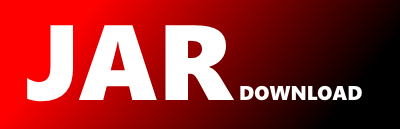
org.enhydra.xml.xmlc.XMLObjectImpl Maven / Gradle / Ivy
The newest version!
/*
* Enhydra Java Application Server Project
*
* The contents of this file are subject to the Enhydra Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License on
* the Enhydra web site ( http://www.enhydra.org/ ).
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific terms governing rights and limitations
* under the License.
*
* The Initial Developer of the Enhydra Application Server is Lutris
* Technologies, Inc. The Enhydra Application Server and portions created
* by Lutris Technologies, Inc. are Copyright Lutris Technologies, Inc.
* All Rights Reserved.
*
* Contributor(s):
*
* $Id: XMLObjectImpl.java,v 1.5 2005/01/26 08:29:24 jkjome Exp $
*/
package org.enhydra.xml.xmlc;
import org.enhydra.xml.dom.DOMOps;
import org.enhydra.xml.io.DOMFormatter;
import org.enhydra.xml.io.DocumentInfo;
import org.enhydra.xml.xmlc.dom.XMLCDomFactory;
import org.w3c.dom.Attr;
import org.w3c.dom.CDATASection;
import org.w3c.dom.Comment;
import org.w3c.dom.DOMConfiguration;
import org.w3c.dom.DOMException;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentFragment;
import org.w3c.dom.DocumentType;
import org.w3c.dom.Element;
import org.w3c.dom.EntityReference;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.w3c.dom.ProcessingInstruction;
import org.w3c.dom.Text;
import org.w3c.dom.UserDataHandler;
//FIXME: Should getDomFactory be in interface?
//FIXME: Should domFactory be be pasted.
/**
* Base class for all compiled XML objects.
* @see XMLObject
*/
public abstract class XMLObjectImpl implements XMLObject, Document, DocumentInfo {
/**
* Default XML formatting object for use by toDocument(). Initialized
* on first use and shared
*/
private static DOMFormatter fFormatter = null;
/**
* The document the object is associated with.
*/
private Document fDocument;
/**
* The MIME type associated with the document.
*/
private String fMIMEType;
/**
* The delegate object.
*/
private XMLObject fDelegate;
/**
* Constructor. The setDocument() method must be called to
* associate a document with this object.
*/
protected XMLObjectImpl() {
}
/**
* Set the DOM document associated with this object and optional encoding.
* This is used by buildDocument() to set the new document. It is done
* separately from the constructor to allow buildDocument() to not be
* called immediatly.
*/
protected void setDocument(Document document,
String mimeType,
String encoding) {
fDocument = document;
fMIMEType = mimeType;
setEncoding(encoding);
// Link Document back to this object if it supports XMLObjectLink
if (document instanceof XMLObjectLink) {
((XMLObjectLink)document).setXMLObject(this);
}
}
/**
* Get the XMLC DOM Factory associated with this document type and DOM
* implementation.
*/
protected abstract XMLCDomFactory getDomFactory();
/**
* @see XMLObject#getDocument
*/
public Document getDocument() {
if (fDelegate != null) {
return fDelegate.getDocument();
} else {
return fDocument;
}
}
/**
* @see XMLObject#getMIMEType
*/
public String getMIMEType() {
if (fDelegate != null) {
return fDelegate.getMIMEType();
} else {
return fMIMEType;
}
}
/**
* @see XMLObject#setDelegate
*/
public void setDelegate(XMLObject delegate) {
fDelegate = delegate;
}
/**
* @see XMLObject#getDelegate
*/
public XMLObject getDelegate() {
return fDelegate;
}
/**
* Check that cloneNode on an entire document is done with the
* deep option.
*/
protected void cloneDeepCheck(boolean deep) {
if (!deep) {
throw new XMLCError("Must use deep clone when cloning entire document");
}
}
/**
* Clone the entire document. Derived objects should override this
* to get the correct derived type. Cloning with deep being false
* is not allowed.
*
* @see org.w3c.dom.Node#cloneNode
*/
abstract public Node cloneNode(boolean deep);
/**
* @see org.w3c.dom.Document#getDoctype
*/
public DocumentType getDoctype() {
if (fDelegate != null) {
return fDelegate.getDoctype();
} else {
return fDocument.getDoctype();
}
}
/**
* @see org.w3c.dom.Document#getImplementation
*/
public DOMImplementation getImplementation() {
if (fDelegate != null) {
return fDelegate.getImplementation();
} else {
return fDocument.getImplementation();
}
}
/**
* @see org.w3c.dom.Document#getDocumentElement
*/
public Element getDocumentElement() {
if (fDelegate != null) {
return fDelegate.getDocumentElement();
} else {
return fDocument.getDocumentElement();
}
}
/**
* @see org.w3c.dom.Document#importNode
*/
public Node importNode(Node importedNode,
boolean deep)
throws DOMException {
if (fDelegate != null) {
return fDelegate.importNode(importedNode, deep);
} else {
return fDocument.importNode(importedNode, deep);
}
}
/**
* @see org.w3c.dom.Document#createElement
*/
public Element createElement(String tagName)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createElement(tagName);
} else {
return fDocument.createElement(tagName);
}
}
/**
* @see org.w3c.dom.Document#createElementNS
*/
public Element createElementNS(String namespaceURI,
String qualifiedName)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createElementNS(namespaceURI, qualifiedName);
} else {
return fDocument.createElementNS(namespaceURI, qualifiedName);
}
}
/**
* @see org.w3c.dom.Document#createDocumentFragment
*/
public DocumentFragment createDocumentFragment() {
if (fDelegate != null) {
return fDelegate.createDocumentFragment();
} else {
return fDocument.createDocumentFragment();
}
}
/**
* @see org.w3c.dom.Document#createTextNode
*/
public Text createTextNode(String data) {
if (fDelegate != null) {
return fDelegate.createTextNode(data);
} else {
return fDocument.createTextNode(data);
}
}
/**
* @see org.w3c.dom.Document#createComment
*/
public Comment createComment(String data) {
if (fDelegate != null) {
return fDelegate.createComment(data);
} else {
return fDocument.createComment(data);
}
}
/**
* @see org.w3c.dom.Document#createCDATASection
*/
public CDATASection createCDATASection(String data)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createCDATASection(data);
} else {
return fDocument.createCDATASection(data);
}
}
/**
* @see org.w3c.dom.Document#createProcessingInstruction
*/
public ProcessingInstruction createProcessingInstruction(String target,
String data)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createProcessingInstruction(target, data);
} else {
return fDocument.createProcessingInstruction(target, data);
}
}
/**
* @see org.w3c.dom.Document#createAttribute
*/
public Attr createAttribute(String qualifiedName)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createAttribute(qualifiedName);
} else {
return fDocument.createAttribute(qualifiedName);
}
}
/**
* @see org.w3c.dom.Document#createAttributeNS
*/
public Attr createAttributeNS(String namespaceURI,
String qualifiedName)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createAttributeNS(namespaceURI, qualifiedName);
} else {
return fDocument.createAttributeNS(namespaceURI, qualifiedName);
}
}
/**
* @see org.w3c.dom.Document#createEntityReference
*/
public EntityReference createEntityReference(String name)
throws DOMException {
if (fDelegate != null) {
return fDelegate.createEntityReference(name);
} else {
return fDocument.createEntityReference(name);
}
}
/**
* @see org.w3c.dom.Document#getElementsByTagName
*/
public NodeList getElementsByTagName(String tagname) {
if (fDelegate != null) {
return fDelegate.getElementsByTagName(tagname);
} else {
return fDocument.getElementsByTagName(tagname);
}
}
/**
* @see org.w3c.dom.Document#getElementsByTagNameNS
*/
public NodeList getElementsByTagNameNS(String namespaceURI,
String localName) {
if (fDelegate != null) {
return fDelegate.getElementsByTagNameNS(namespaceURI, localName);
} else {
return fDocument.getElementsByTagNameNS(namespaceURI, localName);
}
}
/**
* @see org.w3c.dom.Document#getElementById
*/
public Element getElementById(String elementId) {
if (fDelegate != null) {
return fDelegate.getElementById(elementId);
} else {
return fDocument.getElementById(elementId);
}
}
/**
* See org.w3c.dom.Document#getEncoding
*/
public String getEncoding() {
if (fDelegate != null) {
return fDelegate.getEncoding();
} else {
return DOMOps.getEncoding(fDocument);
}
}
/**
* See org.w3c.dom.Document#setEncoding
*/
public void setEncoding(String encoding) {
if (fDelegate != null) {
fDelegate.setEncoding(encoding);
} else {
DOMOps.setEncoding(fDocument, encoding);
}
}
/**
* See org.w3c.dom.Document#getStandalone
*/
public boolean getStandalone() {
if (fDelegate != null) {
return fDelegate.getStandalone();
} else {
return DOMOps.getStandalone(fDocument);
}
}
/**
* See org.w3c.dom.Document#setStandalone
*/
public void setStandalone(boolean standalone) {
if (fDelegate != null) {
fDelegate.setStandalone(standalone);
} else {
DOMOps.setStandalone(fDocument, standalone);
}
}
/**
* See org.w3c.dom.Document#getStrictErrorChecking
*/
public boolean getStrictErrorChecking() {
if (fDelegate != null) {
return fDelegate.getStrictErrorChecking();
} else {
return DOMOps.getStrictErrorChecking(fDocument);
}
}
/**
* See org.w3c.dom.Document#setStrictErrorChecking
*/
public void setStrictErrorChecking(boolean strictErrorChecking) {
if (fDelegate != null) {
fDelegate.setStrictErrorChecking(strictErrorChecking);
} else {
DOMOps.setStrictErrorChecking(fDocument, strictErrorChecking);
}
}
/**
* See org.w3c.dom.Document#getVersion()
*/
public String getVersion() {
if (fDelegate != null) {
return fDelegate.getVersion();
} else {
return DOMOps.getVersion(fDocument);
}
}
/**
* See org.w3c.dom.Document#setVersion
*/
public void setVersion(String version) {
if (fDelegate != null) {
fDelegate.setVersion(version);
} else {
DOMOps.setVersion(fDocument, version);
}
}
/**
* See org.w3c.dom.Document#adoptNode
*/
public Node adoptNode(Node source) throws DOMException {
if (fDelegate != null) {
return fDelegate.adoptNode(source);
} else {
return DOMOps.adoptNode(fDocument, source);
}
}
/**
* @see org.w3c.dom.Node#getNodeName()
*/
public String getNodeName() {
if (fDelegate != null) {
return fDelegate.getNodeName();
} else {
return fDocument.getNodeName();
}
}
/**
* @see org.w3c.dom.Node#getNodeValue()
*/
public String getNodeValue()
throws DOMException {
if (fDelegate != null) {
return fDelegate.getNodeValue();
} else {
return fDocument.getNodeValue();
}
}
/**
* @see org.w3c.dom.Node#setNodeValue
*/
public void setNodeValue(String nodeValue)
throws DOMException {
if (fDelegate != null) {
fDelegate.setNodeValue(nodeValue);
} else {
fDocument.setNodeValue(nodeValue);
}
}
/**
* @see org.w3c.dom.Node#getNodeType
*/
public short getNodeType() {
if (fDelegate != null) {
return fDelegate.getNodeType();
} else {
return fDocument.getNodeType();
}
}
/**
* @see org.w3c.dom.Node#getParentNode
*/
public Node getParentNode() {
if (fDelegate != null) {
return fDelegate.getParentNode();
} else {
return fDocument.getParentNode();
}
}
/**
* @see org.w3c.dom.Node#getChildNodes
*/
public NodeList getChildNodes() {
if (fDelegate != null) {
return fDelegate.getChildNodes();
} else {
return fDocument.getChildNodes();
}
}
/**
* @see org.w3c.dom.Node#getFirstChild
*/
public Node getFirstChild() {
if (fDelegate != null) {
return fDelegate.getFirstChild();
} else {
return fDocument.getFirstChild();
}
}
/**
* @see org.w3c.dom.Node#getLastChild
*/
public Node getLastChild() {
if (fDelegate != null) {
return fDelegate.getLastChild();
} else {
return fDocument.getLastChild();
}
}
/**
* @see org.w3c.dom.Node#getPreviousSibling
*/
public Node getPreviousSibling() {
if (fDelegate != null) {
return fDelegate.getPreviousSibling();
} else {
return fDocument.getPreviousSibling();
}
}
/**
* @see org.w3c.dom.Node#getNextSibling
*/
public Node getNextSibling() {
if (fDelegate != null) {
return fDelegate.getNextSibling();
} else {
return fDocument.getNextSibling();
}
}
/**
* @see org.w3c.dom.Node#getAttributes
*/
public NamedNodeMap getAttributes() {
if (fDelegate != null) {
return fDelegate.getAttributes();
} else {
return fDocument.getAttributes();
}
}
/**
* @see org.w3c.dom.Node#getOwnerDocument
*/
public Document getOwnerDocument() {
if (fDelegate != null) {
return fDelegate.getOwnerDocument();
} else {
return fDocument.getOwnerDocument();
}
}
/**
* @see org.w3c.dom.Node#insertBefore
*/
public Node insertBefore(Node newChild,
Node refChild)
throws DOMException {
if (fDelegate != null) {
return fDelegate.insertBefore(newChild, refChild);
} else {
return fDocument.insertBefore(newChild, refChild);
}
}
/**
* @see org.w3c.dom.Node#replaceChild
*/
public Node replaceChild(Node newChild,
Node oldChild)
throws DOMException {
if (fDelegate != null) {
return fDelegate.replaceChild(newChild, oldChild);
} else {
return fDocument.replaceChild(newChild, oldChild);
}
}
/**
* @see org.w3c.dom.Node#removeChild
*/
public Node removeChild(Node oldChild)
throws DOMException {
if (fDelegate != null) {
return fDelegate.removeChild(oldChild);
} else {
return fDocument.removeChild(oldChild);
}
}
/**
* @see org.w3c.dom.Node#appendChild
*/
public Node appendChild(Node newChild)
throws DOMException {
if (fDelegate != null) {
return fDelegate.appendChild(newChild);
} else {
return fDocument.appendChild(newChild);
}
}
/**
* @see org.w3c.dom.Node#normalize
*/
public void normalize() {
if (fDelegate != null) {
fDelegate.normalize();
} else {
fDocument.normalize();
}
}
/**
* @see org.w3c.dom.Node#isSupported(String, String)
*/
public boolean isSupported(String feature,
String version) {
if (fDelegate != null) {
return fDelegate.isSupported(feature, version);
} else {
return fDocument.isSupported(feature, version);
}
}
/**
* @see org.w3c.dom.Node#getNamespaceURI
*/
public String getNamespaceURI() {
if (fDelegate != null) {
return fDelegate.getNamespaceURI();
} else {
return fDocument.getNamespaceURI();
}
}
/**
* @see org.w3c.dom.Node#getPrefix
*/
public String getPrefix() {
if (fDelegate != null) {
return fDelegate.getPrefix();
} else {
return fDocument.getPrefix();
}
}
/**
* @see org.w3c.dom.Node#setPrefix
*/
public void setPrefix(String prefix) {
if (fDelegate != null) {
fDelegate.setPrefix(prefix);
} else {
fDocument.setPrefix(prefix);
}
}
/**
* @see org.w3c.dom.Node#getLocalName
*/
public String getLocalName() {
if (fDelegate != null) {
return fDelegate.getLocalName();
} else {
return fDocument.getLocalName();
}
}
/**
* @see org.w3c.dom.Node#hasChildNodes
*/
public boolean hasChildNodes() {
if (fDelegate != null) {
return fDelegate.hasChildNodes();
} else {
return fDocument.hasChildNodes();
}
}
/**
* @see org.w3c.dom.Node#hasAttributes
*/
public boolean hasAttributes() {
if (fDelegate != null) {
return fDelegate.hasAttributes();
} else {
return fDocument.hasAttributes();
}
}
/**
* @see XMLObject#toDocument
*/
public String toDocument() {
// Create formatter if needed (no synchronization necessary)
if (fFormatter == null) {
fFormatter = new DOMFormatter(DOMFormatter.getDefaultOutputOptions(getDocument()));
}
if (fDelegate != null) {
return fFormatter.toString(fDelegate);
} else {
return fFormatter.toString(this);
}
}
/**
* Generated function to synchronize the fields used by the access methods.
* This syncronizes just the node and is not recursive.
*/
abstract protected void syncWithDocument(Node node);
/**
* Recursively synchronize access methods.
*/
private void syncAccessMethods(Node node) {
syncWithDocument(node);
for (Node child = node.getFirstChild(); child != null;
child = child.getNextSibling()) {
syncAccessMethods(child);
}
}
/**
* @see XMLObject#syncAccessMethods
*/
public void syncAccessMethods() {
if (fDelegate != null) {
fDelegate.syncAccessMethods();
} else {
syncAccessMethods(fDocument);
}
}
/**
* Old method to initialize the fields used by the generated access
* methods from the current state of the document. This method was
* poorly named and is deprecated.
*
* @deprecated Use syncAccessMethods()
instead.
* @see #syncAccessMethods
*/
public void initFields() {
syncAccessMethods();
}
/**
* @see DocumentInfo#isURLAttribute
*/
public boolean isURLAttribute(Element element,
String attrName) {
return getDomFactory().isURLAttribute(element, attrName);
}
/**
* Used internally to implement a setTextXXX() method. Adds check for
* for null value and helps to minimizes the amount of generated code.
*/
protected final void doSetText(Element element,
String text) {
if (text == null) {
throw new IllegalArgumentException("attempt to set a DOM text node value to null");
}
//jrk_20040703 - org.enhydra.xml.xmlc.compiler.ElementInfo now generates
// setText*() methods for ID's without child nodes. This means
// one can create and expect the setTextFoo(String)
// to be generated. As such, we need to create the text node if it doesn't
// exist before setting data on it.
//XMLCUtil.getFirstText(element).setData(text);
Text textNode = XMLCUtil.findFirstText(element);
if (textNode == null) {
if (fDelegate != null) {
textNode = fDelegate.createTextNode("");
} else {
textNode = fDocument.createTextNode("");
}
element.appendChild(textNode);
}
textNode.setData(text);
}
//DOM3 methods. These are not implemented by Xerces1 which ships with XMLC as of 20050123. Make a note here when they are...
/* (non-Javadoc)
* @see org.w3c.dom.Document#getDocumentURI()
*/
public String getDocumentURI() {
if (fDelegate != null) {
return fDelegate.getDocumentURI();
} else {
return fDocument.getDocumentURI();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#getDomConfig()
*/
public DOMConfiguration getDomConfig() {
if (fDelegate != null) {
return fDelegate.getDomConfig();
} else {
return fDocument.getDomConfig();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#getInputEncoding()
*/
public String getInputEncoding() {
if (fDelegate != null) {
return fDelegate.getInputEncoding();
} else {
return fDocument.getInputEncoding();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#getXmlEncoding()
*/
public String getXmlEncoding() {
if (fDelegate != null) {
return fDelegate.getXmlEncoding();
} else {
return fDocument.getXmlEncoding();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#getXmlStandalone()
*/
public boolean getXmlStandalone() {
if (fDelegate != null) {
return fDelegate.getXmlStandalone();
} else {
return fDocument.getXmlStandalone();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#getXmlVersion()
*/
public String getXmlVersion() {
if (fDelegate != null) {
return fDelegate.getXmlVersion();
} else {
return fDocument.getXmlVersion();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#normalizeDocument()
*/
public void normalizeDocument() {
if (fDelegate != null) {
fDelegate.normalizeDocument();
} else {
fDocument.normalizeDocument();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#renameNode(org.w3c.dom.Node, java.lang.String, java.lang.String)
*/
public Node renameNode(Node arg0, String arg1, String arg2)
throws DOMException {
if (fDelegate != null) {
return fDelegate.renameNode(arg0, arg1, arg2);
} else {
return fDocument.renameNode(arg0, arg1, arg2);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#setDocumentURI(java.lang.String)
*/
public void setDocumentURI(String arg0) {
if (fDelegate != null) {
fDelegate.setDocumentURI(arg0);
} else {
fDocument.setDocumentURI(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#setXmlStandalone(boolean)
*/
public void setXmlStandalone(boolean arg0) throws DOMException {
if (fDelegate != null) {
fDelegate.setXmlStandalone(arg0);
} else {
fDocument.setXmlStandalone(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Document#setXmlVersion(java.lang.String)
*/
public void setXmlVersion(String arg0) throws DOMException {
if (fDelegate != null) {
fDelegate.setXmlVersion(arg0);
} else {
fDocument.setXmlVersion(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#compareDocumentPosition(org.w3c.dom.Node)
*/
public short compareDocumentPosition(Node arg0) throws DOMException {
if (fDelegate != null) {
return fDelegate.compareDocumentPosition(arg0);
} else {
return fDocument.compareDocumentPosition(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#getBaseURI()
*/
public String getBaseURI() {
if (fDelegate != null) {
return fDelegate.getBaseURI();
} else {
return fDocument.getBaseURI();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#getFeature(java.lang.String, java.lang.String)
*/
public Object getFeature(String arg0, String arg1) {
if (fDelegate != null) {
return fDelegate.getFeature(arg0, arg1);
} else {
return fDocument.getFeature(arg0, arg1);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#getTextContent()
*/
public String getTextContent() throws DOMException {
if (fDelegate != null) {
return fDelegate.getTextContent();
} else {
return fDocument.getTextContent();
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#getUserData(java.lang.String)
*/
public Object getUserData(String arg0) {
if (fDelegate != null) {
return fDelegate.getUserData(arg0);
} else {
return fDocument.getUserData(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#isDefaultNamespace(java.lang.String)
*/
public boolean isDefaultNamespace(String arg0) {
if (fDelegate != null) {
return fDelegate.isDefaultNamespace(arg0);
} else {
return fDocument.isDefaultNamespace(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#isEqualNode(org.w3c.dom.Node)
*/
public boolean isEqualNode(Node arg0) {
if (fDelegate != null) {
return fDelegate.isEqualNode(arg0);
} else {
return fDocument.isEqualNode(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#isSameNode(org.w3c.dom.Node)
*/
public boolean isSameNode(Node arg0) {
if (fDelegate != null) {
return fDelegate.isSameNode(arg0);
} else {
return fDocument.isSameNode(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#lookupNamespaceURI(java.lang.String)
*/
public String lookupNamespaceURI(String arg0) {
if (fDelegate != null) {
return fDelegate.lookupNamespaceURI(arg0);
} else {
return fDocument.lookupNamespaceURI(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#lookupPrefix(java.lang.String)
*/
public String lookupPrefix(String arg0) {
if (fDelegate != null) {
return fDelegate.lookupPrefix(arg0);
} else {
return fDocument.lookupPrefix(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#setTextContent(java.lang.String)
*/
public void setTextContent(String arg0) throws DOMException {
if (fDelegate != null) {
fDelegate.setTextContent(arg0);
} else {
fDocument.setTextContent(arg0);
}
}
/* (non-Javadoc)
* @see org.w3c.dom.Node#setUserData(java.lang.String, java.lang.Object, org.w3c.dom.UserDataHandler)
*/
public Object setUserData(String arg0, Object arg1, UserDataHandler arg2) {
if (fDelegate != null) {
return fDelegate.setUserData(arg0, arg1, arg2);
} else {
return fDocument.setUserData(arg0, arg1, arg2);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy