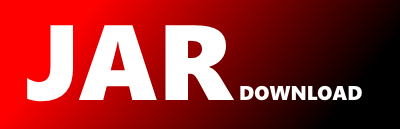
org.enhydra.xml.xmlc.XMLCUtil Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLCUtil.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc;
import java.io.OutputStream;
import java.io.PrintWriter;
import org.enhydra.xml.dom.DOMInfo;
import org.enhydra.xml.dom.DOMOps;
import org.w3c.dom.Attr;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.Text;
//FIXME: move to DOMOps..
/**
* Utility methods for dealing with DOMs.
*/
public abstract class XMLCUtil {
/**
* Find an attribute of a node by name.
*
* @param node Node who's attribute is desired.
* @param name The name of the desired attribute.
* @return The attribute or null if its not found.
* @deprecated use org.w3c.dom.Element#getAttributeNode
* @see Element#getAttributeNode
*/
public static Attr getAttributeByName(Node node, String name) {
NamedNodeMap nodeMap = node.getAttributes();
if (nodeMap == null) {
return null;
} else {
return (Attr)nodeMap.getNamedItem(name);
}
}
/**
* Find the first text descendent node of an element.
* This recursively looks more than one level to search
* for text in font nodes, etc.
*
* @param node The starting node for the search.
* @return The text node or null if not found.
*/
public static Text findFirstText(Node node) {
if (node instanceof Text) {
return (Text)node;
}
for (Node child = node.getFirstChild(); child != null;
child = child.getNextSibling()) {
Text text = findFirstText(child);
if (text != null) {
return text;
}
}
return null;
}
/**
* Find the first text descendent node of an element.
* This recursively looks more than one level to search
* for text in font nodes, etc. If a text node is not
* found, generate an error.
*
* @param node The starting node for the search.
* @return The text node.
* @exception XMLCError If a text node was not found.
*/
public static Text getFirstText(Node node) {
Text text = findFirstText(node);
if (text == null) {
String msg = "No child text mode found for element";
Attr id = getAttributeByName(node, "id");
if (id != null) {
msg += "; id=\"" + id.getValue() + "\"";
}
throw new XMLCError(msg);
}
return text;
}
/**
* Recursively search for an element by id starting at a node.
* Assumes id attribute is named "id". With XMLC, it normally
* better to access elements in a compiled objects directly with
* the generated access methods. This is provided for working on
* cloned pieces of the document or other tasks outside of the XMLC
* generated object.
*
* @param id The element id to find. Case is ignored.
* @param node The node to start the search at.
* @return A point the element if found, otherwise null.
*/
public static Element getElementById(String id, Node node) {
//FIXME: make LazyDOM aware
if (node instanceof Element) {
Element elem = (Element)node;
Attr nodeId = getAttributeByName(elem, "id");
if ((nodeId != null) && nodeId.getValue().equalsIgnoreCase(id)) {
return (Element)node;
}
}
// Search children
for (Node child = node.getFirstChild(); child != null;
child = child.getNextSibling()) {
Element childElem = getElementById(id, child);
if (childElem != null) {
return childElem;
}
}
return null;
}
/**
* Recursively search for an required element by id starting at a node.
*
* @param id The element id to find. Case is ignored.
* @param node The node to start the search at.
* @return A point the element if found.
* @exception XMLCError Thrown if the element is not found.
* @see #getElementById
*/
public static Element getRequiredElementById(String id, Node node) {
Element elem = getElementById(id, node);
if (elem == null) {
// Not found, generate an error.
String msg = "No element found for id \"" + id + "\"";
Attr nodeId = getAttributeByName(node, "id");
if (nodeId != null) {
msg += "; starting at id=\"" + nodeId.getValue() + "\"";
}
throw new XMLCError(msg);
} else {
return elem;
}
}
/**
* Copy a node and its children from one document to another.
*
* @param srcNode The node to copy.
* @param destDocument The destination document. The node will
* belong to this document but will not be inserted in it.
* @return The new node.
* @deprecated use org.w3c.dom.Document.importNode
* @see Document#importNode
*/
public static Node copyNode(Node srcNode, Document destDocument) {
return destDocument.importNode(srcNode, true);
}
/**
* Replace a node with a given one from another document.
* @param srcElement The node to clone and insert
* @param destElement The node to be replaced
* @return the new node that replaces the destination node
* @deprecated use org.enhydra.xml.dom.DOMOps#replaceNode
* @see DOMOps#replaceNode
*/
public static Node replaceNode(Node srcNode, Node destNode) {
return DOMOps.replaceNode(srcNode, destNode);
}
// Deprecated functions for 3.0 compatibility.
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
*/
public static final int PRINT_COMMENT = 0x00;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
*/
public static final int PRINT_TEXT = 0x00;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
* Option to printNode to print the contents of Text nodes.
*/
public static final int PRINT_CDATA = 0x00;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
* Option to printNode to print the contents of DocumentType nodes.
*/
public static final int PRINT_DOCUMENTTYPE = 0x00;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
* All Print options.
*/
public static final int PRINT_ALL = DOMInfo.PRINT_ALL;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
* Default print options.
*/
public static final int PRINT_DEFAULT = DOMInfo.PRINT_DEFAULT;
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
*/
public static void printNode(String msg, Node node,
int options,
PrintWriter out) {
DOMInfo.printTree(msg, node, options, out);
}
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
*/
public static void printNode(String msg, Node node,
PrintWriter out) {
DOMInfo.printTree(msg, node, out);
}
/**
* @deprecated Use DOMInfo
* @see DOMInfo#printTree
*/
public static void printNode(String msg, Node node,
OutputStream out) {
DOMInfo.printTree(msg, node, out);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy