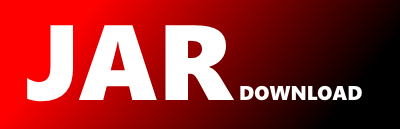
org.enhydra.xml.io.DOMParser Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: DOMParser.java,v 1.5 2005/10/23 03:15:42 jkjome Exp $
*/
package org.enhydra.xml.io;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import org.enhydra.apache.xerces.dom.DOMImplementationImpl;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.xml.sax.EntityResolver;
import org.xml.sax.ErrorHandler;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import org.xml.sax.SAXNotRecognizedException;
// FIXME: Not completely sure how useful this is. It was originally done
// before JAXP was availble in Xerces, was suppose to evolve into a more
// general facility, but is now just used by XMLC.
/**
* XML parser that parsers to a DOM. This implements the JAXP DOM parser
* interface plus the following additional features:
*
*
* - Control over the Document class that is created. This easily
* supports building a DTD-specific DOM.
*
- Simplified error handling.
*
- Finding the initial document via the entity resolver.
*
*
* @see javax.xml.parsers.DocumentBuilder
*/
public class DOMParser extends DocumentBuilder {
/**
* Class name to use for the Document object.
*/
private String documentClassName = null;
/**
* Error handler.
*/
private ErrorHandler errorHandler = null;
/**
* Entity resolver.
*/
private EntityResolver entityResolver = null;
/**
* Namespaces enabled.
*/
private boolean enableNamespaces = true;
/**
* Validation enabled.
*/
private boolean validationEnabled = true;
//FIXME: ErrorReporter reporter = new ErrorReporter(new PrintWriter(System.err, true));
//FIXME: should deal with ignorable white space.
/**
* Need standarderror handler, this is just a tmp hack.
*/
class XercesParser extends org.enhydra.apache.xerces.parsers.DOMParser {
/**
* Construct a parser.
*/
public XercesParser() throws SAXException {
if (documentClassName != null) {
super.setDocumentClassName(documentClassName);
}
if (errorHandler != null) {
super.setErrorHandler(errorHandler);
}
if (entityResolver != null) {
super.setEntityResolver(entityResolver);
}
super.setNamespaces(enableNamespaces);
super.setValidation(validationEnabled);
super.setDeferNodeExpansion(false);
}
/**
* Resolve an input source using the entity resolver.
* @return The resolved source, or the originally specified
* source if it can't be resolved or is already open.
*/
private InputSource resolve(InputSource inputSource)
throws SAXException, IOException {
if ((inputSource.getByteStream() != null)
|| (inputSource.getCharacterStream() != null)) {
return inputSource; // Already open
}
EntityResolver resolver = this.getEntityResolver();
if (resolver == null) {
return inputSource; // No resolver
}
String publicId = inputSource.getPublicId();
String systemId = inputSource.getSystemId();
if ((publicId == null) && (systemId == null)) {
// Shouldn't happen, but let parser generate error
return inputSource;
}
InputSource resolvedSource = resolver.resolveEntity(publicId,
systemId);
if (resolvedSource != null) {
return resolvedSource;
} else {
return inputSource;
}
}
/**
* Parse the document, possibly resolving it via the entity
* resolver.
*/
public void parse(InputSource inputSource)
throws SAXException, IOException {
super.parse(resolve(inputSource));
}
}
//FIXME: Throws exception on parse failure, but ErrorReporter my be
// collectin output in memory. Recompiler deals with this, but it might
// be nice to include a standard mechanism.
/**
* Parse the content of the given input source as an XML document
* and return a new DOM Document object.
*
* @param is InputSource containing the content to be parsed.
* @exception IOException If any IO errors occur.
* @exception SAXException If any parse errors occur.
* @exception IllegalArgumentException If the InputSource is null
* @see javax.xml.parsers.DocumentBuilder#parse
*/
public Document parse(InputSource is)
throws SAXException, IOException {
XercesParser parser = new XercesParser();
parser.parse(is);
return parser.getDocument();
}
/**
* Enable or disable namespaces.
*/
public void setNamespaceAware(boolean enable) {
enableNamespaces = enable;
}
/**
* Indicates whether or not this parser is configured to
* understand namespaces.
* @see javax.xml.parsers.DocumentBuilder#isNamespaceAware
*/
public boolean isNamespaceAware() {
return enableNamespaces;
}
/**
* Enable or disable validation.
*/
public void setValidation(boolean enable) {
validationEnabled = enable;
}
/**
* Indicates whether or not this parser is configured to
* validate XML documents.
* @see javax.xml.parsers.DocumentBuilder#isValidating
*/
public boolean isValidating() {
return validationEnabled;
}
/**
* Specify the EntityResolver
to be used to resolve
* entities present in the XML document to be parsed. Setting
* this to null
will result in the underlying
* implementation using it's own default implementation and
* behavior.
* @see javax.xml.parsers.DocumentBuilder#setEntityResolver
*/
public void setEntityResolver(EntityResolver er) {
entityResolver = er;
}
/**
* Get the EntityResolver
*/
public EntityResolver getEntityResolver() {
return entityResolver;
}
/**
* Specify the ErrorHandler
to be used handle parse
* errors. Setting
* this to null
will result in the underlying
* implementation using it's own default implementation and
* behavior.
* @see javax.xml.parsers.DocumentBuilder#setErrorHandler
*/
public void setErrorHandler(ErrorHandler eh) {
errorHandler = eh;
}
/**
* Get the ErrorHandler
.
*/
public ErrorHandler getErrorHandler() {
return errorHandler;
}
/**
* Set the document class for the document to construct.
* FIXME: need by-class object specificaion.
*/
public void setDocumentClassName(String className) {
documentClassName = className;
}
/**
* get the document class for the document to construct.
*/
public String getDocumentClassName() {
return documentClassName;
}
/**
* Obtain a new instance of a DOM Document object to build a DOM
* tree with.
* @see javax.xml.parsers.DocumentBuilder#newDocument
*/
public Document newDocument() {
String className = (documentClassName != null)
? documentClassName : org.enhydra.apache.xerces.parsers.DOMParser.DEFAULT_DOCUMENT_CLASS_NAME;
try {
Class documentClass = getClass().getClassLoader().loadClass(className);
return (Document)documentClass.newInstance();
} catch (Exception except) {
throw new XMLIOError(except);
}
}
/**
* Obtain an instance of a {@link org.w3c.dom.DOMImplementation} object.
*
* @return A new instance of a DOMImplementation
.
*/
public DOMImplementation getDOMImplementation() {
return DOMImplementationImpl.getDOMImplementation();
}
/**
* Generate a description of various attributes of the parser
* for debugging purposes.
*/
public String toString() {
try {
StringBuffer buf = new StringBuffer(4096);
// Get a parser object with the current settings.
XercesParser parser = new XercesParser();
//FIXME: getFeaturesRecognized() actually returns
// unrecognized features, hence the checks.
String[] features = parser.getFeaturesRecognized();
buf.append("Parser features:\n");
for (int idx = 0; idx < features.length; idx++) {
buf.append(" ");
buf.append(features[idx]);
buf.append("=");
try {
buf.append(parser.getFeature(features[idx]));
} catch (SAXNotRecognizedException except) {
buf.append("*** not recognized ***");
}
buf.append('\n');
}
String[] properties = parser.getPropertiesRecognized();
buf.append("Parser properties:\n");
for (int idx = 0; idx < properties.length; idx++) {
buf.append(" ");
buf.append(properties[idx]);
buf.append("=");
try {
buf.append(parser.getFeature(properties[idx]));
} catch (SAXNotRecognizedException except) {
buf.append("*** not recognized ***");
}
buf.append('\n');
}
return buf.toString();
} catch (SAXException except) {
throw new XMLIOError(except);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy