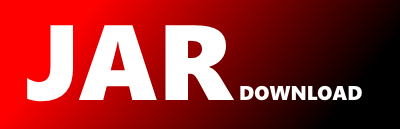
org.enhydra.xml.dom.SimpleDOMTraversal Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: SimpleDOMTraversal.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.dom;
import org.enhydra.xml.lazydom.LazyDOMSimpleTraversal;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
//FIXME: Makes so it doesn't expand, but LazyDOM counts on this.
/**
* Simple DOM traverser that calls a handler for every node in the DOM tree.
*/
public class SimpleDOMTraversal {
/**
* Interface for node callback object.
*/
public interface Handler {
/**
* Handler called for each Node.
*/
public void handleNode(Node node);
}
/** Handler object for the traversal */
protected Handler fHandler;
/**
* Constructor.
* @param handler The object that will be called to handle each
* node.
*/
public SimpleDOMTraversal(SimpleDOMTraversal.Handler handler) {
fHandler = handler;
}
/**
* Traverse a DOM tree or subtree.
*
* @param root The root of the DOM tree or subtree that is to
* be traversed.
*/
public void traverse(Node root) {
processNode(root);
}
/**
* Process the children of a node.
*/
protected void processChildren(Node node) {
//FIXME: Xerces sometimes has DocumentType objects as children (bug?)
for (Node child = node.getFirstChild(); child != null;
child = child.getNextSibling()) {
if (!(child instanceof DocumentType)) {
processNode(child);
}
}
}
/**
* Process the attributes of an element.
*/
protected void processAttributes(Node node) {
NamedNodeMap attrMap = node.getAttributes();
int len = attrMap.getLength();
for (int i = 0; i < len; i++) {
processNode(attrMap.item(i));
}
}
/**
* Process a DocumentType attribute of a Document node, if it exists.
*/
public void processDocumentType(Document document) {
DocumentType docType = document.getDoctype();
if (docType != null) {
processNode(docType);
}
}
/**
* Process the contents of a DocumentType node,
*/
protected void processDocumentTypeContents(DocumentType documentType) {
processNamedNodeMap(documentType.getEntities());
processNamedNodeMap(documentType.getNotations());
}
/**
* Process contents of a NamedNodeMap.
*/
private void processNamedNodeMap(NamedNodeMap nodeMap) {
if (nodeMap != null) {
int len = nodeMap.getLength();
for (int i = 0; i < len; i++) {
processNode(nodeMap.item(i));
}
}
}
/**
* Processing based on node type. All nodes go through here.
*/
protected void processNode(Node node) {
fHandler.handleNode(node);
if (node instanceof Document) {
processDocumentType((Document)node);
} else if (node instanceof DocumentType) {
DocumentType docType = (DocumentType)node;
processNamedNodeMap(docType.getEntities());
processNamedNodeMap(docType.getNotations());
} else if (node instanceof Element) {
processAttributes(node);
}
processChildren(node);
}
/**
* Factory method to create a traverser based on the type of
* a document.
*/
public static SimpleDOMTraversal getTraverser(SimpleDOMTraversal.Handler handler,
Node node) {
/*
* Find the document to determine type. The LazyDOM traverser is only
* used on instance documents. If a template DOM node is specified,
* the template LazyDocument is traverse as any other done.
*/
if (DOMOps.isLazyDOMInstance(DOMOps.getDocument(node))) {
return new LazyDOMSimpleTraversal(handler);
} else {
return new SimpleDOMTraversal(handler);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy