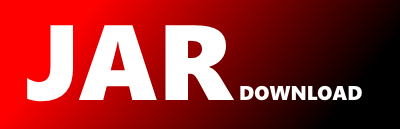
org.enhydra.xml.xhtml.dom.xerces.XHTMLOptionElementImpl Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Original Code is DigitalSesame
* Portions created by DigitalSesame are Copyright (C) 1997-2000 DigitalSesame
* All Rights Reserved.
*
* Contributor(s):
* Rex Tsai
* David Li
*
* $Id: XHTMLOptionElementImpl.java,v 1.2 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xhtml.dom.xerces;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.w3c.dom.Text;
import org.w3c.dom.html.HTMLElement;
import org.w3c.dom.html.HTMLSelectElement;
public class XHTMLOptionElementImpl
extends XHTMLElementImpl
implements org.enhydra.xml.xhtml.dom.XHTMLOptionElement
{
public XHTMLOptionElementImpl (XHTMLDocumentBase owner, String namespaceURI, String tagName) {
super( owner, namespaceURI, tagName);
}
public void setId (String newValue) {
setAttribute("id", newValue);
}
public String getId () {
return getAttribute ("id");
}
public void setLang (String newValue) {
setAttribute("lang", newValue);
}
public String getLang () {
return getAttribute ("lang");
}
public void setDir (String newValue) {
setAttribute("dir", newValue);
}
public String getDir () {
return getAttribute ("dir");
}
public void setClassName (String newValue) {
setAttribute("class", newValue);
}
public String getClassName () {
return getAttribute ("class");
}
public void setTitle (String newValue) {
setAttribute("title", newValue);
}
public String getTitle () {
return getAttribute ("title");
}
public void setValue (String newValue) {
setAttribute("value", newValue);
}
public String getValue () {
return getAttribute ("value");
}
public void setDisabled (boolean newValue) {
setAttribute("disabled", newValue);
}
public boolean getDisabled () {
return getBooleanAttribute("disabled");
}
public void setLabel (String newValue) {
setAttribute("label", newValue);
}
public String getLabel () {
return getAttribute ("label");
}
public void setDefaultSelected (boolean newValue) {
setAttribute("defaultselected", newValue);
}
public boolean getDefaultSelected () {
return getBooleanAttribute("defaultselected");
}
public void setSelected (boolean newValue) {
setAttribute("selected", newValue);
}
public boolean getSelected () {
return getBooleanAttribute("selected");
}
public void setOnKeyUp (String newValue) {
setAttribute("onkeyup", newValue);
}
public String getOnKeyUp () {
return getAttribute ("onkeyup");
}
public void setStyle (String newValue) {
setAttribute("style", newValue);
}
public String getStyle () {
return getAttribute ("style");
}
public void setOnMouseDown (String newValue) {
setAttribute("onmousedown", newValue);
}
public String getOnMouseDown () {
return getAttribute ("onmousedown");
}
public void setOnKeyPress (String newValue) {
setAttribute("onkeypress", newValue);
}
public String getOnKeyPress () {
return getAttribute ("onkeypress");
}
public void setOnDblClick (String newValue) {
setAttribute("ondblclick", newValue);
}
public String getOnDblClick () {
return getAttribute ("ondblclick");
}
public void setOnKeyDown (String newValue) {
setAttribute("onkeydown", newValue);
}
public String getOnKeyDown () {
return getAttribute ("onkeydown");
}
public void setOnMouseMove (String newValue) {
setAttribute("onmousemove", newValue);
}
public String getOnMouseMove () {
return getAttribute ("onmousemove");
}
public void setOnMouseUp (String newValue) {
setAttribute("onmouseup", newValue);
}
public String getOnMouseUp () {
return getAttribute ("onmouseup");
}
public void setXmlLang (String newValue) {
setAttribute("xml:lang", newValue);
}
public String getXmlLang () {
return getAttribute ("xml:lang");
}
public void setOnMouseOut (String newValue) {
setAttribute("onmouseout", newValue);
}
public String getOnMouseOut () {
return getAttribute ("onmouseout");
}
public void setOnClick (String newValue) {
setAttribute("onclick", newValue);
}
public String getOnClick () {
return getAttribute ("onclick");
}
public void setOnMouseOver (String newValue) {
setAttribute("onmouseover", newValue);
}
public String getOnMouseOver () {
return getAttribute ("onmouseover");
}
;
public String getText() {
Node child;
String text;
// Find the Text nodes contained within this element and return their
// concatenated value. Required to go around comments, entities, etc.
child = getFirstChild();
text = "";
while ( child != null )
{
if ( child instanceof Text )
text = text + ( (Text) child ).getData();
child = child.getNextSibling();
}
return text;
}
public void setText( String text ) {
Node child;
Node next;
// Delete all the nodes and replace them with a single Text node.
// This is the only approach that can handle comments and other nodes.
child = getFirstChild();
while ( child != null )
{
next = child.getNextSibling();
removeChild( child );
child = next;
}
insertBefore( getOwnerDocument().createTextNode( text ), getFirstChild() );
}
public int getIndex() {
Node parent;
NodeList options;
int i;
// Locate the parent SELECT. Note that this OPTION might be inside a
// OPTGROUP inside the SELECT. Or it might not have a parent SELECT.
// Everything is possible. If no parent is found, return -1.
parent = getParentNode();
while ( parent != null && ! ( parent instanceof HTMLSelectElement ) )
parent = parent.getParentNode();
if ( parent != null )
{
// Use getElementsByTagName() which creates a snapshot of all the
// OPTION elements under the SELECT. Access to the returned NodeList
// is very fast and the snapshot solves many synchronization problems.
options = ( (HTMLElement) parent ).getElementsByTagName( "option" );
for ( i = 0 ; i < options.getLength() ; ++i )
if ( options.item( i ) == this )
return i;
}
return -1;
}
public void setIndex( int index ) {
Node parent;
NodeList options;
Node item;
// Locate the parent SELECT. Note that this OPTION might be inside a
// OPTGROUP inside the SELECT. Or it might not have a parent SELECT.
// Everything is possible. If no parent is found, just return.
parent = getParentNode();
while ( parent != null && ! ( parent instanceof HTMLSelectElement ) )
parent = parent.getParentNode();
if ( parent != null ) {
// Use getElementsByTagName() which creates a snapshot of all the
// OPTION elements under the SELECT. Access to the returned NodeList
// is very fast and the snapshot solves many synchronization problems.
// Make sure this OPTION is not replacing itself.
options = ( (HTMLElement) parent ).getElementsByTagName( "option" );
if ( options.item( index ) != this ) {
// Remove this OPTION from its parent. Place this OPTION right
// before indexed OPTION underneath it's direct parent (might
// be an OPTGROUP).
getParentNode().removeChild( this );
item = options.item( index );
item.getParentNode().insertBefore( this, item );
}
}
}
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy