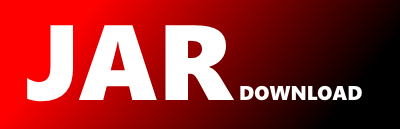
org.enhydra.xml.lazydom.TemplateDOM Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: TemplateDOM.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.lazydom;
import org.enhydra.xml.dom.SimpleDOMTraversal;
import org.w3c.dom.Node;
//FIXME: need to add support for defaultAttrs and elementDefinitons
/**
* Class used to contain hold a Template DOM that is shared by all instance
* of the document. This assign node ids to all nodes in the template,
* mark them as template nodes and makes them read-only.
*/
public final class TemplateDOM {
/** The template document, marked readonly */
private LazyDocument fTemplateDocument;
/** The maximum node id in the document */
private int fMaxNodeId = LazyNode.NULL_NODE_ID;
/** Table, indexed by node id, of nodes in the template doc */
private LazyNode[] fTemplateNodes;
/**
* Constructor.
*/
public TemplateDOM(LazyDocument templateDocument) {
fTemplateDocument = templateDocument;
// Only assigned ids if they don't already exist.
if (fTemplateDocument.getNodeId() == LazyNode.NULL_NODE_ID) {
assignNodeIds();
} else {
findMaxNodeId();
}
initNodeTable();
templateDocument.setReadOnly(true, true);
}
/**
* Assign node ids to all nodes.
*/
private void assignNodeIds() {
fMaxNodeId = LazyNode.DOCUMENT_NODE_ID;
SimpleDOMTraversal traverser
= new SimpleDOMTraversal(new SimpleDOMTraversal.Handler() {
public void handleNode(Node node) {
((LazyNode)node).makeTemplateNode(fMaxNodeId++);
}
});
traverser.traverse(fTemplateDocument);
}
/**
* Find the maximum node id; used when ids are already assigned
*/
private void findMaxNodeId() {
SimpleDOMTraversal traverser
= new SimpleDOMTraversal(new SimpleDOMTraversal.Handler() {
public void handleNode(Node node) {
int nodeId = ((LazyNode)node).getNodeId();
if (nodeId > fMaxNodeId) {
fMaxNodeId = nodeId;
}
}
});
traverser.traverse(fTemplateDocument);
}
/**
* Initialize the node table.
*/
private void initNodeTable() {
fTemplateNodes = new LazyNode[fMaxNodeId+1];
SimpleDOMTraversal traverser
= new SimpleDOMTraversal(new SimpleDOMTraversal.Handler() {
public void handleNode(Node node) {
LazyNode lazyNode = (LazyNode)node;
fTemplateNodes[lazyNode.getNodeId()] = lazyNode;
}
});
traverser.traverse(fTemplateDocument);
}
/**
* Get the maximum node id.
*/
public final int getMaxNodeId() {
return fMaxNodeId;
}
/**
* Get a node, given an id.
* @param nodeId A valid node id for this document, or NULL_NODE_ID.
* @return The template node, or null if NULL_NODE_ID was specified.
*/
public final LazyNode getNode(int nodeId) {
if (nodeId == LazyNode.NULL_NODE_ID) {
return null;
} else {
return fTemplateNodes[nodeId];
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy