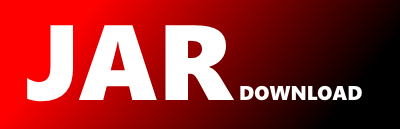
org.enhydra.xml.xmlc.Log4jXMLCLogger Maven / Gradle / Ivy
The newest version!
/**
Log4jXMLCLogger - XMLC logger interface to the enhydra logger
Copyright (C) 2002-2003 Together
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
Log4jXMLCLogger.java
Date: 14.07.2003.
@version 1.0.0
@author: Milosevic Sinisa [email protected]
*/
package org.enhydra.xml.xmlc;
import org.apache.log4j.Level;
/**
* XMLC logger interface to the enhydra logger.
*/
public class Log4jXMLCLogger implements XMLCLogger {
/**
* Constant for name of class to use when recording caller
* of log method. Appending a dot at the end is recommended practice.
*/
private static final String FQCN = Log4jXMLCLogger.class.getName() + ".";
//underlying implementation
private final org.apache.log4j.Logger m_logger;
/**
* Create a logger that delegates to specified category.
*
* @param logImpl the category to delegate to
*/
public Log4jXMLCLogger(final org.apache.log4j.Logger logImpl) {
m_logger = logImpl;
}
/**
* Determine if messages of priority "info" will be logged.
*
* @return true if "info" messages will be logged
*/
public final boolean infoEnabled() {
return m_logger.isInfoEnabled();
}
/**
* Log a info message.
*
* @param message the message
*/ public final void logInfo(final String message) {
m_logger.log(FQCN, Level.INFO, message, null );
}
/**
* Log a info message.
*
* @param message the message
* @param throwable the throwable
*/
public final void logInfo(final String message, final Throwable throwable) {
m_logger.log( FQCN, Level.WARN, message, throwable );
}
/**
* Determine if messages of priority "error" will be logged.
*
* @return true if "error" messages will be logged
*/
public final boolean errorEnabled() {
return m_logger.isEnabledFor(Level.ERROR);
}
/**
* Log a error message.
*
* @param message the message
*/
public final void logError(final String message) {
m_logger.log( FQCN, Level.ERROR, message, null );
}
/**
* Log a error message.
*
* @param message the message
* @param throwable the throwable
*/
public final void logError(final String message, final Throwable throwable) {
m_logger.log( FQCN, Level.ERROR, message, throwable );
}
/**
* Determine if messages of priority "debug" will be logged.
*
* @return true if "debug" messages will be logged
*/
public boolean debugEnabled() {
return m_logger.isDebugEnabled();
}
/**
* Log a debug message.
*
* @param message the message
*/
public final void logDebug(final String message) {
m_logger.log(FQCN, Level.DEBUG, message, null );
}
/**
* Log a debug message.
*
* @param message the message
* @param throwable the throwable
*/
public void logDebug(final String message, final Throwable throwable) {
m_logger.log( FQCN, Level.DEBUG, message, throwable);
}
/**
* Create a new child logger.
* The name of the child logger is [current-loggers-name].[passed-in-name]
* Throws IllegalArgumentException
if name has an empty element name
*
* @param name the subname of this logger
* @return the new logger
*/
public final XMLCLogger getChildLogger(final String name) {
return new Log4jXMLCLogger(
org.apache.log4j.Logger.getLogger(m_logger.getName() + "." + name));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy