.put("value", VoiceXMLValueElementImpl.class);
fElementTypes.put("prompt", VoiceXMLPromptElementImpl.class);
fElementTypes.put("property", VoiceXMLPropertyElementImpl.class);
fElementTypes.put("grammar", VoiceXMLGrammarElementImpl.class);
fElementTypes.put("vxml", VoiceXMLVxmlElementImpl.class);
fElementTypes.put("disconnect", VoiceXMLDisconnectElementImpl.class);
fElementTypes.put("assign", VoiceXMLAssignElementImpl.class);
fElementTypes.put("catch", VoiceXMLCatchElementImpl.class);
fElementTypes.put("return", VoiceXMLReturnElementImpl.class);
fElementTypes.put("div", VoiceXMLDivElementImpl.class);
fElementTypes.put("menu", VoiceXMLMenuElementImpl.class);
fElementTypes.put("reprompt", VoiceXMLRepromptElementImpl.class);
fElementTypes.put("initial", VoiceXMLInitialElementImpl.class);
fElementTypes.put("dtmf", VoiceXMLDtmfElementImpl.class);
}
/* DOM level 2 */
public VoiceXMLDocumentImpl(DocumentType doctype) {
super(doctype, false);
}
public synchronized Element getElementById( String elementId )
{
return getElementById( elementId, this );
}
/**
* Recursive method retreives an element by its id
attribute.
* Called by {@link #getElementById(String)}.
*
* @param elementId The id
value to look for
* @return The node in which to look for
*/
private Element getElementById( String elementId, Node node )
{
Node child;
Element result;
child = node.getFirstChild();
while ( child != null )
{
if ( child instanceof Element )
{
if ( elementId.equals( ( (Element) child ).getAttribute( "id" ) ) )
return (Element) child;
result = getElementById( elementId, child );
if ( result != null )
return result;
}
child = child.getNextSibling();
}
return null;
}
//-------------------------------------------------------------------------
// XMLObjectLink implementation
//-------------------------------------------------------------------------
/**
* Reference to XMLObject containing this Document.
*/
private XMLObject fXmlObjectLink;
/**
* @see XMLObjectLink#setXMLObject
*/
public void setXMLObject(XMLObject xmlObject) {
fXmlObjectLink = xmlObject;
}
/**
* @see XMLObjectLink#getXMLObject
*/
public XMLObject getXMLObject() {
return fXmlObjectLink;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy