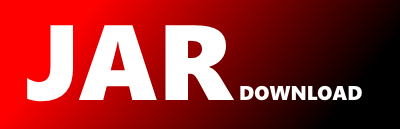
org.enhydra.xml.io.HTMLElements Maven / Gradle / Ivy
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: HTMLElements.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.io;
import java.util.HashMap;
import org.w3c.dom.Element;
/**
* Information about HTML elements and attributes for use HTML formatter.
*/
final public class HTMLElements {
/**
* Format element as a block.
*/
public static final Integer BLOCK_FORMATTING = new Integer(1);
/**
* Format element as a header.
*/
public static final Integer HEADER_FORMATTING = new Integer(2);
/**
* Format element as a inline.
*/
public static final Integer INLINE_FORMATTING = new Integer(3);
/**
* Block-formatted elements.
*/
private static final String[] BLOCK_FORMATTED_ELEMENTS = {
"HTML", "HEAD", "BODY", "SCRIPT", "P", "UL", "OL", "DL", "DIR",
"MENU", "PRE", "LISTING", "XMP", "PLAINTEXT", "ADDRESS", "BLOCKQUOTE",
"FORM", "ISINDEX", "FIELDSET", "TABLE", "HR", "DIV", "NOSAVE", "LAYER",
"NOLAYER", "ALIGN", "CENTER", "INS", "DEL", "NOFRAMES", "NOSCRIPT",
"AREA"
};
/**
* Header-formatted elements.
*/
private static final String[] HEADER_FORMATTED_ELEMENTS = {
"TITLE", "H1", "H2", "H3", "H4", "H5", "H6"
};
/**
* Tag name to formatting. All tags not found are assumed
* to be inline.
*/
private static final HashMap fFormattingMap = new HashMap();
/**
* List of attributes that do not have values. If the attribute
* exists and is empty, we output it without a value.
* Note: HTML attribute names are normalized to lowercase in the DOM.
*/
private static final String[] NO_VALUE_ATTRS = {
"checked", "compact", "declare", "defer", "disabled", "ismap",
"nohref", "noresize", "noshade", "nowrap", "readonly", "selected"
};
private static final HashMap fNoValueAttrs = new HashMap();
/**
* List and table of element classes where closing elements are forbidden
* (or in the case, just not desired).
*/
private static final String[] NO_CLOSE_TAGS = {
"AREA", "BASE", "BASEFONT", "BR", "COL", "FRAME", "HR", "IMG",
"INPUT", "ISINDEX", "LINK", "META", "PARAM", "P"
};
private static final HashMap fNoCloseTags = new HashMap();
/**
* Static constructor.
*/
static {
for (int idx = 0; idx < NO_CLOSE_TAGS.length; idx++) {
fNoCloseTags.put(NO_CLOSE_TAGS[idx], NO_CLOSE_TAGS[idx]);
}
for (int idx = 0; idx < NO_VALUE_ATTRS.length; idx++) {
fNoValueAttrs.put(NO_VALUE_ATTRS[idx], NO_VALUE_ATTRS[idx]);
}
for (int idx = 0; idx < BLOCK_FORMATTED_ELEMENTS.length; idx++) {
fFormattingMap.put(BLOCK_FORMATTED_ELEMENTS[idx],
BLOCK_FORMATTING);
}
for (int idx = 0; idx < HEADER_FORMATTED_ELEMENTS.length; idx++) {
fFormattingMap.put(HEADER_FORMATTED_ELEMENTS[idx],
HEADER_FORMATTING);
}
}
/**
* Prevent instanciation.
*/
private HTMLElements() {
}
/**
* Check if an attribute does not have a value (is boolean).
* @param attrName Nme of attribute, must be lower-case.
*/
public static boolean isBooleanAttr(String attrName) {
return fNoValueAttrs.containsKey(attrName);
}
/**
* Determine the formatting to use for an element. Unknown
* elements are returned as INLINE_FORMATTING.
* @param tagName Name of element, must be upper-case.
* @return One of the *_FORMATTING constants; references can
* be compared.
*/
public Integer getElementFormatting(String tagName) {
Integer formatting = (Integer)fFormattingMap.get(tagName);
if (formatting == null) {
return INLINE_FORMATTING;
} else {
return formatting;
}
}
/**
* Check if an element is may have a close tag.
* @param tagName Name of element, must be upper-case.
*/
public static boolean hasCloseTag(String tagName) {
return !fNoCloseTags.containsKey(tagName);
}
/**
* Test if an Element is a script or style element, which have special
* content handling.
*/
public static boolean isScriptStyle(Element element) {
String tagName = element.getTagName();
return (tagName.equals("SCRIPT") || tagName.equals("STYLE"));
}
// FIXME: Add factory methods for all HTML elements.
}