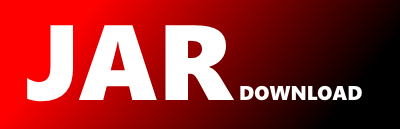
org.enhydra.xml.lazydom.NodeIdMap Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: NodeIdMap.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.lazydom;
import java.util.ArrayList;
import java.util.HashMap;
import org.enhydra.xml.dom.SimpleDOMTraversal;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Node;
/**
* Class to assign and map node ids to a node. Use in the construction of
* template DOMs.
*/
public class NodeIdMap {
/**
* Map of node to node id.
*/
private HashMap fNodeIdMap = new HashMap();
/**
* Map of node id to node. Used to assign node ids.
*/
private ArrayList fIdNodeMap = new ArrayList();
/**
* Constructor.
*/
public NodeIdMap(Document document) {
buildNodeIdMap(document);
}
/**
* Traverse document and assign node ids.
*/
private void buildNodeIdMap(Document document) {
SimpleDOMTraversal traverser
= new SimpleDOMTraversal(new SimpleDOMTraversal.Handler() {
public void handleNode(Node node) {
put(node);
}
});
traverser.traverse(document);
if (fIdNodeMap.get(LazyNode.DOCUMENT_NODE_ID) != document) {
throw new LazyDOMError("document assigned wrong id");
}
}
/**
* Add a node to the map, assigning it id.
*/
private void put(Node node) {
int nodeId = fIdNodeMap.size();
fNodeIdMap.put(node, new Integer(nodeId));
fIdNodeMap.add(node);
}
/**
* Get a node, given its id.
*/
public Node getNode(int id) {
return (Node)fIdNodeMap.get(id);
}
/**
* Get the node id for a node, generating an error if not found.
* If null is passed in, NULL_NODE_ID is returned.
*/
public int getId(Node node) {
if (node == null) {
return LazyNode.NULL_NODE_ID;
}
Integer id = (Integer)fNodeIdMap.get(node);
if (id == null) {
throw new LazyDOMError("Id not found for node: " + node
+ ": " + node.getClass());
}
return id.intValue();
}
/**
* Get an id as a string.
*/
public String getIdStr(Node node) {
return Integer.toString(getId(node));
}
/**
* Get the maximum node id assigned.
*/
public int getMaxNodeId() {
return fIdNodeMap.size()-1;
}
/**
* Get the size of the node map.
*/
public int size() {
return fIdNodeMap.size();
}
/**
* Get the document type node id.
*/
public int getDocumentTypeNodeId(Node node) {
Document document = node.getOwnerDocument();
if (document == null) {
document = (Document)node;
}
DocumentType documentType = document.getDoctype();
if (documentType == null) {
return LazyNode.NULL_NODE_ID;
} else {
return getId(documentType);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy