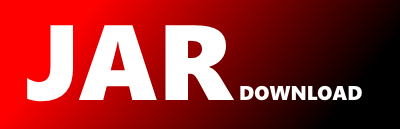
org.enhydra.xml.io.InputSourceOps Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: InputSourceOps.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.io;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import org.xml.sax.InputSource;
/**
* Various operations on InputSources.
*/
public final class InputSourceOps {
/**
* XML document header prefix.
*/
private static String XML_DOCUMENT_HEADER_PREFIX = "= 2) && (colonIdx < systemId.indexOf('/')));
}
/**
* Open a bytestream given a system id.
*/
public static InputStream openSystemId(String systemId) throws IOException {
if (systemId == null) {
throw new IllegalArgumentException("no open stream or system id specified in InputSource");
}
if (hasScheme(systemId)) {
return new URL(systemId).openStream();
} else {
return new FileInputStream(systemId);
}
}
/**
* Open a character input stream for an input source if one is not
* already open.
*
* @param inputSource Specification of the document to open.
* @return A pointer to the character stream.
*/
public static Reader open(InputSource inputSource) throws IOException {
if (inputSource.getCharacterStream() != null) {
return inputSource.getCharacterStream();
}
InputStream in = inputSource.getByteStream();
if (in == null) {
in = openSystemId(inputSource.getSystemId());
}
String encoding = inputSource.getEncoding();
if (encoding != null) {
return new BufferedReader(new InputStreamReader(in, encoding));
} else {
return new BufferedReader(new InputStreamReader(in));
}
}
/**
* Determine if an input source has an open byte or character stream.
*/
public static boolean isOpen(InputSource input) {
return (input.getCharacterStream() != null)
|| (input.getByteStream() != null);
}
/**
* Close an InputSource, if open.
*/
public static void close(InputSource input) throws IOException {
if (input.getCharacterStream() != null) {
input.getCharacterStream().close();
}
if (input.getByteStream() != null) {
input.getByteStream().close();
}
}
/**
* Close a byte stream returned by open()
, only if it was
* actually opened by open.
*
* @param inputSource Specification of the document that was opened.
* @param reader The character stream returned by open.
*/
public static void closeIfOpened(InputSource inputSource,
Reader reader) throws IOException {
if ((inputSource.getCharacterStream() == null)
&& (inputSource.getByteStream() == null)) {
reader.close();
}
}
/**
* Get a description of an input source.
*/
public static String getName(InputSource input) {
if (input == null) {
return "";
}
String name = null;
if (input.getSystemId() != null) {
name = input.getSystemId();
} else if (input.getPublicId() != null) {
name = input.getPublicId();
}
// If byte or character stream is open, indicate that with the name.
if (input.getByteStream() != null) {
if (name == null) {
return "";
} else {
return name +"";
}
}
if (input.getCharacterStream() != null) {
if (name == null) {
return "";
} else {
return name +"";
}
}
if (name != null) {
return name;
} else {
return "";
}
}
/**
* Determine an document reader is attached to an XML document
* by checking the first few bytes. Reader must be positioned
* at the start.
*
* @param reader Character stream.
*/
public static boolean isXMLDocument(Reader reader) throws IOException {
char[] buf = new char[XML_DOCUMENT_HEADER_PREFIX.length()];
reader.mark(XML_DOCUMENT_HEADER_PREFIX.length()+1);
if (reader.read(buf) != buf.length) {
return false;
}
return String.copyValueOf(buf).equals(XML_DOCUMENT_HEADER_PREFIX);
}
/**
* Determine an input source points to an XML document.
*
* @param inputSource Specification of the document to examine.
*/
public static boolean isXMLDocument(InputSource inputSource) throws IOException {
Reader reader = open(inputSource);
try {
return isXMLDocument(reader);
} finally {
closeIfOpened(inputSource, reader);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy