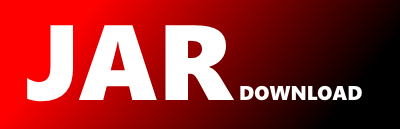
org.enhydra.xml.xmlc.XMLObject Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLObject.java,v 1.3 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc;
import org.enhydra.xml.io.DocumentInfo;
import org.w3c.dom.DOMException;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
/**
* Interface for all compiled XML objects.
*/
public interface XMLObject extends Document, DocumentInfo {
/**
* The name of the class field that is used to identify an XMLC
* generated class. The field will contain a reference to the
* Class object for the class. This is used to find the XMLC generated
* class in an inheritance hierarchy.
*/
public static final String XMLC_GENERATED_CLASS_FIELD_NAME
= "XMLC_GENERATED_CLASS";
/**
* Field name in the generated class that contains the
* name of the source file. This is used to find
* the source file for recompilation. It is a relative
* file path, normally the name of the file within the
* package containing the class.
*/
public static final String XMLC_SOURCE_FILE_FIELD_NAME
= "XMLC_SOURCE_FILE";
/**
* Generated method to build the DOM. This is normally call by the
* constructor. However, one of the generated constructors takes a boolean
* flag to indicate if this should be called automatically. This method
* may also be called to reinitialize the object to its empty state.
*/
public void buildDocument();
/**
* Set the delegate object. Delegation is used to support automatic
* recompilation of documents into XMLC objects. If the delegate is
* not null, the methods of the delegate are called to handle most
* of the methods of this object.
*
* @param delegate The delegate object, or null to remove delegation.
* The delegate must implement the same interface as the derived, generate
* object.
*/
public void setDelegate(XMLObject delegate);
/**
* Initialize the fields used by the generated access methods from the
* current state of the document. Missing DOM element ids will result in
* their acccess method returning null
.
*/
public void syncAccessMethods();
/**
* Get the delegate.
* @return The delegate object, or null if there is no delegate.
*/
public XMLObject getDelegate();
/**
* Get the actual document object. One should normally just use the
* XMLObject methods to access the Document functionality, this is for the
* initialization of derived objects.
*/
public Document getDocument();
/**
* Get the MIME type associated with the document, or null if none was
* associated.
*/
public String getMIMEType();
/**
* Get the encoding specified in the document. Note that this is the
* symbolic name of the XML encoding, which is not the same as the
* Java encoding names.
*
* @return the encoding or null if no encoding was explicitly
* specified.
* @see org.enhydra.xml.io.DOMFormatter
* @see org.enhydra.xml.io.OutputOptions
*/
public String getEncoding();
/**
* See org.w3c.dom.Document#setEncoding
*/
public void setEncoding(String encoding);
/**
* See org.w3c.dom.Document#getStandalone
*/
public boolean getStandalone();
/**
* See org.w3c.dom.Document#setStandalone
*/
public void setStandalone(boolean standalone);
/**
* See org.w3c.dom.Document#getStrictErrorChecking
*/
public boolean getStrictErrorChecking();
/**
* See org.w3c.dom.Document#setStrictErrorChecking
*/
public void setStrictErrorChecking(boolean strictErrorChecking);
/**
* See org.w3c.dom.Document#getVersion()
*/
public String getVersion();
/**
* See org.w3c.dom.Document#setVersion
*/
public void setVersion(String version);
/**
* See org.w3c.dom.Document#adoptNode
*/
public Node adoptNode(Node source) throws DOMException;
/**
* Convert the document to a string representation of the document, that
* is a string containing XML. The results are parsable into the same
* DOM hierarchy. The formatting provided by this method does not
* begin to cover all of the issues involved with publishing
* a XML document, such as character encoding. Use the
* {@link org.enhydra.xml.io.DOMFormatter DOMFormatter} class if
* more options are required.
*
* @return A string containing the full XML.
* @see org.enhydra.xml.io.DOMFormatter
*/
public String toDocument();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy