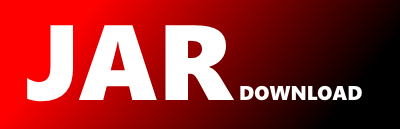
org.enhydra.xml.io.CharacterSet Maven / Gradle / Ivy
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: CharacterSet.java,v 1.1.1.1 2003/03/10 16:36:16 taweili Exp $
*/
package org.enhydra.xml.io;
/**
* Information and operations associated with a specific character set.
* A global table of these objects is built by the Encodings class.
* Instances are immutable, classes can be derived to define override
* the operations that can't be handled by the default static parameters.
*/
class CharacterSet {
/** Encoding name */
private final String fName;
/** Size of characters, in bits */
private final int fCharSize;
/** The maximum value of a character */
private final int fMaxCharValue;
/** MIME prefered name, null if not specified. */
private final String fMimePreferred;
/** List of aliases, zero length if none */
private final String[] fAliases;
/** Constructor */
public CharacterSet(String name,
int charSize,
String mimePreferred,
String[] aliases) {
fName = name;
fCharSize = charSize;
fMimePreferred = mimePreferred;
fAliases = aliases;
// Roughly determine the maximum value of a character.
int maxCharValue;
if (charSize == 7) {
maxCharValue = 0x7e;
} else if (charSize == 8) {
maxCharValue = 0xFF;
} else {
maxCharValue = 0xFFFF;
}
fMaxCharValue = maxCharValue;
}
/** Get the name */
public final String getName() {
return fName;
}
/** Get the character size, in bits */
public final int getCharSize() {
return fCharSize;
}
/** Get the maximum value for a character */
public final int getMaxCharValue() {
return fMaxCharValue;
}
/** Get the MIME preferred name or null if unspecified */
public final String getMIMEPreferred() {
return fMimePreferred;
}
/** Get the aliases */
public final String[] getAliases() {
return fAliases;
}
// FIXME: do this correctly, but requires a com.sun class...
/**
* Determine if a unicode character has a valid mapping to this
* character set.
* WARNING: This is only well implemented for a few character sets
* on an as-needed basis; the rest just go by the character size.
*/
public boolean isValid(char ch) {
return (ch <= fMaxCharValue);
}
/**
* Determine if another character set has the same valid range of
* character codes as this character set. That is, will
* isValid()
return the same value for any arbitrary
* unicode character.
*
WARNING: This is only well implemented for a few character sets
* on an as-needed basis; the rest just go by the character size.
* @deprecated use {qlink #isCompatible} instead
*/
public boolean sameValidCharRange(CharacterSet otherSet) {
return isCompatible(otherSet);
}
/**
* Determine if another character set is compatible to this character set.
* "Compatible" means that for every character where
* otherSet.isValid()
return true
,
* this.isValid()
will return true
as well.
*
WARNING: This is only well implemented for a few character sets
* on an as-needed basis; the rest just go by the character set size.
*/
public boolean isCompatible(CharacterSet otherSet) {
return (otherSet.getMaxCharValue() == fMaxCharValue);
}
/** Get string representation of object */
public String toString() {
StringBuffer buf = new StringBuffer(256); // larger than default
buf.append(fName);
buf.append(": ");
buf.append(fCharSize);
buf.append(' ');
buf.append(fMimePreferred);
for (int i = 1; i < fAliases.length; i++) {
buf.append(' ');
buf.append(fAliases[i]);
}
return buf.toString();
}
}