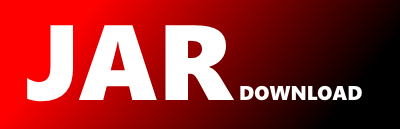
org.enhydra.xml.lazydom.LazyDocument Maven / Gradle / Ivy
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: LazyDocument.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.lazydom;
import org.enhydra.apache.xerces.dom.DocumentImpl;
import org.enhydra.xml.io.OutputOptions;
import org.enhydra.xml.io.PreFormattedTextDocument;
import org.enhydra.xml.xmlc.XMLObject;
import org.enhydra.xml.xmlc.XMLObjectLink;
import org.w3c.dom.Attr;
import org.w3c.dom.CDATASection;
import org.w3c.dom.Comment;
import org.w3c.dom.DOMException;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.Entity;
import org.w3c.dom.EntityReference;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.w3c.dom.Notation;
import org.w3c.dom.ProcessingInstruction;
import org.w3c.dom.Text;
// FIXME: not sure the Lazy* constructors with TemplateNodes are actually
// needed, as we go thorugh the factory methods.
//FIXME: If no childred/attributes/etc exist, initialize expanded flag to true.
// FIXME: need serializable support.
//FIXME: Need clone support.
//FIXME: are createXXX(nodeId) really needed.
/**
* A DOM Document that supports lazy instantiation of a template DOM. Nodes
* in the instance DOM are created as accessed. This can be either by
* traversing the tree or by direct access to a node by id number.
* Instantiation of nodes in the middle of the virtual tree is support. Thus
* a node can exist without a parent being expanded. This is used by XMLC,
* were the dynamic nodes tend to be towards the leaves of the tree.
*
* Instances contain a reference to a DOM that is a shared template for the
* document. Each node in the template is assigned an integer node id that be
* used to index tables to directly look up the template of a node created
* from the template.
*
* This DOM also supports associating pre-formatted text with some nodes, which
* is used to avoid exprensive string scanning operations during the output
* of unmodified nodes.
*
* When a child of a node is requested, all direct children are expanded.
* This eliminates a lot of difficult book keep. Attributes are treated
* as a separate set from children, only instantiated when an atttribute
* is accessed.
*
* Expansion of nodes accesed from an existing node works as follows:
*
* - Lazy nodes have internal flag to indicate if the particular type
* of expansion has occured (parent, child, or attribute).
*
- When an access is made, check the flag to see if the expansion has
* occured.
*
- If expansion has not occured, call the appropriate LazyDocument
* doExpand method, which is synchronized. This method again check
* the flag on the node and expands if necessary. Some expansion
* are only used by one node class and maybe implemented there,
* but still synchronize on the document.
*
- To simplify the book keeping, all children of a node will be expanded
* when one is expanded.
*
- When the parent of a node is requested, the parent and all of the other
* children will be expanded and linked.
*
- Nodes created using create methods (without node ids), are always
* marked as expanded, as they always go through standard DOM methods to
* be inserted into the tree.
*
*
* This coarse-grained locking approach minimizes the number of locks * on the
* assumption that collisions do not occur frequently
*
* To created an extended DOM, one must override both the factory methods that
* take strings and those that take node ids.
*/
public class LazyDocument extends DocumentImpl
implements LazyParent, PreFormattedTextDocument, XMLObjectLink {
/**
* Constructor.
* @param documentType Document type to associate with this document,
* or null if no doctype or should be obtained from template.
* @param templateDOM Template DOM, with each node cotaining a node id.
* Maybe null if no associated template.
*/
public LazyDocument(DocumentType documentType,
TemplateDOM templateDOM) {
super(null); // can't set doctype here (see below)!
if (templateDOM != null) {
fTemplateDOM = templateDOM;
fExpandedNodes = new LazyNode[fTemplateDOM.getMaxNodeId()+1];
fExpandedNodes[DOCUMENT_NODE_ID] = this;
fTemplateNode = (LazyDocument)fTemplateDOM.getNode(DOCUMENT_NODE_ID);
fNodeId = fTemplateNode.getNodeId();
if (documentType != null) {
throw new LazyDOMException(LazyDOMException.NOT_SUPPORTED_ERR,
"can't specify both DocumentType and TemplateDOM");
}
} else {
// No template, mark as expanded.
fDocTypeExpanded = true;
fChildrenExpanded = true;
fIsTemplateNode = true; // No template, so this must be a template.
// Have to wait until here to set up DocumentType. Can't pass it
// to the constructor, as it will call appendChild before the
// above template initialization has taken place. So now we do
// what the constructor would have done.
if (documentType != null) {
try {
((LazyDocumentType)documentType).setOwnerDocument(this);
} catch (ClassCastException e) {
throw new LazyDOMException(LazyDOMException.WRONG_DOCUMENT_ERR,
"DOM005 Wrong document");
}
appendChild(documentType);
}
}
}
/**
* Constructor with no argument, for LazyHTMLDocument.
*/
public LazyDocument() {
this(null, null);
}
/**
* @see Document#getDocumentElement
*/
public Element getDocumentElement() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getDocumentElement();
}
/**
* @see Document#getImplementation
*/
public DOMImplementation getImplementation() {
return LazyDOMImplementation.getDOMImplementation();
}
//-------------------------------------------------------------------------
// Document-wide expansion support (FIXME: move to a different class???)
//-------------------------------------------------------------------------
/**
* Template document DOM. This is shared by all instances and must not be
* modified.
*/
private TemplateDOM fTemplateDOM;
/**
* Table of LazyNodes that have already been expanded, indexed
* by nodeId. Entry is null if the node has not been expanded.
*/
private LazyNode[] fExpandedNodes;
/**
* Detection of recursive calls of one of the expansion routines.
*/
private boolean fExpanding = false;
/**
* Flag that an expansion is in progress, which is used to detect
* recursion. MUST be synchronized on document.
*/
protected final void enterExpansion() {
if (fExpanding) {
throw new LazyDOMError("recursive call doing lazy DOM expansion");
}
fExpanding = true;
}
/**
* Flag that an expansion is complete.
* MUST be synchronized on document.
*/
protected final void leaveExpansion() {
fExpanding = false;
}
/**
* Create a new node from a template, placing it in the expanded table.
* Uses factory methods so that derived document can override node type.
* MUST be synchronized on document.
*/
private LazyNode createLazyNode(int nodeId) {
LazyNode template = fTemplateDOM.getNode(nodeId);
switch (template.getNodeType()) {
case ELEMENT_NODE:
return createElement(nodeId);
case ATTRIBUTE_NODE:
return createAttribute(nodeId);
case TEXT_NODE:
return createTextNode(nodeId);
case CDATA_SECTION_NODE:
return createCDATASection(nodeId);
case ENTITY_REFERENCE_NODE:
return createEntityReference(nodeId);
case ENTITY_NODE:
return createEntity(nodeId);
case PROCESSING_INSTRUCTION_NODE:
return createProcessingInstruction(nodeId);
case COMMENT_NODE:
return createComment(nodeId);
case NOTATION_NODE:
return createNotation(nodeId);
case DOCUMENT_TYPE_NODE:
return createDocumentType(nodeId);
case DOCUMENT_NODE:
case DOCUMENT_FRAGMENT_NODE:
throw new LazyDOMError(nodeId, "Can't create node from template of type "
+ template.getNodeType());
default:
throw new LazyDOMError(nodeId, "Invalid node type "
+ template.getNodeType());
}
}
/**
* Get or create a lazy node, given its id. Expanding if it doesn't
* exist. All node creation must go through here so that a derived
* document create factory is used.
*/
public final LazyNode getNodeById(int nodeId) {
if (fExpandedNodes[nodeId] == null) {
synchronized(this) {
if (fExpandedNodes[nodeId] == null) {
fExpandedNodes[nodeId] = createLazyNode(nodeId);
}
}
}
return fExpandedNodes[nodeId];
}
/**
* Get or create a node given, the template node.
* @see #getNodeById
*/
public final LazyNode getNodeFromTemplate(LazyNode template) {
return getNodeById(template.getNodeId());
}
/**
* Method to expand children of a node once synchronized and fExpanding is
* set.
* MUST be synchronized on document.
*/
private void expandNodeChildren(LazyParent node) {
if (!node.areChildrenExpanded()) {
LazyNode template = node.getTemplateNode();
if (template == null) {
throw new LazyDOMError("bug: expandNodeChildren null template on: "
+ node.getClass()); // FIXME: should be assert.
}
for (LazyNode templateChild = (LazyNode)template.getFirstChild();
templateChild != null;
templateChild = (LazyNode)templateChild.getNextSibling()) {
// Ignore doctype if its a child
if (!(templateChild instanceof DocumentType)) {
LazyNode child = getNodeFromTemplate(templateChild);
node.appendChildWhileExpanding(child);
if (child instanceof LazyParent) {
// Tell child parent is expanded
((LazyParent)child).setParentExpanded();
}
}
}
node.setChildrenExpanded();
}
}
/**
* Do work of expanding the parent of a node, if it is not already
* expanded. This also expands the children, as this keeps the expansion
* bookkeeping simple and getting a parent not a common operation except to
* access a sibling or add a new sibling
* Only used internally to this package.
*/
protected synchronized void doExpandParent(LazyParent node) {
// Check again for it being expanded now that we are synchronized
// getParent used during insertion of new node, so allow for recursion
// without actually expanding.
if (!node.isParentExpanded() && !fExpanding) {
enterExpansion();
try {
LazyParent parentTemplate = (LazyParent)node.getTemplateNode().getParentNode();
if (parentTemplate != null) {
expandNodeChildren((LazyParent)getNodeFromTemplate(parentTemplate));
}
node.setParentExpanded();
} finally {
leaveExpansion();
}
}
}
/**
* Do work of expanding the children of a node, if they are not already
* expanded. Only lazy parent's have a flag for siblings, as data nodes
* can never be accessed without going through the parent and expanding
* the children.
* Only used internally to this package.
*/
protected synchronized void doExpandChildren(LazyParent node) {
enterExpansion();
try {
expandNodeChildren(node);
} finally {
leaveExpansion();
}
}
/**
* Get a pointer to a node if its been expanded, otherwise
* return null.
*/
public final LazyNode getExpandedNode(int nodeId) {
return fExpandedNodes[nodeId];
}
/**
* Get a template node, given a node id.
*/
public final LazyNode getTemplateNode(int nodeId) {
return fTemplateDOM.getNode(nodeId);
}
//-------------------------------------------------------------------------
// LazyDocument specific
//-------------------------------------------------------------------------
/**
* Has the DocumentType been expanded?
*/
private boolean fDocTypeExpanded = false;
/**
* Detection of recursive calls of doctype expansion. A seperate
* flag, since doctype maybe expanded while expanding attributes.
*/
private boolean fExpandingDocType = false;
/**
* Template for this Document.
*/
private LazyDocument fTemplateNode = null;
/**
* Has the DocumentType been expanded?
*/
public boolean isDocTypeExpanded() {
return fDocTypeExpanded;
}
/**
* Get the template for this node.
* @see LazyNode#getTemplateNode
*/
public LazyDocument getTemplateDocument() {
return fTemplateNode;
}
/**
* @see Node#cloneNode
*/
public Node cloneNode(boolean deep) {
// N.B. This closely follows the code in Xerces
// DocumentImpl.cloneNode(), may have to track changes.
if (deep) {
// If children are copied, we must expand now.
if (!fDocTypeExpanded) {
doExpandDocType();
}
if (!fChildrenExpanded) {
expandChildren();
}
}
/**
* This does a clone(), must clean up all fields and make
* fully expanded.
* FIXME: Should support cloning in unexpanded state.
*/
LazyDocument newdoc = new LazyDocument();
newdoc.fTemplateDOM = null;
newdoc.fExpandedNodes = null;
newdoc.fDocTypeExpanded = true;
newdoc.fChildrenExpanded = true;
cloneNode(newdoc, deep);
newdoc.mutationEvents = mutationEvents;
return newdoc;
}
//-------------------------------------------------------------------------
// LazyNode support
//-------------------------------------------------------------------------
/*
* Node id for this element.
*/
private int fNodeId = NULL_NODE_ID;
/**
* Is this a template node?
*/
private boolean fIsTemplateNode;
/*
* @see LazyNode#makeTemplateNode
*/
public void makeTemplateNode(int nodeId) {
fNodeId = nodeId;
fIsTemplateNode = true;
}
/**
* @see LazyNode#getNodeId
*/
public int getNodeId() {
return fNodeId;
}
/**
* @see LazyNode#isTemplateNode
*/
public boolean isTemplateNode() {
return fIsTemplateNode;
}
/**
* @see LazyNode#getTemplateNode
*/
public LazyNode getTemplateNode() {
return fTemplateNode;
}
/**
* @see LazyNode#templateClone
*/
public LazyNode templateClone(Document ownerDocument) {
throw new LazyDOMError("templateClone not support on LazyDocument node");
}
/**
* Set the node value, invalidating the id. All node data is modified
* by this routine.
* @see org.w3c.dom.Node#setNodeValue
*/
public void setNodeValue(String value) {
fNodeId = NULL_NODE_ID;
super.setNodeValue(value);
}
//-------------------------------------------------------------------------
// LazyParent support
//-------------------------------------------------------------------------
/**
* Children expanded flag.
* NB: code is special, since there is node parent.
*/
private boolean fChildrenExpanded = false;
/**
* @see LazyParent#isParentExpanded()
*/
public boolean isParentExpanded() {
return true;
}
/**
* @see LazyParent#setParentExpanded
*/
public void setParentExpanded() {
throw new LazyDOMError("setParentExpanded invalid on document");
}
/**
* @see LazyParent#setParentWhileExpanding
*/
public void setParentWhileExpanding(Node parent) {
throw new LazyDOMError("setParentWhileExpanding invalid on document");
}
/**
* @see LazyParent#areChildrenExpanded()
*/
public boolean areChildrenExpanded() {
return fChildrenExpanded;
}
/**
* @see LazyParent#setChildrenExpanded
*/
public void setChildrenExpanded() {
fChildrenExpanded = true;
}
/**
* @see LazyParent#appendChildWhileExpanding
*/
public void appendChildWhileExpanding(Node child) {
super.insertBefore(child, null);
}
/**
* Expand the parent of this element, if it is not already expanded.
*/
private void expandParent() {
doExpandParent(this);
}
/**
* Expand the children of this element, if they are not already expanded.
*/
private void expandChildren() {
doExpandChildren(this);
}
/**
* @see org.w3c.dom.Node#getChildNodes
*/
public NodeList getChildNodes() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getChildNodes();
}
/**
* @see org.w3c.dom.Node#getFirstChild
*/
public Node getFirstChild() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getFirstChild();
}
/**
* @see org.w3c.dom.Node#getLastChild
*/
public Node getLastChild() {
if (!fChildrenExpanded) {
expandChildren();
}
return super.getLastChild();
}
/**
* @see org.w3c.dom.Node#insertBefore
*/
public Node insertBefore(Node newChild,
Node refChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.insertBefore(newChild, refChild);
}
/**
* @see org.w3c.dom.Node#replaceChild
*/
public Node replaceChild(Node newChild,
Node oldChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.replaceChild(newChild, oldChild);
}
/**
* @see org.w3c.dom.Node#removeChild
*/
public Node removeChild(Node oldChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.removeChild(oldChild);
}
/**
* @see org.w3c.dom.Node#appendChild
*/
public Node appendChild(Node newChild)
throws DOMException {
if (!fChildrenExpanded) {
expandChildren();
}
return super.appendChild(newChild);
}
/**
* @see org.w3c.dom.Node#hasChildNodes
*/
public boolean hasChildNodes() {
if (!fChildrenExpanded) {
return fTemplateNode.hasChildNodes();
} else {
return super.hasChildNodes();
}
}
/**
* @see org.w3c.dom.Node#normalize
*/
public void normalize() {
if (!fChildrenExpanded) {
expandChildren();
}
super.normalize();
}
//-------------------------------------------------------------------------
// DocumentType support
//-------------------------------------------------------------------------
/**
* Expand the document type if needed.
*/
private synchronized void doExpandDocType() {
if (!fDocTypeExpanded) {
if (fExpandingDocType) {
throw new LazyDOMError("recursive call expanding Lazy DOM DocumentType");
}
fExpandingDocType = true;
try {
LazyDocumentType template = (LazyDocumentType)fTemplateNode.getDoctype();
if (template != null) {
docType = (LazyDocumentType)getNodeFromTemplate(template);
}
fDocTypeExpanded = true;
} finally {
fExpandingDocType = false;
}
}
}
/**
* @see Document#getDoctype
*/
public DocumentType getDoctype() {
if (!fDocTypeExpanded) {
doExpandDocType();
}
return super.getDoctype();
}
//-------------------------------------------------------------------------
// Implemention of node org.w3c.dom.Document factories
//-------------------------------------------------------------------------
/**
* @see org.w3c.dom.Document#createElement
*/
public Element createElement(String tagName) throws DOMException {
return new LazyElementNoNS(this, null, tagName);
}
/**
* @see org.w3c.dom.Document#createTextNode
*/
public Text createTextNode(String data) {
return new LazyText(this, null, data);
}
/**
* @see org.w3c.dom.Document#createComment
*/
public Comment createComment(String data) {
return new LazyComment(this, null, data);
}
/**
* @see org.w3c.dom.Document#createCDATASection
*/
public CDATASection createCDATASection(String data) throws DOMException {
return new LazyCDATASection(this, null, data);
}
/**
* @see org.w3c.dom.Document#createProcessingInstruction
*/
public ProcessingInstruction createProcessingInstruction(String target,
String data) {
return new LazyProcessingInstruction(this, null, target, data);
}
/**
* @see org.w3c.dom.Document#createAttribute
*/
public Attr createAttribute(String name) throws DOMException {
return new LazyAttrNoNS(this, null, name);
}
/**
* See org.w3c.dom.Document#createNotation
*/
public Notation createNotation(String name) throws DOMException {
return new LazyNotation(this, null, name, null, null);
}
/**
* @see org.w3c.dom.Document#createEntityReference
*/
public EntityReference createEntityReference(String name) throws DOMException {
return new LazyEntityReference(this, null, name);
}
/**
* @see org.w3c.dom.Document#createElementNS
*/
public Element createElementNS(String namespaceURI,
String qualifiedName) throws DOMException {
return new LazyElementNS(this, null, namespaceURI, qualifiedName);
}
/**
* @see org.w3c.dom.Document#createAttributeNS
*/
public Attr createAttributeNS(String namespaceURI,
String qualifiedName) throws DOMException {
return new LazyAttrNS(this, null, namespaceURI, qualifiedName);
}
/**
* Create a new DocumentType object (Non-DOM).
* @see DocumentImpl#createDocumentType
*/
public DocumentType createDocumentType(String qualifiedName,
String publicID,
String systemID,
String internalSubset) throws DOMException {
return new LazyDocumentType(this, null, qualifiedName, publicID, systemID, internalSubset);
}
/**
* Create a new DocumentType object (Non-DOM). This overrides the method
* in the Xerces DOM used by importNode().
* @see DocumentImpl#createDocumentType
*/
public DocumentType createDocumentType(String qualifiedName,
String publicID,
String systemID) throws DOMException {
return new LazyDocumentType(this, null, qualifiedName, publicID, systemID, null);
}
/**
* Create a new Entity object (Non-DOM).
* @see DocumentImpl#createEntity
*/
public Entity createEntity(String name) throws DOMException {
return new LazyEntity(this, null, name, null, null, null);
}
//-------------------------------------------------------------------------
// Node id-based node creation methods.
//-------------------------------------------------------------------------
/**
* Create a element from a template given its id.
* @see org.w3c.dom.Document#createElement
*/
public LazyElement createElement(int nodeId) throws DOMException {
Node template = (LazyElement)fTemplateDOM.getNode(nodeId);
if (template instanceof LazyElementNS) {
return new LazyElementNS(this, (LazyElementNS)template, null, null);
} else {
return new LazyElementNoNS(this, (LazyElementNoNS)template, null);
}
}
/**
* Create a text node from a template given its id.
* @see org.w3c.dom.Document#createTextNode
*/
public LazyText createTextNode(int nodeId) {
return new LazyText(this, (LazyText)fTemplateDOM.getNode(nodeId), null);
}
/**
* Create a comment from a template given its id.
* @see org.w3c.dom.Document#createComment
*/
public LazyComment createComment(int nodeId) {
return new LazyComment(this, (LazyComment)fTemplateDOM.getNode(nodeId), null);
}
/**
* Create a CDATASection from a template given its id.
* @see org.w3c.dom.Document#createCDATASection
*/
public LazyCDATASection createCDATASection(int nodeId) throws DOMException {
return new LazyCDATASection(this, (LazyCDATASection)fTemplateDOM.getNode(nodeId), null);
}
/**
* Create a process instruction node from a template given its id.
* @see org.w3c.dom.Document#createProcessingInstruction
*/
public LazyProcessingInstruction createProcessingInstruction(int nodeId) {
return new LazyProcessingInstruction(this, (LazyProcessingInstruction)fTemplateDOM.getNode(nodeId), null, null);
}
/**
* Create an attribute from a template given its id.
* @see org.w3c.dom.Document#createAttribute
*/
public LazyAttr createAttribute(int nodeId) throws DOMException {
Node template = fTemplateDOM.getNode(nodeId);
if (template instanceof LazyAttrNS) {
return new LazyAttrNS(this, (LazyAttrNS)template, null, null);
} else {
return new LazyAttrNoNS(this, (LazyAttrNoNS)fTemplateDOM.getNode(nodeId), null);
}
}
/**
* Create a notation node from a template given its id.
* See org.w3c.dom.Document#createNotation
*/
public LazyNotation createNotation(int nodeId) throws DOMException {
return new LazyNotation(this, (LazyNotation)fTemplateDOM.getNode(nodeId),
null, null, null);
}
/**
* Create a entity reference from a template given its id.
* @see org.w3c.dom.Document#createEntityReference
*/
public LazyEntityReference createEntityReference(int nodeId) throws DOMException {
return new LazyEntityReference(this, (LazyEntityReference)fTemplateDOM.getNode(nodeId), null);
}
/**
* Create a new DocumentType object (Non-DOM).
* @see DocumentImpl#createDocumentType
*/
public LazyDocumentType createDocumentType(int nodeId) throws DOMException {
// FIXME: This isn't all that useful, as one can't set doctype after creating
// the document.
return new LazyDocumentType(this, (LazyDocumentType)fTemplateDOM.getNode(nodeId),
null, null, null, null);
}
/**
* Create a new Entity object (Non-DOM).
* @see DocumentImpl#createEntity
*/
public LazyEntity createEntity(int nodeId) throws DOMException {
return new LazyEntity(this, (LazyEntity)fTemplateDOM.getNode(nodeId),
null, null, null, null);
}
//-------------------------------------------------------------------------
// Template node creation methods.
//-------------------------------------------------------------------------
/**
* Create a template element. This will call the derived document create
* method.
*
* @see #createElement(String)
* @see org.w3c.dom.Document#createElement
*/
public LazyElement createTemplateElement(String tagName,
int nodeId,
String preFormattedText) throws DOMException {
LazyElement element = (LazyElement)createElement(tagName);
element.makeTemplateNode(nodeId, preFormattedText);
return element;
}
/**
* Create a template text node. This will call the derived document
* create method.
*
* @see LazyDocument#createTextNode(String)
* @see org.w3c.dom.Document#createTextNode
*/
public LazyText createTemplateTextNode(String data,
int nodeId,
String preFormattedText) {
LazyText text = (LazyText)createTextNode(data);
text.makeTemplateNode(nodeId, preFormattedText);
return text;
}
/**
* Create a template comment. This will call the derived document create
* method.
*
* @see #createComment(String)
* @see org.w3c.dom.Document#createComment
*/
public LazyComment createTemplateComment(String data,
int nodeId) {
LazyComment comment = (LazyComment)createComment(data);
comment.makeTemplateNode(nodeId);
return comment;
}
/**
* Create a template CDATASection. This will call the derived document
* create method.
*
* @see #createCDATASection(String)
* @see org.w3c.dom.Document#createCDATASection
*/
public LazyCDATASection createTemplateCDATASection(String data,
int nodeId) throws DOMException {
LazyCDATASection cdataSection = (LazyCDATASection)createCDATASection(data);
cdataSection.makeTemplateNode(nodeId);
return cdataSection;
}
/**
* Create a template ProcessingInstruction. This will call the derived
* document create method.
*
* @see #createProcessingInstruction(String,String)
* @see org.w3c.dom.Document#createProcessingInstruction
*/
public LazyProcessingInstruction createTemplateProcessingInstruction(String target,
String data,
int nodeId) {
LazyProcessingInstruction pi = (LazyProcessingInstruction)createProcessingInstruction(target, data);
pi.makeTemplateNode(nodeId);
return pi;
}
/**
* Create a template Attr. This will call the derived document create
* method.
*
* @see LazyDocument#createAttribute(String)
* @see org.w3c.dom.Document#createAttribute
*/
public LazyAttr createTemplateAttribute(String name,
int nodeId) throws DOMException {
LazyAttr attr = (LazyAttr)createAttribute(name);
attr.makeTemplateNode(nodeId);
return attr;
}
/**
* Create a template Attr. This will call the derived document create
* method.
*
* @see LazyDocument#createAttributeNS(String,String)
* @see org.w3c.dom.Document#createAttributeNS
*/
public LazyAttr createTemplateAttributeNS(String namespaceURI,
String qualifiedName,
int nodeId) throws DOMException {
LazyAttr attr = (LazyAttr)createAttributeNS(namespaceURI, qualifiedName);
attr.makeTemplateNode(nodeId);
return attr;
}
/**
* Create a template Notation. This will call the derived document create
* method.
*
* See org.w3c.dom.Document#createNotation
* @see #createNotation(String)
*/
public LazyNotation createTemplateNotation(String name,
int nodeId) throws DOMException {
LazyNotation notation = (LazyNotation) createNotation(name);
notation.makeTemplateNode(nodeId);
return notation;
}
/**
* Create a template EntityReference. This will call the derived document
* create method.
*
* @see #createEntityReference(String)
* @see org.w3c.dom.Document#createEntityReference
*/
public LazyEntityReference createTemplateEntityReference(String name,
int nodeId) throws DOMException {
LazyEntityReference entityRef = (LazyEntityReference)createEntityReference(name);
entityRef.makeTemplateNode(nodeId);
return entityRef;
}
/**
* Create a template Element with namespace. This will call the derived
* document create method.
*
* @see #createElementNS(String,String)
* @see org.w3c.dom.Document#createElementNS
*/
public LazyElement createTemplateElementNS(String namespaceURI,
String qualifiedName,
int nodeId,
String preFormattedText) throws DOMException {
LazyElement element = (LazyElement)createElementNS(namespaceURI,
qualifiedName);
element.makeTemplateNode(nodeId, preFormattedText);
return element;
}
/**
* Create a template Attr with namespace. This will call the derived
* document create method.
*
* @see #createAttributeNS(String,String)
* @see org.w3c.dom.Document#createAttributeNS
*/
public LazyAttr createAttributeNS(String namespaceURI,
String qualifiedName,
int nodeId) throws DOMException {
LazyAttr attr = (LazyAttr)createAttributeNS(namespaceURI, qualifiedName);
attr.makeTemplateNode(nodeId);
return attr;
}
/**
* Create a template DocumentType.
*
* @see #createDocumentType(String,String,String,String)
* @see DocumentImpl#createDocumentType
*/
public LazyDocumentType createTemplateDocumentType(String qualifiedName,
String publicID,
String systemID,
String internalSubset,
int nodeId) throws DOMException {
LazyDocumentType docType = new LazyDocumentType(this, null, qualifiedName, publicID,
systemID, internalSubset);
docType.makeTemplateNode(nodeId);
return docType;
}
/**
* Create a template Entity.
*
* @see #createEntity(String)
* @see DocumentImpl#createEntity
*/
public LazyEntity createTemplateEntity(String name,
String publicId,
String systemId,
String notationName,
int nodeId) throws DOMException {
LazyEntity entity = new LazyEntity(this, null, name, publicId,
systemId, notationName);
entity.makeTemplateNode(nodeId);
return entity;
}
//-------------------------------------------------------------------------
// PreFormattedTextDocument specific
//-------------------------------------------------------------------------
/**
* OutputOptions used for creating the preformatted text.
*/
private OutputOptions fPreFormatOutputOptions;
/**
* Set the output options that were used to preformat this document.
* @param outputOptions The output options; should be read-only.
*/
public void setPreFormatOutputOptions(OutputOptions outputOptions) {
fPreFormatOutputOptions = outputOptions;
}
/**
* @see PreFormattedTextDocument#getPreFormatOutputOptions
*/
public OutputOptions getPreFormatOutputOptions() {
return fPreFormatOutputOptions;
}
//-------------------------------------------------------------------------
// XMLObjectLink implementation
//-------------------------------------------------------------------------
/**
* Reference to XMLObject containing this Document.
*/
private XMLObject fXmlObjectLink;
/**
* @see XMLObjectLink#setXMLObject
*/
public void setXMLObject(XMLObject xmlObject) {
fXmlObjectLink = xmlObject;
}
/**
* @see XMLObjectLink#getXMLObject
*/
public XMLObject getXMLObject() {
return fXmlObjectLink;
}
}