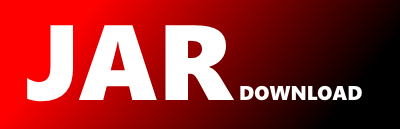
org.enhydra.xml.io.ErrorReporter Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: ErrorReporter.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.io;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.io.StringWriter;
import org.xml.sax.ErrorHandler;
import org.xml.sax.Locator;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
//FIXME: Make util.ChainedThrowableUtil more general and used for
//print stack trace.
/**
* Object used to report error message for the user. Can write eror messages
* to a file or save them in a memory buffer.
* This is also a SAX compliant ErrorHandler.
*/
public class ErrorReporter implements ErrorHandler {
/*
* Error message are written to this file.
*/
private PrintWriter fOut;
/*
* String buffered writer used when an external output stream is not
* specified. Is null if going to a stream.
*/
private StringWriter fStringOut;
/**
* Should warnings be printed?
*/
private boolean fPrintWarnings = true;
/*
* This controls the printing of stack traces that are normally supressed.
*/
private boolean fPrintDebug = false;
/*
* Count of errors that have occured.
*/
private int fErrorCnt = 0;
/*
* Count of warning that have occured.
*/
private int fWarningCnt = 0;
/**
* Construct a new error report object.
*
* @param output Writer to recieve error output.
*/
public ErrorReporter(PrintWriter output) {
fOut = output;
}
/**
* Construct a new error report object.
*
* @param output PrintStream to recieve error output.
*/
public ErrorReporter(PrintStream output) {
this(new PrintWriter(output, true));
}
/**
* Construct a new error report object with output
* saved in a string buffer.
*/
public ErrorReporter() {
fStringOut = new StringWriter();
fOut = new PrintWriter(fStringOut, true);
}
/**
* Get the value of the printWarnings flag.
*/
public boolean getPrintWarnings() {
return fPrintWarnings;
}
/**
* Set the value of the printWarnings flag.
*/
public void setPrintWarnings(boolean value) {
fPrintWarnings = value;
}
/**
* Get the value of the printDebug flag.
*/
public boolean getPrintDebug() {
return fPrintDebug;
}
/**
* Set the value of the printDebug flag. This controls the printing
* of stack traces that are normally supressed.
*/
public void setPrintDebug(boolean value) {
fPrintDebug = value;
}
/**
* Get the count of errors that have occured.
*/
public int getErrorCnt() {
return fErrorCnt;
}
/**
* Get the count of warnings that have occured.
*/
public int getWarningCnt() {
return fWarningCnt;
}
/**
* Get the buffer containing the output if internal buffering
* has been used.
*
* @return buffer containing output or null if output was written to
* an external stream.
*/
public String getOutput() {
if (fStringOut == null) {
return null;
} else {
return fStringOut.toString();
}
}
/**
* Generate a message with file name and line number.
* This is in a format parsable by emacs and other
* editors.
*/
private void printFileLineMsg(String msg,
boolean isError,
String fileName,
int lineNum) {
fOut.println(fileName + ":" + lineNum + ": "
+ (isError ? "Error: " : "Warning: ")
+ msg);
}
/**
* Report an error.
*/
public void error(String msg) {
fOut.print("Error: ");
fOut.println(msg);
fErrorCnt++;
}
/**
* Report an error with file name and line number.
*/
public void error(String msg,
String fileName,
int lineNum) {
printFileLineMsg(msg, true, fileName, lineNum);
fErrorCnt++;
}
/**
* Report an error with file name and line number.
*/
public void error(String msg,
Locator locator) {
printFileLineMsg(msg, true, locator.getSystemId(),
locator.getLineNumber());
fErrorCnt++;
}
/**
* Report an error from an exception
*/
public void error(String msg,
Throwable except) {
fOut.println("Error: " + msg + ": " + except.toString());
except.printStackTrace(fOut);
fErrorCnt++;
}
/**
* Report a warning.
*/
public void warning(String msg) {
if (fPrintWarnings) {
fOut.print("Warning: ");
fOut.println(msg);
}
fWarningCnt++;
}
/**
* Report a warning with file name and line number.
*/
public void warning(String msg,
String fileName,
int lineNum) {
if (fPrintWarnings) {
printFileLineMsg(msg, false, fileName, lineNum);
}
fWarningCnt++;
}
/**
* Report a warning with file name and line number.
*/
public void warning(String msg,
Locator locator) {
if (fPrintWarnings) {
printFileLineMsg(msg, false, locator.getSystemId(),
locator.getLineNumber());
}
fWarningCnt++;
}
/**
* Print a SAX parser message in a consistent style, consistent
* with emacs compile mode (same as grep, cc, javac, etc).
*/
private void printSAXMsg(boolean isError,
SAXParseException exception) {
printFileLineMsg(exception.getMessage(), isError,
exception.getSystemId(),
exception.getLineNumber());
if (fPrintDebug) {
fOut.println(exception.toString());
Exception cause = exception.getException();
if (cause != null) {
fOut.println("Cause: " + cause.getMessage());
cause.printStackTrace(fOut);
}
}
}
/**
* Receive notification of a SAX warning.
*/
public void warning(SAXParseException exception) throws SAXException {
if (fPrintWarnings) {
printSAXMsg(false, exception);
}
fWarningCnt++;
}
/**
* Receive notification of a SAX recoverable error.
*/
public void error(SAXParseException exception) throws SAXException {
if (fPrintWarnings) {
printSAXMsg(true, exception);
}
fErrorCnt++;
}
/**
* Receive notification of a SAX non-recoverable error.
*/
public void fatalError(SAXParseException exception)
throws SAXException {
printSAXMsg(true, exception);
fErrorCnt++;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy