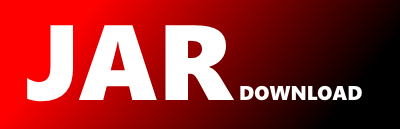
org.enhydra.wireless.wml.WMLDomFactory Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Original Code is DigitalSesame
* Portions created by DigitalSesame are Copyright (C) 1997-2000 DigitalSesame
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: WMLDomFactory.java,v 1.2 2005/01/26 08:28:45 jkjome Exp $
*/
package org.enhydra.wireless.wml;
import java.util.HashSet;
import java.util.StringTokenizer;
import org.enhydra.apache.xerces.dom.DocumentTypeImpl;
import org.enhydra.wireless.wml.dom.WMLDocument;
import org.enhydra.wireless.wml.dom.xerces.WMLDOMImplementationImpl;
import org.enhydra.xml.xmlc.XMLCError;
import org.enhydra.xml.xmlc.dom.XMLCDomFactory;
import org.enhydra.xml.xmlc.dom.xerces.XercesDomFactory;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
/**
* XMLC DOM factory for creating WML-specified DocumentType and Document
* objects. Specifying this class as the XMLCDomFctory
to xmlc
* will produce XMLC document class that are WMLDocument
classes.
* This is specified using:
*
*
* xmlc -dom-factory org.enhydra.wireless.wml.WMLDomFactory
*
*/
public class WMLDomFactory extends XercesDomFactory {
/**
* WML URL attributes.
*/
private static String[] URL_ATTRIBUTES = {
"onenterforward", "onenterbackward",
"ontimer", "href",
"onpick", "src"
};
/**
* HashSet built from URL_ATTRIBUTES.
*/
private static final HashSet urlAttributes;
private static final String IMPL_CLASS_SUFFIX = "Impl";
private static final String WML_IMPLEMENTATION_DOT = "org.enhydra.wireless.wml.dom.xerces";
private static final String WML_INTERFACE_DOT = "org.enhydra.wireless.wml.dom";
/**
* Class initializer.
*/
static {
urlAttributes = new HashSet(URL_ATTRIBUTES.length);
for (int idx = 0; idx < URL_ATTRIBUTES.length; idx++) {
urlAttributes.add(URL_ATTRIBUTES[idx]);
}
}
/**
* @see XMLCDomFactory#createDocumentType
*/
public DocumentType createDocumentType(String qualifiedName,
String publicId,
String systemId,
String internalSubset) {
DOMImplementation domImpl = WMLDOMImplementationImpl.getDOMImplementation();
DocumentTypeImpl docType = (DocumentTypeImpl)domImpl.createDocumentType(qualifiedName, publicId, systemId);
docType.setInternalSubset(internalSubset);
return docType;
}
/**
* @see XMLCDomFactory#createDocument
*/
public Document createDocument(String namespaceURI,
String qualifiedName,
DocumentType docType) {
DOMImplementation domImpl = WMLDOMImplementationImpl.getDOMImplementation();
Document doc = domImpl.createDocument(namespaceURI, qualifiedName,
docType);
return doc;
}
/**
* @see XMLCDomFactory#getMIMEType
*/
public String getMIMEType() {
return "text/vnd.wap.wml";
}
/**
* @see XMLCDomFactory#getInterfaceNames
*/
public String[] getInterfaceNames() {
return new String[] {WMLDocument.class.getName()};
}
/**
* @see XMLCDomFactory#nodeClassToInterface
*/
public String nodeClassToInterface(Node node) {
String ret = null;
String className = node.getClass().getName();
if (className.startsWith(WML_IMPLEMENTATION_DOT)) {
int suffixIdx = className.lastIndexOf(IMPL_CLASS_SUFFIX);
if (suffixIdx < 0) {
throw new XMLCError("Class \"" + className
+ "\" does not have suffix \"" + IMPL_CLASS_SUFFIX
+ "\" (maybe be mismatch between XMLC code DOM implementation)");
}
ret = WML_INTERFACE_DOT +
className.substring(WML_IMPLEMENTATION_DOT.length(), suffixIdx);
} else
ret = super.nodeClassToInterface(node);
return ret;
}
/**
* @see XMLCDomFactory#getElementClassNames
*/
public String[] getElementClassNames(Element elem) {
String classNames = elem.getAttribute("class");
if ((classNames == null) || (classNames.length() ==0)) {
return null;
}
// Parse the class name.
StringTokenizer tokens = new StringTokenizer(classNames);
String[] names = new String[tokens.countTokens()];
for (int idx = 0; idx < names.length; idx++) {
names[idx] = tokens.nextToken();
}
return names;
}
/**
* @see XMLCDomFactory#isURLAttribute
*/
public boolean isURLAttribute(Element element,
String attrName) {
return urlAttributes.contains(attrName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy