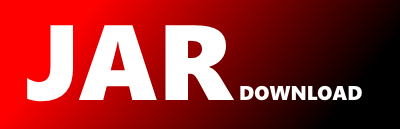
org.enhydra.xml.io.OutputOptions Maven / Gradle / Ivy
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: OutputOptions.java,v 1.10 2005/07/21 06:18:14 jkjome Exp $
*/
package org.enhydra.xml.io;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import org.enhydra.xml.xmlc.codegen.JavaCode;
import org.enhydra.xml.xmlc.codegen.JavaLang;
/**
* Object that specifies how a HTML or XML file will be formatted.
*
* Use of certain options may be required when using certain document types
* in order to provide compatiblity for buggy or less capable browsers. Make
* sure to read the option descriptions and do your own testing to make sure
* these options are really necessary for use in your application...
*
* - HTML
* - {@link #setUseAposEntity oo.setUseAposEntity(false)}
* - {@link #setOmitAttributeCharEntityRefs oo.setOmitAttributeCharEntityRefs(true)}
* - {@link #setOmitDocType oo.setOmitDocType(false)} (html dom doesn't preserve doctype, so use this and two options below...)
* - {@link #setPublicId oo.setPublicId("-//W3C//DTD HTML 4.01//EN")}
* - {@link #setSystemId oo.setSystemId("http://www.w3.org/TR/html401/strict.dtd")}
* - XHTML
* - {@link #setEnableXHTMLCompatibility oo.setEnableXHTMLCompatibility(true)}
* - {@link #setUseAposEntity oo.setUseAposEntity(false)}
* - XML
* - Overriding the defaults of most of these options is not recommended for pure XML and may cause output that doesn't strictly follow the XML spec and/or, in the worst case, invalid XML.
*
*
*
* Note: the pretty-printing options are not yet implemented by the
* formatters.
*/
public final class OutputOptions {
// N.B. Be sure to modify createCodeGenerator and toString if any fields
// are added or default values changed.
/**
* Desired output format enumerated type.
*/
public static class Format {
/** Name of format */
private final String fName;
/** Constructor (package only) */
Format(String name) {
fName = name;
}
/** Get string name of format */
public String toString() {
return fName;
}
}
/**
* Constant indicating format should be determined automatically
* from examining the document object.
*/
public static final Format FORMAT_AUTO = new Format("FORMAT_AUTO");
/**
* Constant indicating HTML format.
*/
public static final Format FORMAT_HTML = new Format("FORMAT_HTML");
/**
* Constant indicating XML format.
*/
public static final Format FORMAT_XML = new Format("FORMAT_XML");
/**
* The output file format to use.
*/
private Format fFormat = FORMAT_AUTO;
/**
* The encoding to use.
*/
private String fEncoding = null;
/**
* Whether the document be pretty-printed.
*/
private boolean fPrettyPrinting = false;
/**
* Number of characters to indent if using pretty printing.
*/
private int fIndentSize = 4;
/**
* Whether space is preserved where not otherwise specified by
* the document.
*/
private boolean fPreserveSpace = true;
/**
* Whether to omit the XML header (for XML documents).
*/
private boolean fOmitXMLHeader = false;
/**
* Whether to omit the DOCTYPE for either XML or HTML.
*/
private boolean fOmitDocType = false;
/**
* Whether to omit the encoding in the XML header.
*/
private boolean fOmitEncoding = false;
/**
* Whether id's on HTML <span> tags are removed
*/
private boolean fDropHtmlSpanIds = false;
/**
* Whether to omit character entity references in attribute values.
*/
private boolean fOmitAttributeCharEntityRefs = false;
/**
* The Rewriter for URL attribute values.
*/
private URLRewriter fURLRewriter;
/**
* Set of attribute names to perform URL rewriting upon. Overrides defaults set per XMLCDomFactory.
*/
private Set fURLRewriteAttributes;
/**
* The public id to use in the DOCTYPE. Overrides the DocumentType.
*/
private String fPublicId;
/**
* The system id to use in the DOCTYPE. Overrides the DocumentType.
*/
private String fSystemId;
/**
* Optional MIME type for an output routine to use.
*/
private String fMIMEType;
/**
* Whether to use internal XHTML compatibility workarounds.
*
* @see #setEnableXHTMLCompatibility()
*/
private boolean fEnableXHTMLCompatibility = false;
/**
* Whether to use ' to escape single quotes
*/
private boolean fUseAposEntity = true;
/**
* Whether this object is read-only.
*/
private boolean fReadOnly = false;
/**
* Whether to use named character entities for all entities found in HTML
* 4.0.
*/
private boolean fUseHTML4Entities = false;
/**
* Construct with default values.
*/
public OutputOptions() {
}
/**
* Copy constructor. The read-only property is not copied, the
* resulting object may be modified.
*/
public OutputOptions(OutputOptions src) {
fFormat = src.fFormat;
fEncoding = src.fEncoding;
fPrettyPrinting = src.fPrettyPrinting;
fIndentSize = src.fIndentSize;
fPreserveSpace = src.fPreserveSpace;
fOmitXMLHeader = src.fOmitXMLHeader;
fOmitDocType = src.fOmitDocType;
fOmitEncoding = src.fOmitEncoding;
fDropHtmlSpanIds = src.fDropHtmlSpanIds;
fOmitAttributeCharEntityRefs = src.fOmitAttributeCharEntityRefs;
fURLRewriter = src.fURLRewriter;
fURLRewriteAttributes = src.fURLRewriteAttributes;
fPublicId = src.fPublicId;
fSystemId = src.fSystemId;
fMIMEType = src.fMIMEType;
fUseHTML4Entities = src.fUseHTML4Entities;
fEnableXHTMLCompatibility = src.fEnableXHTMLCompatibility;
fUseAposEntity = src.fUseAposEntity;
}
/**
* Validate that the object is writable.
*/
private void readOnlyCheck() {
if (fReadOnly) {
throw new XMLIOError(OutputOptions.class.getName() + " object is marked as read-only");
}
}
/**
* Mark the object as read-only. Once made read-only, it may never be modified.
* If a modification is required, create a new object using the copy-constructor.
*
* @see #OutputOptions(OutputOptions)
*/
public void markReadOnly() {
fReadOnly = true;
}
/**
* Set the output format for the file. Specifying an incorrect format
* will result in an invalid document. Default is FORMAT_AUTO,
* which determines the format from the DOM.
*
* @param format one of FORMAT_AUTO, FORMAT_HTML, FORMAT_XML
*/
public void setFormat(Format format) {
readOnlyCheck();
fFormat = format;
}
/**
* Get the output format for the file.
*
* @return the output format
*/
public Format getFormat() {
return fFormat;
}
/**
* Get the encoding.
*
* @return The encoding or null if not specified.
*/
public String getEncoding() {
return fEncoding;
}
/**
* Set the encoding.
*
* @param encoding The new encoding, or null to clear.
*/
public void setEncoding(String encoding) {
readOnlyCheck();
fEncoding = encoding;
}
/**
* Get the MIME encoding.
*
* @return The MIME-preferred name for the encoding, null if
* no encoding is specified.
*/
public String getMIMEEncoding() {
if (fEncoding != null) {
String mimeEncoding = Encodings.getEncodings().getMIMEPreferred(fEncoding);
if (mimeEncoding != null) {
return mimeEncoding;
}
}
return fEncoding;
}
/**
* Get use-apos-entity flag
*
* @return true if enabled, false if disabled. The default is enabled.
*/
public boolean getUseAposEntity() {
return fUseAposEntity;
}
/**
* Enable or disable the use of ' for escaping single quotes.
* ' was added in to the XML spec and doesn't exist in the
* HTML spec. As such, some browsers (some versions of IE) handle this
* poorly in attributes (think JavaScript). It is recommended that you
* disable the use of ' entity if you run into problems. This
* option will, eventually, be unnecessary once more browsers provide
* support for the ' entity.
*
* @param enable true to enable, false to disable.
*/
public void setUseAposEntity(boolean enable) {
readOnlyCheck();
fUseAposEntity = enable;
}
/**
* Get enable-xhtml-compatibility flag.
*
* @since 2.2
* @return true if enabled, false if disabled. The default is disabled.
*/
public boolean getEnableXHTMLCompatibility() {
return fEnableXHTMLCompatibility;
}
/**
* Enable or disable XMLC XHTML compatibility workarounds
*
* Many browsers still in heavy use
* don't fully support XHTML. In order to make the transition to XHTML,
* XMLC must allow for reasonable workarounds to known compatibility issues
* in these less capable browsers. Setting this flag to 'true' tells XMLC
* and, specifically, the XMLFormater to do anything it needs to maintain
* compatibility with older browsers. Currently, the following XHTML
* compatibility issues are accounted for:
*
* - {@link http://www.w3.org/TR/xhtml1/#C_2}
* - {@link http://www.w3.org/TR/xhtml1/#C_3}
.
*
* This allows browsers, using an HTML parser, to render XHTML properly.
* It also allows IE 5.5 and IE6 to evaluate javascript properly, since
* said browsers fail to understand the minimized <script ... />.
*
* Note that this method name is intentionally generic to allow for the
* application of other arbitrary workarounds without requiring new methods
* to be added to OutputOptions.
*
* @since 2.2
* @param enable true to enable, false to disable
*/
public void setEnableXHTMLCompatibility(boolean enable) {
readOnlyCheck();
fEnableXHTMLCompatibility = enable;
}
/**
* Get pretty-printing flag.
*
* @return true if enabled, false if disabled. The default is disabled.
*/
public boolean getPrettyPrinting() {
return fPrettyPrinting;
}
/**
* Enable or disable pretty-printing.
*
* @param enable true to enable, false to disable
*/
public void setPrettyPrinting(boolean enable) {
readOnlyCheck();
fPrettyPrinting = enable;
}
/**
* Get indentation size.
*
* @return Number of characters to indent at each level. The default is 4.
*/
public int getIndentSize() {
return fIndentSize;
}
/**
* Set indentation size. Only used if pretty printing is enabled.
*
* @param size Number of characters to indent at each level.
*/
public void setIndentSize(int size) {
readOnlyCheck();
fIndentSize = size;
if (fIndentSize < 0) {
fIndentSize = 0;
}
}
/**
* Get the default space-preservation flag.
*
* @return true if preserving space where not otherwise specified by
* the document, false otherwise. The default is true.
*/
public boolean getPreserveSpace() {
return fPreserveSpace;
}
/**
* Set the default space-preservation flag.
*
* @param perserve true if preserving space where not otherwise specified
* by the document
*/
public void setPreserveSpace(boolean preserve) {
readOnlyCheck();
fPreserveSpace = preserve;
}
/**
* Get flag indicating if the XML header should be omitted.
*
* @return true if omitted, false if admitted. The default is admitted.
*/
public boolean getOmitXMLHeader() {
return fOmitXMLHeader;
}
/**
* Set flag indicating if the XML header should be omitted.
*
* @param omit true to omit, false to admit
*/
public void setOmitXMLHeader(boolean omit) {
readOnlyCheck();
fOmitXMLHeader = omit;
}
/**
* Get flag indicating if the DOCTYPE should be omitted.
*
* @return true if omitted, false if admitted. The default is admitted.
*/
public boolean getOmitDocType() {
return fOmitDocType;
}
/**
* Set flag indicating if the DOCTYPE should be omitted.
*
* @param omit true to omit, false to admit
*/
public void setOmitDocType(boolean omit) {
readOnlyCheck();
fOmitDocType = omit;
}
/**
* Get flag indicating if encoding should be omitted from the XML header.
*
* @return true if omitted, false if admitted. The default is admitted.
*/
public boolean getOmitEncoding() {
return fOmitEncoding;
}
/**
* Set flag indicating if encoding should be omitted from the XML header.
* This is provided as a hack for WML. Several devices need ASCII
* encoding but can't handle the header.
*
* @param omit true to omit, false to admit
*/
public void setOmitEncoding(boolean omit) {
readOnlyCheck();
fOmitEncoding = omit;
}
/**
* Get the drop HTML SPAN element ids flag.
*
* @return true if dropped, false if kept. The default is kept.
*/
public boolean getDropHtmlSpanIds() {
return fDropHtmlSpanIds;
}
/**
* Set the drop HTML <span> element id's flag.
* MS Internet Explorer 4.0 gets very confused about keep-alive
* connections if HTML <span> tags have "id" attributes.
* Until we find another workaround, this removes "id"'s from
* <span> tags.
* Unless you have to support IE4 users and require keep-alive
* connections to your application, this option is not recommended
*
* @param drop true to drop, false to keep
*/
public void setDropHtmlSpanIds(boolean drop) {
readOnlyCheck();
fDropHtmlSpanIds = drop;
}
/**
* Get value of flag that enables or disables the use of character entity
* references in attribute values.
*
* @return true if omitted, false if admitted. The default is admitted.
*/
public boolean getOmitAttributeCharEntityRefs() {
return fOmitAttributeCharEntityRefs;
}
/**
* Set value of flag that enables or disables the use of character entity
* references in attribute values.
* By default, all standard character
* entity references are used in attribute values. While this is legal in
* HTML and XML, some HTML clients may not handle this well
* (for instance, one of the major browsers didn't correctly expand the
* entity references in PARAM values passed to applets).
* If this flag is set, then standard character entity
* references (such as &) will not be substituted. Numeric
* character entity references will still be substituted for quotes and
* for characters that can't be represented in the encoding.
* This option is not recommended for XML and should be avoided in any
* case unless this issue becomes a problem in your application.
*
* @param omit true to omit, false to admit
* @see #getOmitAttributeCharEntityRefs()
*/
public void setOmitAttributeCharEntityRefs(boolean omit) {
fOmitAttributeCharEntityRefs = omit;
}
/**
* Set the URLRewriter that all URL attributes will be passed through.
* Documents must implement DocumentInfo for the URL rewriter to
* work.
*
* Note: URL rewriting curret only works when the XMLC document
* object is passed to the formatter. Passing the contained document or
* any other node results in no URL rewriting.
*
* @param urlRewriter The URLRewriter object, or null to disassociate
* any URL rewriter.
* @see DocumentInfo
*/
public void setURLRewriter(URLRewriter urlRewriter) {
readOnlyCheck();
fURLRewriter = urlRewriter;
}
/**
* Get the URLRewriter.
*
* @return The URLRewriter object.
* @see DocumentInfo
*/
public URLRewriter getURLRewriter() {
return fURLRewriter;
}
/**
* Get the URL attribute names to apply session rewriting. If null, defaults
* defined per respective XMLCDomFactory are used.
*
* @return the set of attribute name strings
*/
public Set getURLRewriteAttributes() {
return fURLRewriteAttributes;
}
/**
* Set the attribute names to apply URLRewriting to (assuming
* URLRewriting is being used instead of session cookies). If set, this
* overrides the defaults provided by each respective XMLCDomFactory
* implementation.
*
* @param attributes a set of attribute name strings to rewrite, null to use
* defaults defined in each respective XMLCDomFactory
* implementation.
*/
public void setURLRewriteAttributes(Set attributes) {
readOnlyCheck();
fURLRewriteAttributes = attributes;
}
/**
* @param attributes
* @see #setURLRewriteAttributes(Set)
*/
public void setURLRewriteAttributes(String[] attributes) {
if (attributes == null) {
setURLRewriteAttributes((Set) null);
return;
}
setURLRewriteAttributes(new HashSet(Arrays.asList(attributes)));
}
/**
* Get the public id to be used in the DOCUMENT.
*
* @return the public id
*/
public String getPublicId() {
return fPublicId;
}
/**
* Set the public id to use in the DOCUMENT. This overrides the
* default value determined from the DocumentType.
*
* @return the public id
*/
public void setPublicId(String publicId) {
readOnlyCheck();
fPublicId = publicId;
}
/**
* Get the system id to be used in the DOCUMENT.
*
* @return the system id
*/
public String getSystemId() {
return fSystemId;
}
/**
* Set the system id to use in the DOCUMENT. This overrides the
* default value determined from the DocumentType.
*
* @return the system id
*/
public void setSystemId(String systemId) {
readOnlyCheck();
fSystemId = systemId;
}
/**
* Get the MIME for an output routine to use.
*
* @return The overriding MIME type, null if one was not specified.
*/
public String getMIMEType() {
return fMIMEType;
}
/**
* Set the MIME for an output routine to use. This is stored in this
* object for use by output routines, DOMFormatters don't actually use it.
* It is normally used to override the default MIME type that would be
* stored in the XMLObject.
*
* @param mimeType the mime-type to use
*/
public void setMIMEType(String mimeType) {
readOnlyCheck();
fMIMEType = mimeType;
}
/**
* Generate code to set a String property.
*/
private void genSet(String varName,
JavaCode code,
String setMethod,
String value) {
code.addln(varName + "." + setMethod + "("
+ JavaLang.createStringConst(value) + ");");
}
/**
* Generate code to set a boolean property.
*/
private void genSet(String varName,
JavaCode code,
String setMethod,
boolean value) {
code.addln(varName + "." + setMethod + "("
+ (value ? "true" : "false") + ");");
}
/**
* Generate code to set an int property.
*/
private void genSet(String varName,
JavaCode code,
String setMethod,
int value) {
code.addln(varName + "." + setMethod + "("
+ Integer.toString(value) + ");");
}
/**
* Generate code to create an object with the same attributes as this
* object.
*
* The following attributes are not generated in the new code:
*
* - readOnly - Set to the value of the makeReadOnly parameter.
*
- urlRewriter
*
*
* @param varName Variable or field name of variable to store
* the object in. It must already be declared.
* @param makeReadOnly Should the created object be made read-only?
* @param code Add generated code to this object.
*/
public void createCodeGenerator(String varName,
boolean makeReadOnly,
JavaCode code) {
//FIXME: Change to only generate code if not default
code.addln(varName + " = new " + OutputOptions.class.getName() + "();");
code.addln(varName + ".setFormat("
+ OutputOptions.class.getName()
+ "." + fFormat.toString() + ");");
// Java encoding set by setting XML encoding
genSet(varName, code, "setEncoding", fEncoding);
genSet(varName, code, "setPrettyPrinting", fPrettyPrinting);
genSet(varName, code, "setEnableXHTMLCompatibility", fEnableXHTMLCompatibility);
genSet(varName, code, "setUseAposEntity", fUseAposEntity);
genSet(varName, code, "setIndentSize", fIndentSize);
genSet(varName, code, "setPreserveSpace", fPreserveSpace);
genSet(varName, code, "setOmitXMLHeader", fOmitXMLHeader);
genSet(varName, code, "setOmitDocType", fOmitDocType);
genSet(varName, code, "setOmitEncoding", fOmitEncoding);
genSet(varName, code, "setDropHtmlSpanIds", fDropHtmlSpanIds);
genSet(varName, code, "setOmitAttributeCharEntityRefs", fOmitAttributeCharEntityRefs);
genSet(varName, code, "setPublicId", fPublicId);
genSet(varName, code, "setSystemId", fSystemId);
genSet(varName, code, "setMIMEType", fMIMEType);
if (makeReadOnly) {
code.addln(varName + ".markReadOnly();");
}
}
/**
* Convert to a string for debugging.
*/
public String toString() {
return "readOnly=" + fReadOnly
+ ", format=" + fFormat
+ ", encoding=" + fEncoding
+ ", prettyPrinting=" + fPrettyPrinting
+ ", useAposEntity=" + fUseAposEntity
+ ", enableXHTMLCompatibility=" + fEnableXHTMLCompatibility
+ ", indentSize=" + fIndentSize
+ ", preserveSpace=" + fPreserveSpace
+ ", omitXMLHeader=" + fOmitXMLHeader
+ ", omitDocType=" + fOmitDocType
+ ", omitEncoding=" + fOmitEncoding
+ ", dropHtmlSpanIds=" + fDropHtmlSpanIds
+ ", omitAttributeCharEntityRefs=" + fOmitAttributeCharEntityRefs
+ ", urlRewriter=" + fURLRewriter
+ ", publicId=" + fPublicId
+ ", systemId=" + fSystemId
+ ", mimeType=" + fMIMEType;
}
/**
* Get the encoding.
*
* @deprecated Use getEncoding() or getMIMEEncoding().
* @see #getEncoding
* @see #getMIMEEncoding
*/
public String getJavaEncoding() {
return getEncoding();
}
/**
* Set the encoding.
*
* @deprecated Use setEncoding().
* @see #setEncoding
*/
public void setJavaEncoding(String newJavaEncoding) {
setEncoding(newJavaEncoding);
}
/**
* Get the MIME-preferred encoding.
*
* @deprecated Use getMIMEEncoding().
* @see #getMIMEEncoding
*/
public String getXmlEncoding() {
return getMIMEEncoding();
}
/**
* Set the encoding.
*
* @deprecated Use setEncoding().
*/
public void setXmlEncoding(String newXmlEncoding) {
setEncoding(newXmlEncoding);
}
/**
* Get the flag telling whether to use named entities from HTML 4.0 or
* not.
*
* @return true if enabled, false if disabled. The default is disabled.
*/
public boolean getUseHTML4Entities() {
return fUseHTML4Entities;
}
/**
* Set the flag telling whether to use named entities from HTML 4.0 or
* not.
*
* @param useHTML4Entities true to enable, false to disable
*/
public void setUseHTML4Entities(boolean useHTML4Entities) {
fUseHTML4Entities = useHTML4Entities;
}
}