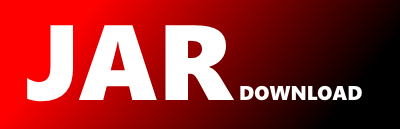
org.enhydra.xml.lazydom.LazyAttrNoNS Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: LazyAttrNoNS.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.lazydom;
import org.enhydra.apache.xerces.dom.AttrImpl;
import org.enhydra.apache.xerces.dom.NodeImpl;
import org.w3c.dom.DOMException;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
* Implementation of the DOM Attr that supports lazy instantiation of
* a template DOM. This version does not support namespaaces. It is
* used by HTML, where non-standard attributes containing `:' are
* occasionally invented. If this was derived from AttrNSImpl, it
* would do validation on the name and generate an error.
*/
public class LazyAttrNoNS extends AttrImpl implements LazyAttr {
/**
* Constructor with no namespace.
* @param ownerDoc The document that owns this node.
* @param template If not-null, get the parameters from this template.
* @param qualifiedName The attribute name.
* Will be ignored if template is not-null.
*/
protected LazyAttrNoNS(LazyDocument ownerDoc,
LazyAttrNoNS template,
String qualifiedName) {
super(ownerDoc,
(template != null) ? template.getNodeName() : qualifiedName);
if (template != null) {
fTemplateNode = template;
fNodeId = template.getNodeId();
} else {
// Not created from a template, mark all as expanded.
fParentExpanded = true;
fChildrenExpanded = true;
}
}
//-------------------------------------------------------------------------
// LazyAttrNoNS specific
//-------------------------------------------------------------------------
/**
* Template for this Attr.
*/
private LazyAttrNoNS fTemplateNode = null;
/**
* Get the template for this node.
* @see LazyNode#getTemplateNode
*/
public LazyAttrNoNS getTemplateAttr() {
return fTemplateNode;
}
/**
* @see Node#cloneNode
*/
public Node cloneNode(boolean deep) {
if (deep) {
// If children are copied, we must expand now.
if (!fChildrenExpanded) {
expandChildren();
}
}
// This does a clone(), must clean up all fields.
LazyAttrNoNS newAttr = (LazyAttrNoNS)super.cloneNode(deep);
newAttr.fNodeId = NULL_NODE_ID;
newAttr.fParentExpanded = true;
newAttr.fChildrenExpanded = true;
return newAttr;
}
/**
* @see org.w3c.dom.Attr#getValue
*/
public String getValue() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getValue();
}
/**
* @see org.w3c.dom.Attr#setValue
*/
public void setValue(String value) throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
super.setValue(value);
}
/**
* @see org.w3c.dom.Node#getNodeValue
*/
public String getNodeValue() throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getNodeValue();
}
/**
* @see org.w3c.dom.Node#setNodeValue
*/
public void setNodeValue(String nodeValue) throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
super.setNodeValue(nodeValue);
}
//-------------------------------------------------------------------------
// LazyNode support
//-------------------------------------------------------------------------
/*
* Node id for this element.
*/
private int fNodeId = NULL_NODE_ID;
/**
* Is this a template node?
*/
private boolean fIsTemplateNode;
/*
* @see LazyNode#makeTemplateNode
*/
public void makeTemplateNode(int nodeId) {
fNodeId = nodeId;
fIsTemplateNode = true;
}
/**
* @see LazyNode#getNodeId
*/
public int getNodeId() {
return fNodeId;
}
/**
* @see LazyNode#isTemplateNode
*/
public boolean isTemplateNode() {
return fIsTemplateNode;
}
/**
* @see LazyNode#getTemplateNode
*/
public LazyNode getTemplateNode() {
return fTemplateNode;
}
/**
* @see LazyNode#templateClone
*/
public LazyNode templateClone(Document ownerDocument) {
return new LazyAttrNoNS((LazyDocument)ownerDocument, this, null);
}
// NB: Attr has specicia functionality for setNodeValue.
//-------------------------------------------------------------------------
// LazyParent support
//-------------------------------------------------------------------------
/**
* Parent and children expanded flags.
*/
private boolean fParentExpanded = false;
private boolean fChildrenExpanded = false;
/**
* @see LazyParent#isParentExpanded()
*/
public boolean isParentExpanded() {
return fParentExpanded;
}
/**
* @see LazyParent#setParentExpanded
*/
public void setParentExpanded() {
fParentExpanded = true;
}
/**
* @see LazyParent#setParentWhileExpanding
*/
public void setParentWhileExpanding(Node parent) {
ownerNode = (NodeImpl)parent;
flags |= OWNED;
fParentExpanded = true;
}
/**
* @see LazyParent#areChildrenExpanded()
*/
public boolean areChildrenExpanded() {
return fChildrenExpanded;
}
/**
* @see LazyParent#setChildrenExpanded
*/
public void setChildrenExpanded() {
fChildrenExpanded = true;
}
/**
* @see LazyParent#appendChildWhileExpanding
*/
public void appendChildWhileExpanding(Node child) {
super.insertBefore(child, null);
}
/**
* Expand the parent of this element, if it is not already expanded.
*/
private void expandParent() {
((LazyDocument)getOwnerDocument()).doExpandParent(this);
}
/**
* Expand the children of this element, if they are not already expanded.
*/
private void expandChildren() {
((LazyDocument)getOwnerDocument()).doExpandChildren(this);
}
/**
* @see org.w3c.dom.Node#getParentNode
*/
public Node getParentNode() {
if (!fParentExpanded) {
expandParent();
}
return super.getParentNode();
}
/**
* @see org.w3c.dom.Node#getChildNodes
*/
public NodeList getChildNodes() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getChildNodes();
}
/**
* @see org.w3c.dom.Node#getFirstChild
*/
public Node getFirstChild() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getFirstChild();
}
/**
* @see org.w3c.dom.Node#getLastChild
*/
public Node getLastChild() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getLastChild();
}
/**
* @see org.w3c.dom.Node#getPreviousSibling
*/
public Node getPreviousSibling() {
if (!fParentExpanded) {
expandParent();
}
return super.getPreviousSibling();
}
/**
* @see org.w3c.dom.Node#getNextSibling
*/
public Node getNextSibling() {
if (!fParentExpanded) {
expandParent();
}
return super.getNextSibling();
}
/**
* @see org.w3c.dom.Node#insertBefore
*/
public Node insertBefore(Node newChild,
Node refChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.insertBefore(newChild, refChild);
}
/**
* @see org.w3c.dom.Node#replaceChild
*/
public Node replaceChild(Node newChild,
Node oldChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.replaceChild(newChild, oldChild);
}
/**
* @see org.w3c.dom.Node#removeChild
*/
public Node removeChild(Node oldChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.removeChild(oldChild);
}
/**
* @see org.w3c.dom.Node#appendChild
*/
public Node appendChild(Node newChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.appendChild(newChild);
}
/**
* @see org.w3c.dom.Node#hasChildNodes
*/
public boolean hasChildNodes() {
if (!fChildrenExpanded) {
return fTemplateNode.hasChildNodes();
} else {
return super.hasChildNodes();
}
}
/**
* @see org.w3c.dom.Node#normalize
*/
public void normalize() {
if (!fChildrenExpanded) {
expandChildren();
}
super.normalize();
}
/**
* Return string for debugging. Xerces toString() method calls
* getValue(), which can cause expansion, so we override.
*/
public String toString() {
return getName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy