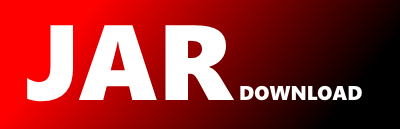
org.enhydra.xml.xmlc.StreamXMLCLogger Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: StreamXMLCLogger.java,v 1.1.1.1 2003/03/10 16:36:19 taweili Exp $
*/
package org.enhydra.xml.xmlc;
import java.io.PrintWriter;
import java.io.StringWriter;
/**
* XMLC logger interface to PrintWriter streams.
*/
public class StreamXMLCLogger implements XMLCLogger {
/**
* Writer for XMLC informative logging or null if not enabled.
*/
private PrintWriter fInfoWriter;
/**
* Writer for XMLC debug logging or null if not enabled.
*/
private PrintWriter fDebugWriter;
/**
* Writer for XMLC error logging or null if no logging.
*/
private PrintWriter fErrorWriter;
/**
* Constructor with no streams specified. No loggin is initially enabled.
*/
public StreamXMLCLogger() {
}
/**
* Constructor specifing a single stream to be used for both info
* and error logging.
*/
public StreamXMLCLogger(PrintWriter logWriter) {
fInfoWriter = logWriter;
fErrorWriter = logWriter;
}
/**
* Constructor specifing a streams for info, error and debug.
* Any maybe null to disable.
*/
public StreamXMLCLogger(PrintWriter infoWriter,
PrintWriter errorWriter,
PrintWriter debugWriter) {
fInfoWriter = infoWriter;
fErrorWriter = errorWriter;
fDebugWriter = debugWriter;
}
/**
* Log an error message with an exception
*/
private void logExcept(PrintWriter writer,
String msg,
Throwable except) {
if (writer != null) {
// Get stack trace formatted
StringWriter trace = new StringWriter(1024);
PrintWriter ptrace = new PrintWriter(trace, true);
except.printStackTrace(ptrace);
writer.println(msg + "\n" + except.toString()
+ "\n" + trace);
writer.flush();
}
}
/**
* Determine if info logging is enabled.
*/
public boolean infoEnabled() {
return (fInfoWriter != null);
}
/**
* Get the info logging writer.
*/
public PrintWriter getInfoWriter() {
return fInfoWriter;
}
/**
* Set the info logging writer; disable by setting to null.
*/
public void setInfoWriter(PrintWriter writer) {
fInfoWriter = writer;
}
/**
* Login an info message.
*/
public void logInfo(String msg) {
if (fInfoWriter != null) {
fInfoWriter.println(msg);
fInfoWriter.flush();
}
}
/**
* Login an info message with exception.
*/
public void logInfo(String msg,
Throwable except) {
if (fInfoWriter != null) {
logExcept(fInfoWriter, msg, except);
}
}
/**
* Determine if error logging is enabled.
*/
public boolean errorEnabled() {
return (fErrorWriter != null);
}
/**
* Get the error logging writer.
*/
public PrintWriter getErrorWriter() {
return fErrorWriter;
}
/**
* Set the error logging writer; disable by setting to null.
*/
public void setErrorWriter(PrintWriter writer) {
fErrorWriter = writer;
}
/**
* Login an error message.
*/
public void logError(String msg) {
if (fErrorWriter != null) {
fErrorWriter.println(msg);
fErrorWriter.flush();
}
}
/**
* Login an error message with exception.
*/
public void logError(String msg,
Throwable except) {
if (fErrorWriter != null) {
logExcept(fErrorWriter, msg, except);
}
}
/**
* Determine if debug logging is enabled.
*/
public boolean debugEnabled() {
return (fDebugWriter != null);
}
/**
* Get the debug logging writer.
*/
public PrintWriter getDebugWriter() {
return fDebugWriter;
}
/**
* Set the debug logging writer; disable by setting to null.
*/
public void setDebugWriter(PrintWriter writer) {
fDebugWriter = writer;
}
/**
* Login an debug message.
*/
public void logDebug(String msg) {
if (fDebugWriter != null) {
fDebugWriter.println(msg);
fDebugWriter.flush();
}
}
/**
* Login an debug message with exception.
*/
public void logDebug(String msg,
Throwable except) {
if (fDebugWriter != null) {
logExcept(fDebugWriter, msg, except);
}
}
/**
* Close all streams associated with this object.
*/
public void close() {
if (fInfoWriter != null) {
fInfoWriter.close();
}
if (fErrorWriter != null) {
fErrorWriter.close();
}
if (fDebugWriter != null) {
fDebugWriter.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy