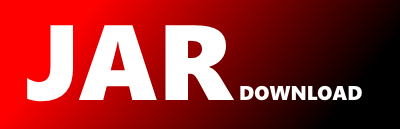
org.enhydra.xml.xhtml.HTMLDomFactory Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Original Code is DigitalSesame
* Portions created by DigitalSesame are Copyright (C) 1997-2000 DigitalSesame
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: HTMLDomFactory.java,v 1.4 2005/10/23 03:23:22 jkjome Exp $
*/
package org.enhydra.xml.xhtml;
import org.enhydra.apache.xerces.dom.DocumentTypeImpl;
import org.enhydra.xml.xhtml.dom.xerces.XHTMLDOMImplementationImpl;
import org.enhydra.xml.xmlc.XMLCError;
import org.enhydra.xml.xmlc.dom.HTMLDomFactoryMethods;
import org.enhydra.xml.xmlc.dom.XMLCDomFactory;
import org.enhydra.xml.xmlc.dom.xerces.XercesDomFactory;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
/**
* XMLC DOM factory for XHTML.
* @see XMLCDomFactory
*/
public class HTMLDomFactory extends XercesDomFactory implements XMLCDomFactory {
private static final String IMPL_CLASS_SUFFIX = "Impl";
private static final String XHTML_IMPLEMENTATION_DOT = "org.enhydra.xml.xhtml.dom.xerces.X";
private static final String XHTML_INTERFACE_DOT = "org.w3c.dom.html.";
/*
* Special-case element names to interface name that can't be
* mapped just by changing the package.
*/
private static final String[][] CLASS_INTERFACE_MAP = {
// Note: the following list was auto-generated by test2 in the
// src/tests/org/enhydra/xml/xhtml directory, as the output file
// 'classes.map'
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLEmElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSmallElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLNoframesElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLBdoElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLDtElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSpanElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLStrongElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLKbdElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLDdElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLBigElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLCodeElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLTbodyElementImpl", "org.w3c.dom.html.HTMLTableSectionElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLTheadElementImpl", "org.w3c.dom.html.HTMLTableSectionElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLTfootElementImpl", "org.w3c.dom.html.HTMLTableSectionElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLUElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLIElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLBElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLTtElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLCenterElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSampElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLDfnElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLNoscriptElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSupElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLStrikeElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLAcronymElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLSubElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLCiteElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLVarElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLAbbrElementImpl", "org.w3c.dom.html.HTMLElement"},
{"org.enhydra.xml.xhtml.dom.xerces.XHTMLAddressElementImpl", "org.w3c.dom.html.HTMLElement"},
};
/**
* @see XMLCDomFactory#createDocumentType
*/
public DocumentType createDocumentType(String qualifiedName,
String publicId,
String systemId,
String internalSubset) {
DOMImplementation domImpl = XHTMLDOMImplementationImpl.getHTMLDOMImplementation();
DocumentTypeImpl docType =
(DocumentTypeImpl)domImpl.createDocumentType(qualifiedName, publicId, systemId);
docType.setInternalSubset(internalSubset);
return docType;
}
/**
* @see XMLCDomFactory#createDocument
*/
public Document createDocument(String namespaceURI,
String qualifiedName,
DocumentType docType) {
if (qualifiedName == null) {
throw new XMLCError("qualifiedName is null for XHTML document;"
+ " maybe trying to use a HTML parser on XHTML");
}
DOMImplementation domImpl = XHTMLDOMImplementationImpl.getHTMLDOMImplementation();
return domImpl.createDocument(namespaceURI, qualifiedName, docType);
}
/**
* The mime type for XHTML is a matter of debate. There are arguments
* for text/xml, text/xhtml, text/html, application/xml,
* or application/xhtml+xml. This returns text/html.
* @see XMLCDomFactory#getMIMEType
* @see XHTMLDomFactory#getMIMEType
*/
public String getMIMEType() {
//return "text/xml";
//text/html for compatibility with non-XHTML compliant user agents.
//See http://www.w3.org/TR/xhtml1/#guidelines C.9
return "text/html";
}
/**
* @see XMLCDomFactory#getBaseClassName
*/
public String getBaseClassName() {
return HTMLDomFactoryMethods.getBaseClassName();
}
/**
* @see XMLCDomFactory#getInterfaceNames
*/
public String[] getInterfaceNames() {
return HTMLDomFactoryMethods.getInterfaceNames();
}
/**
* @see XMLCDomFactory#nodeClassToInterface
*/
public String nodeClassToInterface(Node node) {
String className = node.getClass().getName();
// Search explict class name mappings.
for (int idx = 0; idx < CLASS_INTERFACE_MAP.length; idx++) {
if (className.equals(CLASS_INTERFACE_MAP[idx][0])) {
return CLASS_INTERFACE_MAP[idx][1];
}
}
if (className.startsWith(XHTML_IMPLEMENTATION_DOT)) {
int suffixIdx = className.lastIndexOf(IMPL_CLASS_SUFFIX);
if (suffixIdx < 0) {
throw new XMLCError("Class \"" + className
+ "\" does not have suffix \"" + IMPL_CLASS_SUFFIX
+ "\" (maybe be mismatch between XMLC code DOM implementation)");
}
return XHTML_INTERFACE_DOT +
className.substring(XHTML_IMPLEMENTATION_DOT.length(), suffixIdx);
} else {
// Unable to find or translate the node implementation class to a suitable
// iterface, so throw an exception.
throw new XMLCError("Can't determine DOM interface for node of class \""
+ className
+ "\" (maybe be mismatch between XMLCDomFactory DOM implementation)");
}
}
/**
* @see XMLCDomFactory#getElementClassNames
*/
public String[] getElementClassNames(Element element) {
return HTMLDomFactoryMethods.getElementClassNames(element);
}
/**
* @see XMLCDomFactory#isURLAttribute
*/
public boolean isURLAttribute(Element element,
String attrName) {
return HTMLDomFactoryMethods.isURLAttribute(element, attrName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy